By: CS2103AUG2017 T16-B2
Since: Sep 2017
Licence: MIT
- 1. Introduction
- 2. Setting up
- 3. Design
- 4. Implementation
- 4.1. Command Alias mechanism
- 4.2. Optional Inputs/Fields mechanism
- 4.3. Remark mechanism
- 4.4. FindTags mechanism
- 4.5. Undo/Redo mechanism
- 4.6. Sort mechanism
- 4.7. Add Schedule mechanism
- 4.8. View Schedule mechanism
- 4.9. Home Page mechanism
- 4.10. GraphPanel Mechanism
- 4.11. Tabs mechanism
- 4.12. Statistics Panel mechanism
- 4.13. Unique Color Tag mechanism
- 4.14. Logging
- 4.15. Configuration
- 5. Unused/Removed Features
- 6. Documentation
- 7. Testing
- 8. Dev Ops
- Appendix A: Suggested Programming Tasks to Get Started
- Appendix B: User Stories
- Appendix C: Use Cases
- Appendix D: Non Functional Requirements
- Appendix E: Glossary
- Appendix F: Product Survey
1. Introduction
1.1. Software Overview
Welcome to cherBook, the Command Line application designed to help teachers monitor students' academic performance easily. With our tagging system and data analysis tools, teachers can view statistics of any specific groups of students. This helps teachers by breaking down students' grades and identifying students who need extra attention. Finally, if ever the need arises, student’s parent contact details can be found in just one command, making cherBook the fastest way to contact students' parents.
1.2. About this guide
This developer guide provides future contributors and maintainers of cherBook with the necessary information to understand the cherBook source code and guidelines for contributing to the repository. Such information include cherBook’s software architecture, major components, APIs, Documentation and contributing guidelines.
2. Setting up
Follow the instructions below to set up and configure cherBook project files on your computer.
2.1. Prerequisites
Before you can start configuring, you need to have the following software installed:
-
JDK
1.8.0_60
or laterHaving any Java 8 version is not enough.
This app will not work with earlier versions of Java 8. -
IntelliJ IDE
IntelliJ by default has Gradle and JavaFx plugins installed.
Do not disable them. If you have disabled them, go toFile
>Settings
>Plugins
to re-enable them.
2.2. Setting up the project in your computer
-
Fork this repo, and clone the fork to your computer
-
Open IntelliJ (if you are not in the welcome screen, click
File
>Close Project
to close the existing project dialog first) -
Set up the correct JDK version for Gradle
-
Click
Configure
>Project Defaults
>Project Structure
-
Click
New…
and find the directory of the JDK
-
-
Click
Import Project
-
Locate the
build.gradle
file and select it. ClickOK
-
Click
Open as Project
-
Click
OK
to accept the default settings -
Open a console and run the command
gradlew processResources
(Mac/Linux:./gradlew processResources
). It should finish with theBUILD SUCCESSFUL
message.
This will generate all resources required by the application and tests.
2.3. Verifying the setup
-
Run the
seedu.address.MainApp
and try a few commands -
Run the tests to ensure they all pass.
2.4. Configurations to do before writing code
2.4.1. Configuring the coding style
This project follows oss-generic coding standards. IntelliJ’s default style is mostly compliant with ours but it uses a different import order from ours. To rectify,
-
Go to
File
>Settings…
(Windows/Linux), orIntelliJ IDEA
>Preferences…
(macOS) -
Select
Editor
>Code Style
>Java
-
Click on the
Imports
tab to set the order-
For
Class count to use import with '*'
andNames count to use static import with '*'
: Set to999
to prevent IntelliJ from contracting the import statements -
For
Import Layout
: The order isimport static all other imports
,import java.*
,import javax.*
,import org.*
,import com.*
,import all other imports
. Add a<blank line>
between eachimport
-
Optionally, you can follow the UsingCheckstyle.adoc document to configure Intellij to check style-compliance as you write code.
2.4.2. Updating documentation to match your fork
After forking the repo, links in the documentation will still point to the CS2103AUG2017-T16-B2/main
repo. If you plan to develop this as a separate product (i.e. instead of contributing to the CS2103AUG2017-T16-B2/main
) , you should replace the URL in the variable repoURL
in DeveloperGuide.adoc
and UserGuide.adoc
with the URL of your fork.
2.4.3. Setting up CI
Set up Travis to perform Continuous Integration (CI) for your fork. See UsingTravis.adoc to learn how to set it up.
Optionally, you can set up AppVeyor as a second CI (see UsingAppVeyor.adoc).
Having both Travis and AppVeyor ensures your App works on both Unix-based platforms and Windows-based platforms (Travis is Unix-based and AppVeyor is Windows-based) |
2.4.4. Getting started with coding
When you are ready to start coding,
-
Get some sense of the overall design by reading the Architecture section.
-
Take a look at the section Suggested Programming Tasks to Get Started.
3. Design
This section explains to you the design of the app, its architecture and components. It also contains the details, reasons and mechanisms behind major system components and the interactions between these components.
3.1. Architecture
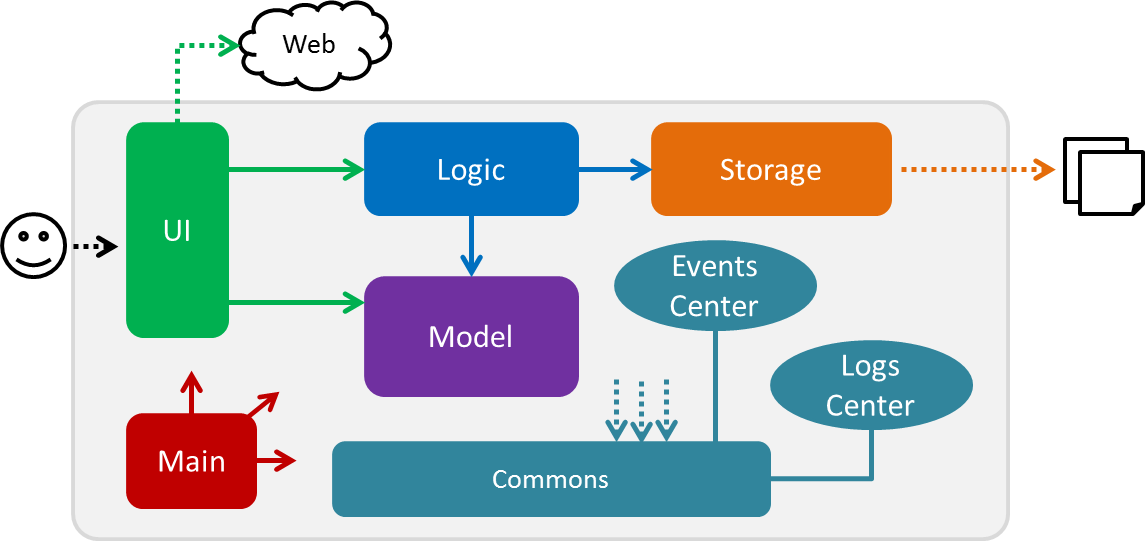
Figure 3.1.1 : Architecture Diagram
The Architecture Diagram given above explains the high-level design of the App. Given below is a quick overview of each component.
The .pptx files used to create diagrams in this document can be found in the diagrams folder. To update a diagram, modify the diagram in the pptx file, select the objects of the diagram, and choose Save as picture .
|
Main
has only one class called MainApp
. It is responsible for,
-
At app launch: Initializes the components in the correct sequence, and connects them up with each other.
-
At shut down: Shuts down the components and invokes cleanup method where necessary.
Commons
represents a collection of classes used by multiple other components. Two of those classes play important roles at the architecture level.
-
EventsCenter
: This class (written using Google’s Event Bus library) is used by components to communicate with other components using events (i.e. a form of Event Driven design) -
LogsCenter
: Used by many classes to write log messages to the App’s log file.
The rest of the App consists of four components.
Each of the four components
-
Defines its API in an
interface
with the same name as the Component. -
Exposes its functionality using a
{Component Name}Manager
class.
For example, the Logic
component (see the class diagram given below) defines it’s API in the Logic.java
interface and exposes its functionality using the LogicManager.java
class.
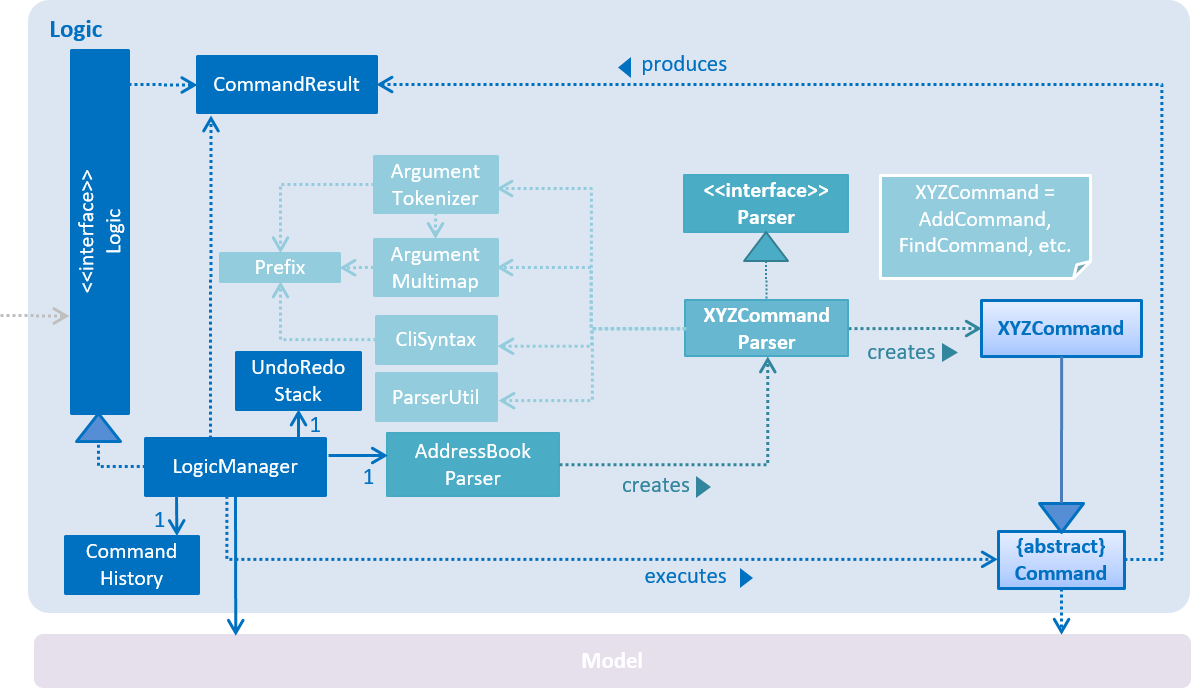
Figure 3.1.2 : Class Diagram of the Logic Component
Events-Driven nature of the design
The Sequence Diagram below shows how the components interact for the scenario where the user issues the command delete 1
.
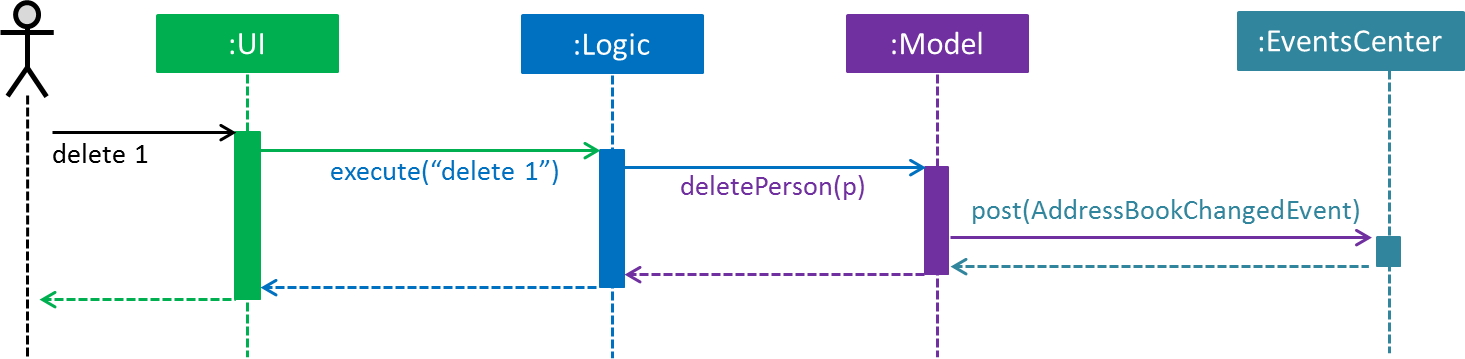
Figure 3.1.3a : Component interactions for delete 1
command (part 1)
Note how the Model simply raises a AddressBookChangedEvent when the Address Book data are changed, instead of asking the Storage to save the updates to the hard disk.
|
The diagram below shows how the EventsCenter
reacts to that event, which eventually results in the updates being saved to the hard disk and the status bar of the UI being updated to reflect the 'Last Updated' time.
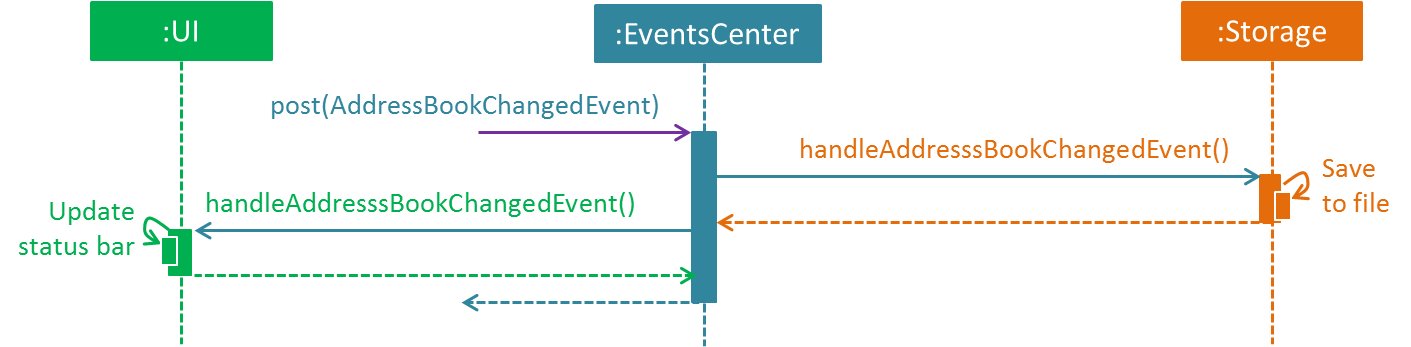
Figure 3.1.3b : Component interactions for delete 1
command (part 2)
Note how the event is propagated through the EventsCenter to the Storage and UI without Model having to be coupled to either of them. This is an example of how this Event Driven approach helps us reduce direct coupling between components.
|
The sections below give more details of each component.
3.2. UI component
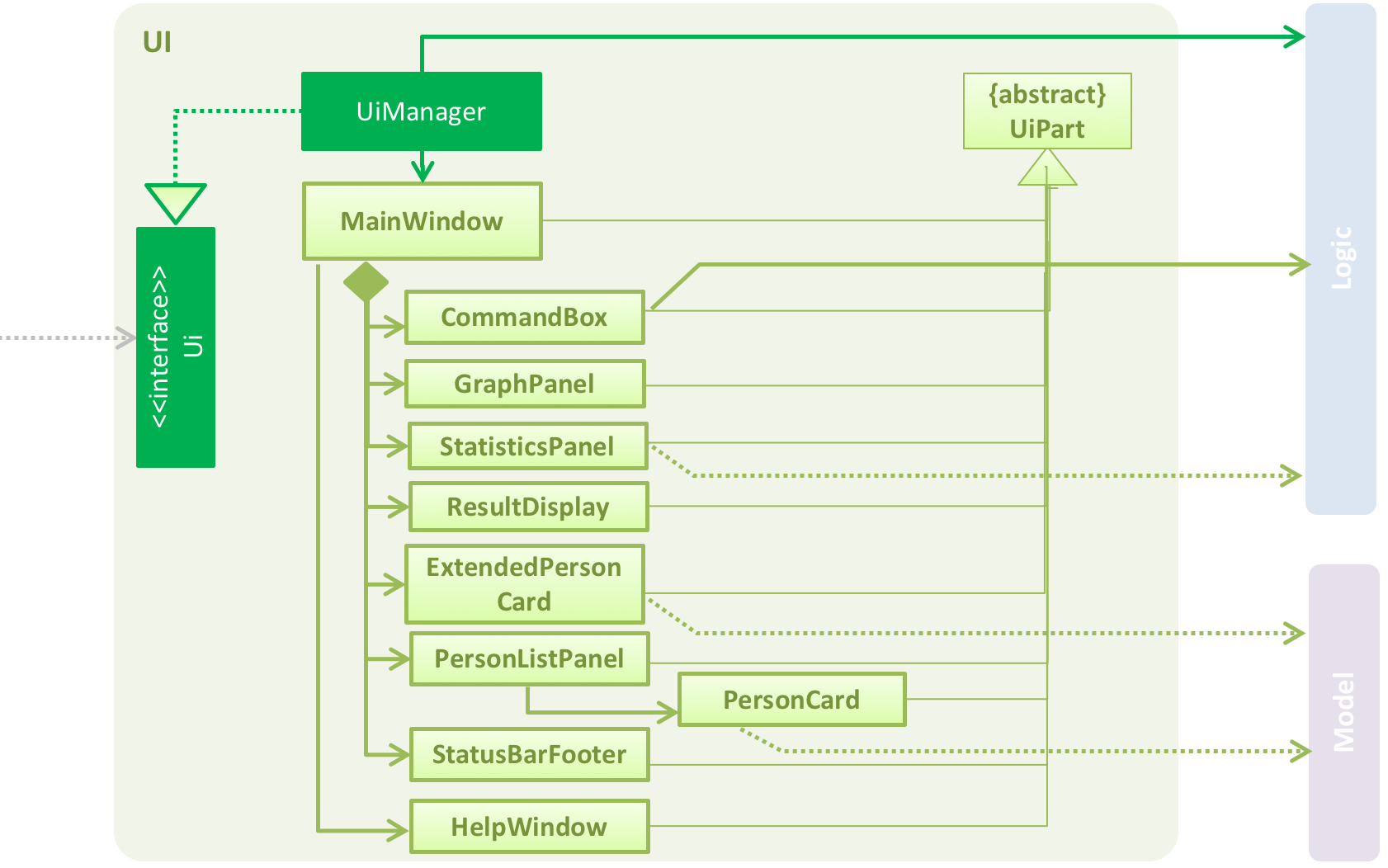
Figure 3.2.1 : Structure of the UI Component
API : Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, PersonListPanel
, StatusBarFooter
, ExtendedPersonCard
, StatisticsPanel
, GraphPanel
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
-
Executes user commands using the
Logic
component. -
Binds itself to some data in the
Model
so that the UI can auto-update when data in theModel
change. -
Responds to events raised from various parts of the App and updates the UI accordingly.
3.3. Logic component
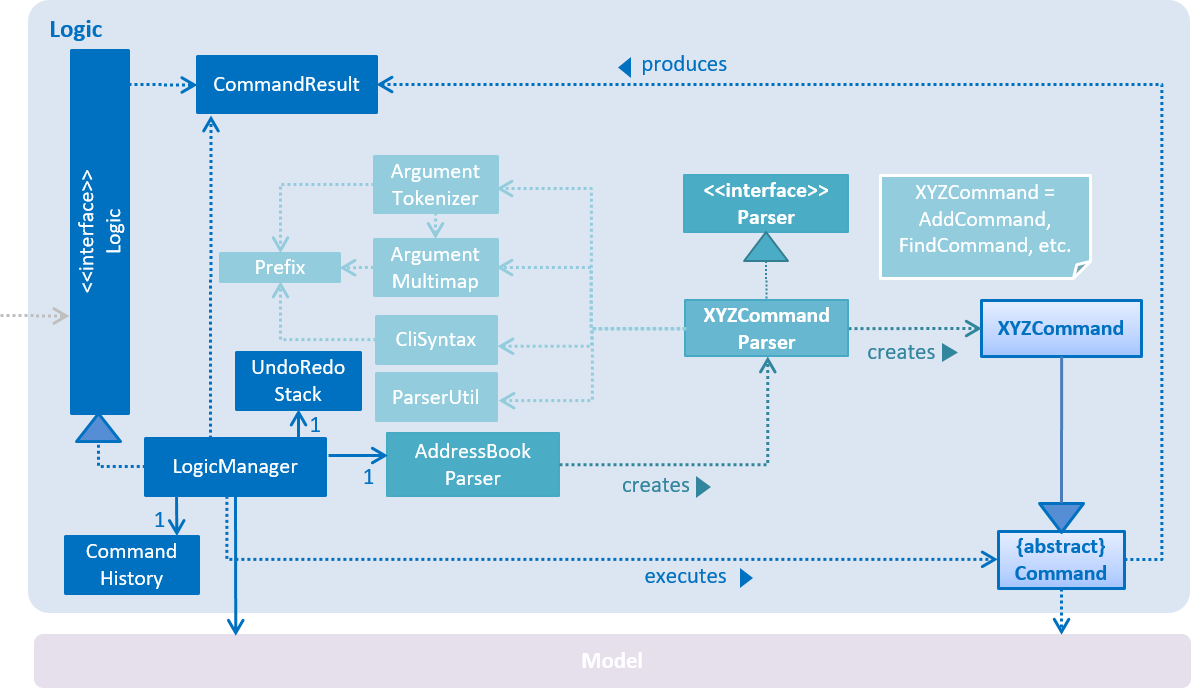
Figure 3.3.1 : Structure of the Logic Component
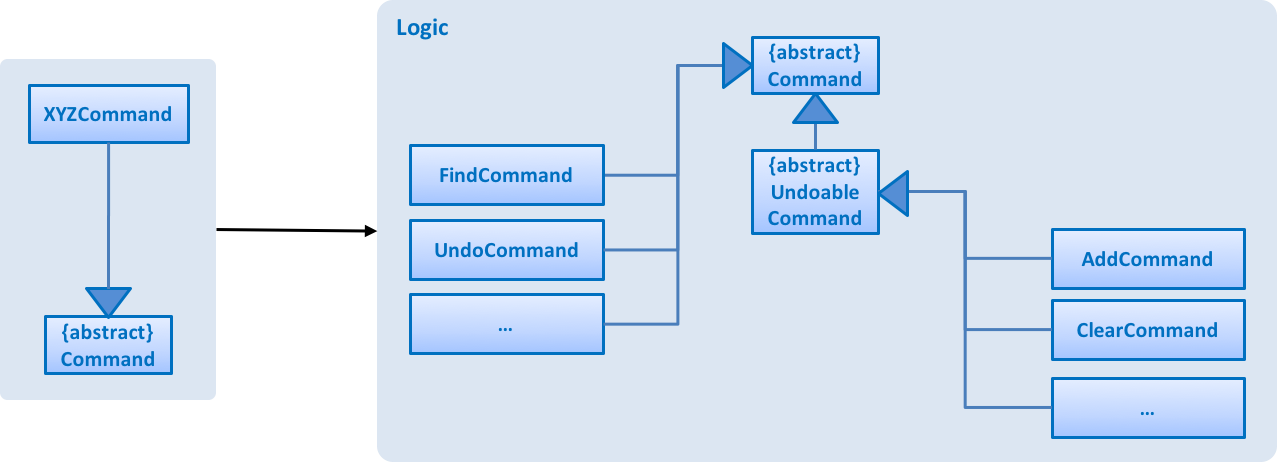
Figure 3.3.2 : Structure of Commands in the Logic Component. This diagram shows finer details concerning XYZCommand
and Command
in Figure 2.3.1
API :
Logic.java
-
Logic
uses theAddressBookParser
class to parse the user command. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. adding a person) and/or raise events. -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("delete 1")
API call.
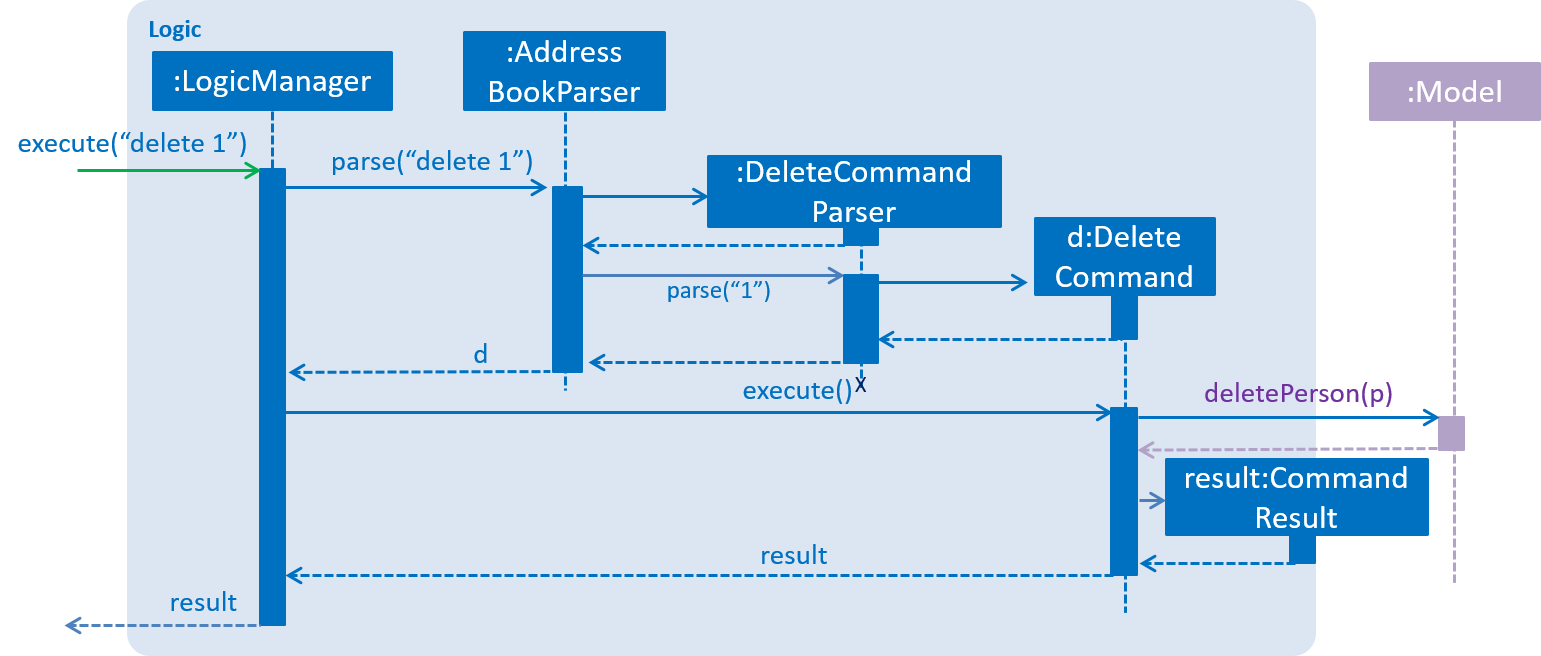
Figure 3.3.3 : Interactions Inside the Logic Component for the delete 1
Command
3.4. Model component
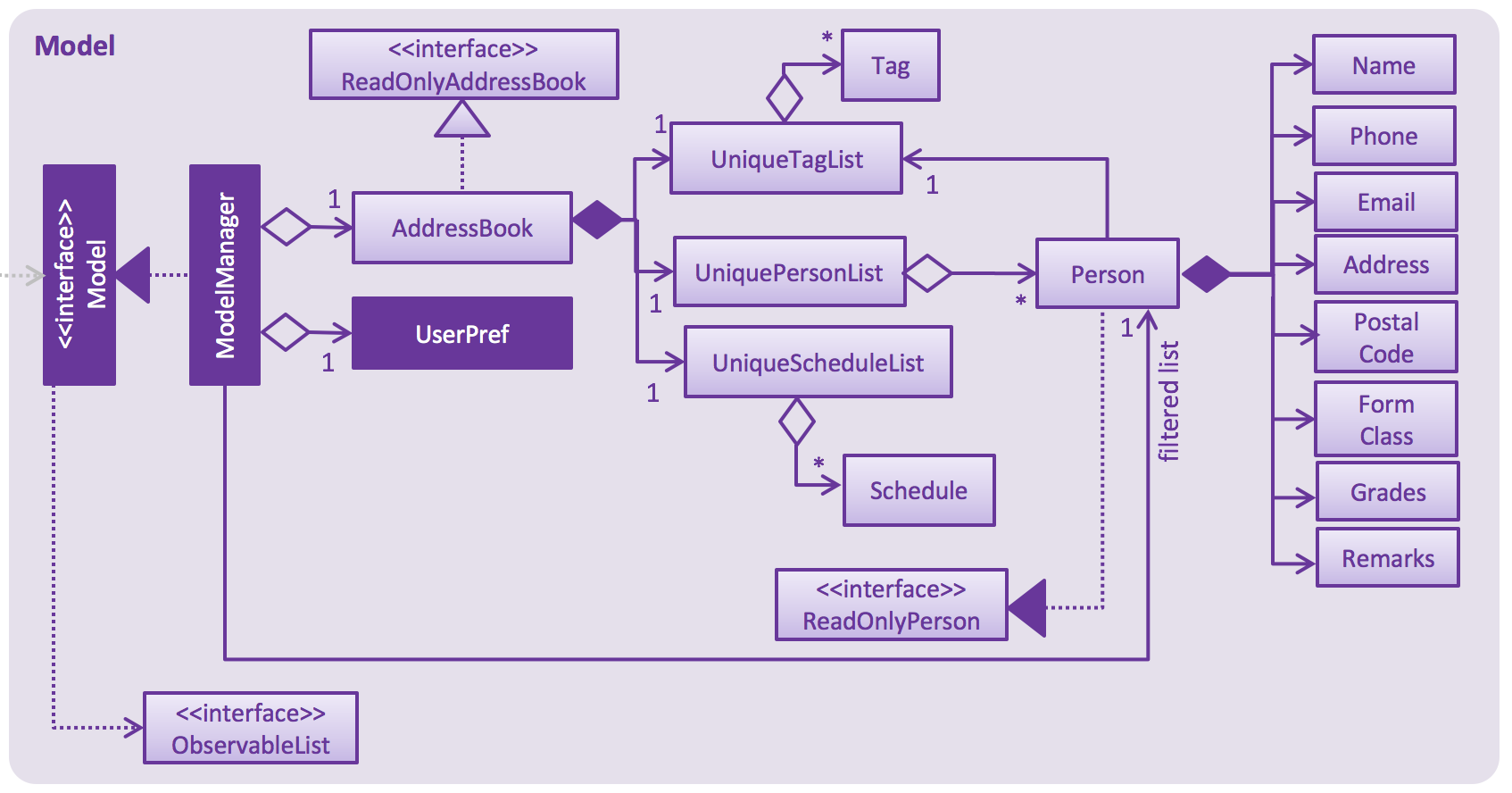
Figure 3.4.1 : Structure of the Model Component
API : Model.java
The Model
,
-
stores a
UserPref
object that represents the user’s preferences. -
stores the Address Book data.
-
exposes an unmodifiable
ObservableList<ReadOnlyPerson>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. -
does not depend on any of the other three components.
3.5. Storage component
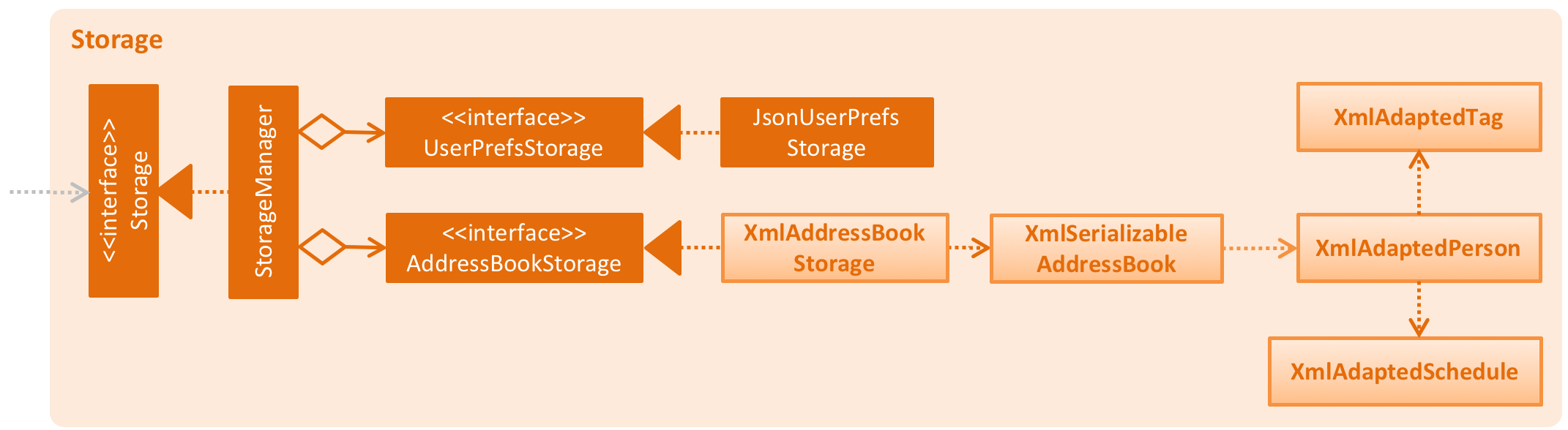
Figure 3.5.1 : Structure of the Storage Component
API : Storage.java
The Storage
component,
-
can save
UserPref
objects in json format and read it back. -
can save the Address Book data in xml format and read it back.
3.6. Common classes
Classes used by multiple components are in the seedu.addressbook.commons
package.
4. Implementation
This section explains to you in detail how certain features of the cherBook are implemented. The relevant diagrams and code examples will be used to aid the understanding of the features' implementation.
4.1. Command Alias mechanism
The Command Alias mechanism is facilitated by the COMMAND_ALIAS
in each Command
class and the parseCommand()
method in AddressBookParser
.
The AddressBookParser
is responsible for analyzing command words while each command’s COMMAND_ALIAS
stores the command alias to be accepted by the parser.
The aliased added to each command class is implemented like this e.g. FindCommand
:
public class FindCommand extends Command {
public static final String COMMAND_WORD = "find";
public static final String COMMAND_ALIAS = "f";
//...... other logic ......
}
Just like how we store each individual command word constant COMMAND_WORD
inside each Command
class e.g. FindCommand.COMMAND_WORD
, DeleteCommand.COMMAND_WORD
, a new constant was added for each command alias e.g. FindCommand.COMMAND_ALIAS
.
The parseCommand()
in AddressBookParser
is implemented like this:
public Command parseCommand(String userInput) throws ParseException {
final Matcher matcher = BASIC_COMMAND_FORMAT.matcher(userInput.trim());
if (!matcher.matches()) {
throw new ParseException(String.format(MESSAGE_INVALID_COMMAND_FORMAT, HelpCommand.MESSAGE_USAGE));
}
final String commandWord = matcher.group("commandWord");
final String arguments = matcher.group("arguments");
switch (commandWord) {
case AddCommand.COMMAND_WORD://Fallthrough
case AddCommand.COMMAND_ALIAS:
return new AddCommandParser().parse(arguments);
// ...... more conditions ......
default:
throw new ParseException(MESSAGE_UNKNOWN_COMMAND);
}
}
When a command alias is detected in the switch statement, it will result in the return statement below it, causing it to behave similarly to its corresponding command word. When a command word is detected, it will fall through and executes the command as per normal.
4.1.1. Design Considerations
Aspect: Where to add the command Aliases
Alternative 1 (current choice): Add the aliases in each Command
Class
Pros: More cohesive command classes.
Cons: None.
Alternative 2: Add the aliases in the AddressBookParser.parseCommand(String) method.
Pros: Easier to read code in the AddressBookParser .
Cons: Use of magic numbers/strings means that the code is harder to trace and debug.
4.2. Optional Inputs/Fields mechanism
The optional inputs/fields
mechanism is facilitated by making changes to AddCommandParser
class in the Logic
component.
This feature enables several input/fields of the student to be optional.
Student’s phone number, email, address and postal code are optional inputs/fields. |
The following sequence diagram shows you how the optional inputs/fields
operation works:
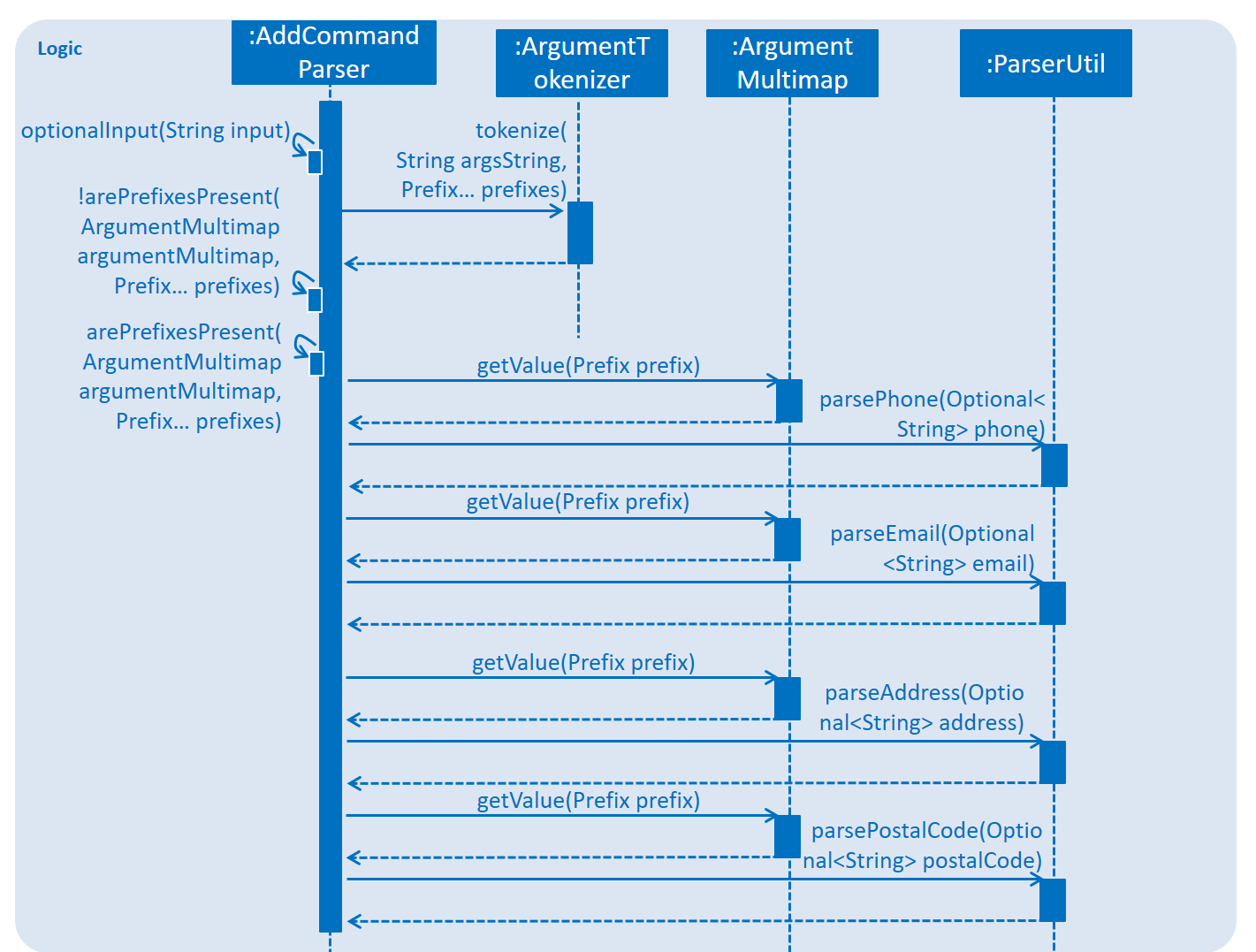
Figure 4.2.1 : Sequence Diagram of Optional Inputs/Fields Operation
The optionalInput method will append messages indicating that those optional fields are not recorded if the user chooses not to include those fields. The method can be seen in the code section below.
public static String optionalInput(String input) { if (!input.contains("a/")) { input = input + " a/ (Address not recorded)"; } if (!input.contains("e/")) { input = input + " e/ (Email not recorded)"; } if (!input.contains("c/")) { input = input + " c/ (Postal code not recorded)"; } if (!input.contains(" p/")) { input = input + " p/ (Student phone not recorded)"; } return input; }
The output of the method is illustrated in the following picture.
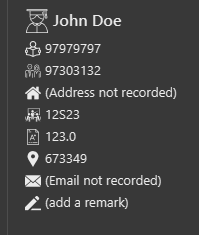
Figure 4.2.2 : Messages shown when the user does not enter the optional fields
Prefixes of the optional fields - PREFIX_PHONE
, PREFIX_EMAIL
, PREFIX_ADDRESS
and PREFIX_POSTALCODE
are removed from
the code segment below. The ensures that the ParseException
will not be thrown if the user chooses not to include the
optional fields.
if (!arePrefixesPresent(argMultimap, PREFIX_NAME, PREFIX_PHONE, PREFIX_PARENTPHONE, PREFIX_FORMCLASS, PREFIX_GRADES)) { throw new ParseException(String.format(MESSAGE_INVALID_COMMAND_FORMAT, AddCommand.MESSAGE_USAGE)); }
4.2.1. Design Considerations
Aspect: Implementation of optional fields/inputs.
Alternative 1 (current choice): This feature manipulates the input to include the missing optional input prefix, along with a message
stating that the field is not recorded.
Pros: Simpler to understand and tweak this feature as it only manipulates the parsed input of the user.
Cons: Unable to implement UI features that requires the attribute to have a optional property.
Alternative 2: This feature implements a optional characteristic in optional attributes.
Pros: Optionality aspect of the attributes can be used by other features of cherBook.
Cons: Harder to understand the mechanism and more work is required implement changes to this feature.
4.3. Remark mechanism
The remark
mechanism is facilitated by making changes to the Remark
class in the Model
component. It enables the user to overwrite a single remark
of the student.
To avoid confusion and an overly complicated UI
, only one remark
per person is stored.
The following sequence diagram shows how the remark
operation works:
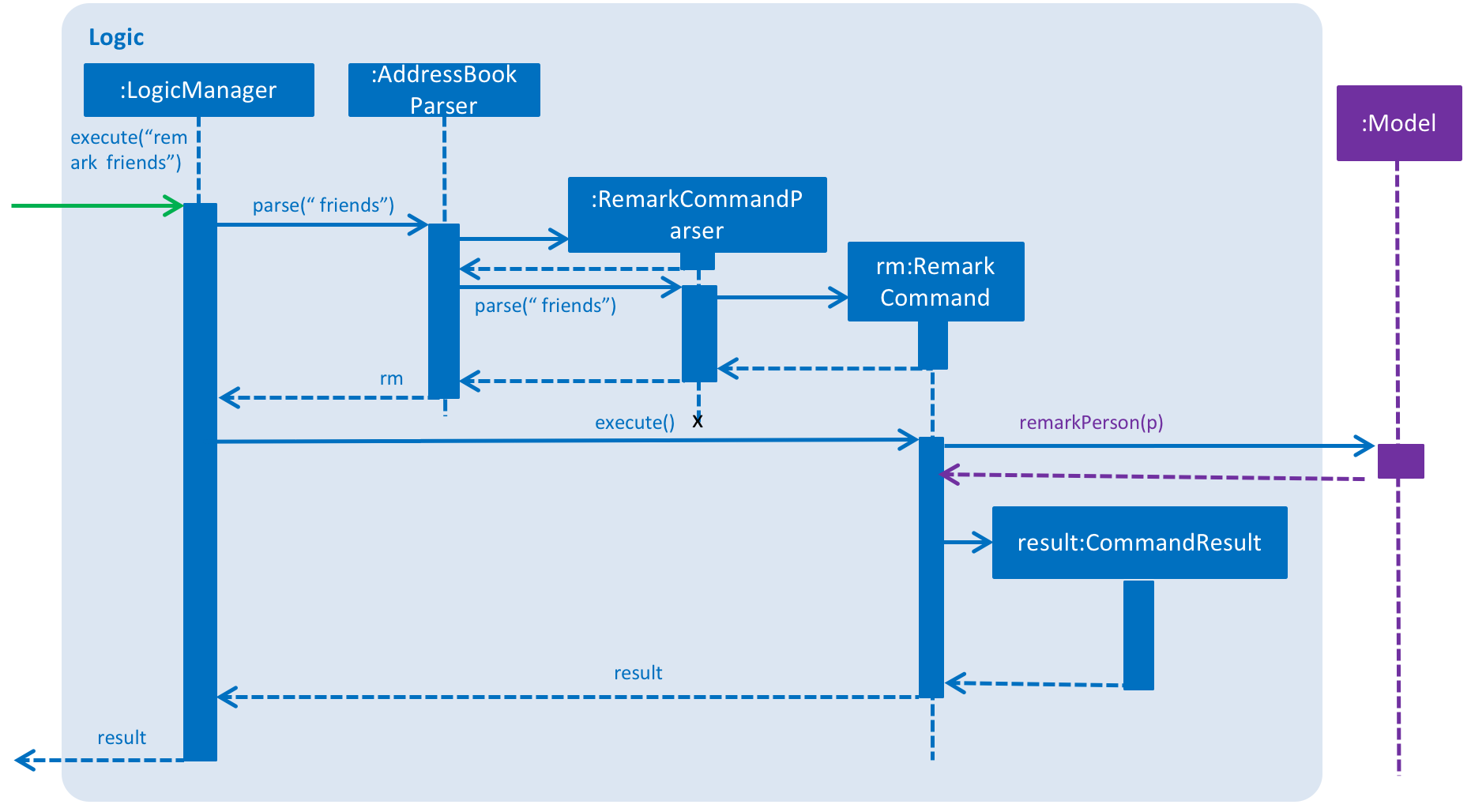
Figure 4.3.1 : Sequence Diagram of Remark
Operation
Remark
only deals with UndoableCommands
. Commands that cannot be undone will inherit from Command
instead. The following diagram shows the inheritance diagram for commands:
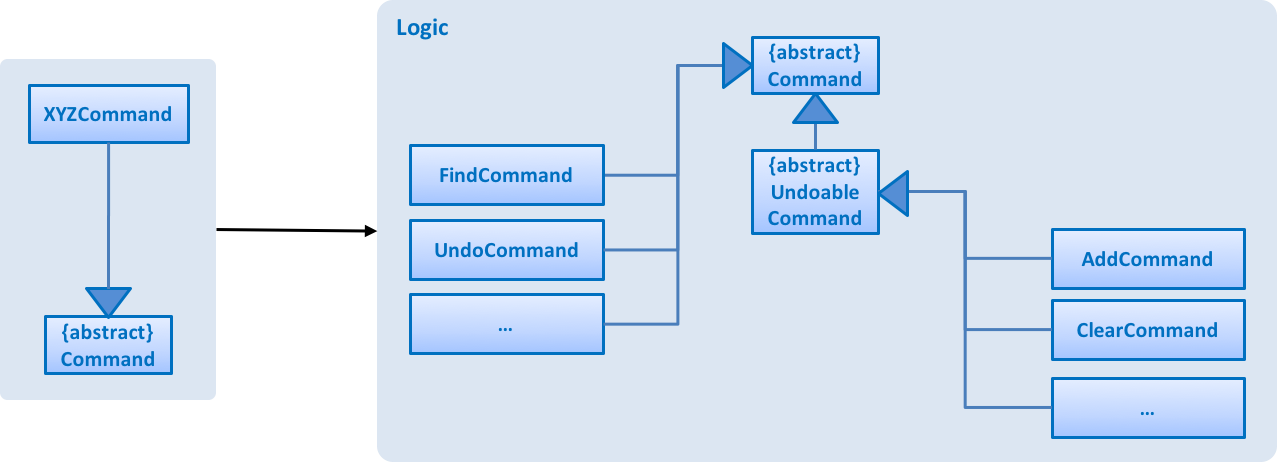
Figure 4.3.2 : Inheritance Diagram for Commands
As you can see from the diagram, UndoableCommand
adds an extra layer between the abstract Command
class and concrete commands that can be undone, such as the DeleteCommand
. Note that extra tasks need to be done when executing a command in an undoable way, such as saving the state of the address book before execution. UndoableCommand
contains the high-level algorithm for those extra tasks while the child classes implements the details of how to execute the specific command. Note that this technique of putting the high-level algorithm in the parent class and lower-level steps of the algorithm in child classes is also known as the template pattern.
Commands that are not undoable are implemented this way:
public class RemarkCommand extends Command {
@Override
public CommandResult execute() {
// ... remark logic ...
}
}
With the extra layer, the commands that are undoable are implemented this way:
public abstract class UndoableCommand extends Command {
@Override
public CommandResult execute() {
// ... undo logic ...
executeUndoableCommand();
}
}
public class DeleteCommand extends UndoableCommand {
@Override
public CommandResult executeUndoableCommand() {
// ... delete logic ...
}
}
When the user adds a new person
to the address book, that person’s remark
field will be denoted by (add a remark)
. Users are then able to add a single remark
.
If the user tries to add remark together with adding a new Person , it will display as invalid as the addCommand does not allow the addition of remarks other then through the use of using the Remark command.
|
When the user attempts to add /edit a remark via the add /edit command, he will be prompted by a message stating
that adding of remarks in add /edit command is not allowed.
|
Only one remark is saved at any one time, if the user does Remark on the same person, it will be overwritten.
|
4.3.1. Design Considerations
Aspect: Implementation of UndoableCommand
Alternative 1 (current choice): Add a new abstract method executeUndoableCommand()
.
Pros: We will not lose any undone/redone functionality as it is now part of the default behaviour. Classes that deal with Command
do not have to know that executeUndoableCommand()
exist.
Cons: Hard for new developers to understand the template pattern.
Alternative 2: Just override execute()
.
Pros: Does not involve the template pattern, easier for new developers to understand.
Cons: Classes that inherit from UndoableCommand
must remember to call super.execute()
, or lose the ability to undo/redo.
Aspect Implementation of Remark
field.
Alternative 1 (current choice): Add a field in person as well as making it a command.
Pros: Able to easily change context of the field without much hassle.
Cons: Harder to debug as it is both a field and a command.
Alternative 2: Making it a field only.
Pros: Easier to keep track and debug.
Cons: Much harder and longer to implement a feature to solely change the remarks.
4.4. FindTags mechanism
The FindTagsCommand
class inheriteds from the Command
class.
It allows users to search students by their tags.
The FindTags
mechanism is facilitated by the TagsContainKeywordsPredicate
, which resides within FindTagsCommand
.
The TagsContainKeywordsPredicate
tests whether each person’s tags contain all keywords input by the user
and thus supports AND search.
The following sequence diagram shows how the FindTags operation works:
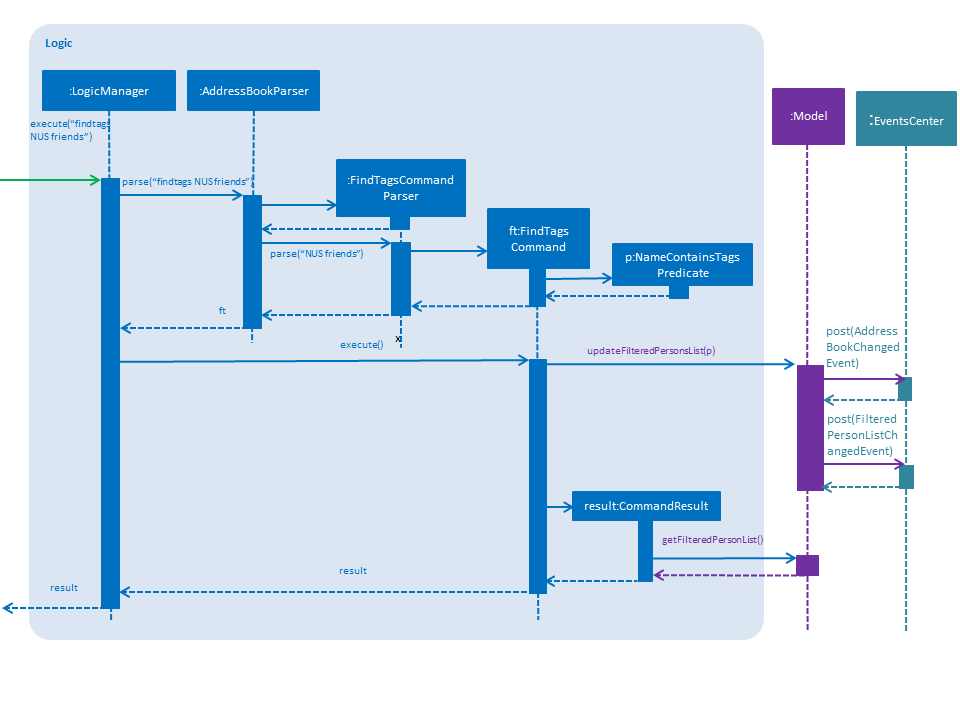
Figure 4.4.1 : Sequence Diagram of FindTags
Operation
As can seen from above, when a FindTags
command is called, E.g. findtags NUS friends
.
An instance of TagsContainKeywordsPredicate
is created.
The newly created TagsContainsKeywordsPredicate
used to implement FindTagsCommand
is shown below:
public class TagsContainsKeywordsPredicate implements Predicate<ReadOnlyPerson> { private final List<String> keywords; @Override public boolean test(ReadOnlyPerson person) { return keywords.stream() .allMatch(keyword -> StringUtil.containsWordIgnoreCase(person.getTagsAsString(), keyword)); } // ... other logic ... }
The test is case insensitive as StringUtil.containsWordIgnoreCase() ignores case.
|
This search operation is an AND search
So only persons with at least both friends and NUS will be displayed. Persons with only friends or only NUS will not be displayed. |
Inside the TagsContainKeywordsPredicate
, the search terms NUS
and friends
are stored inside the keywords
list.
The overidding test()
method evluates whether the person’s tags matches all the search terms in keywords
.
This TagsContainKeywordsPredicate
is then used to test every person in the AddressBook and filter them.
The newly created FindTagsCommand
class is shown below:
public class FindTagsCommand extends Command { private final TagsContainsKeywordsPredicate predicate; @Override public CommandResult execute() { model.updateFilteredPersonList(predicate); return new CommandResult( getMessageForPersonListShownSummary(model.getFilteredPersonList().size())); } // ... other logic ... }
The predicate
is passed through model.updateFilteredPersonList(predicate)
to update the filteredPersonList
.
This causes the filteredPersonList
to only contain students whose tags match all keywords NUS
and friends
and also display them in the PersonListPanel
UI element.
However if there are no persons with all keywords in the tags, the PersonListPanel
will just be blank.
Meanwhile, the ModelManager
posts a AddressBookChanged event
and a FilteredPersonListChangedEvent
to the EventsCenter
.
The AddressBookChangedEvent
indicates that the model
has changed and the causing the FilteredPersonListChangedEvent
updates the StatisticsPanel
of the UI to reflect the new Statistics for the new list of students.
This search feature is implemented using Predicate
because Predicate
help move the business logic to a more central place, helping in unit-testing them separately.
Also, the Predicate
can can be reused, improving code manageability and readability.
4.4.1. Design Considerations
Aspect: Have a separate predicate class to support findtags
Alternative 1 (current choice): Have a TagsContainsKeywordsPredicate
to facilitate search.
Pros: Easy for new Computer Science undergraduates to understand. They are likely to be the new incoming developers of our project.
Cons: Makes the application more complex to understand according to the More Is More Complex (MIMC) principle. For example, a separate TagsContainsKeywordsPredicate
class is needed when the same can be done by inserting it as an inner class in the FindTagsCommand
class.
Alternative 2: Create TagsContainsKeywordsPredicate
as an inner class in FindTagsCommand
class.
Pros: It makes the codebase less complex as there are less classes.
Cons: It adds complexity to the FindTagsCommand
source file and reduces re-usability of the Predicate
outside of the FindTagsCommand
Aspect: Whether to use AND or OR search
Alternative 1 (current choice): AND search
Pros: Allows users to find students with a specific combination of tags much more efficiently.
E.g. findtags band dance
only returns the list of students who have both the band
and dance
tag rather all students with either the band
or dance
tag.
Cons: Does not allow users to calculate statistics for more than one group of students at a time.
E.g. findtags band dance
cannot calculate statistics for the track
and dance
groups combined.
Alternative 2: OR search
Pros: Allows users to calculate statistics for more than one group of students at a time.
E.g. findtags track dance
calculates statistics for the track
and dance
groups combined.
Cons: Or search does not help teachers find students with a specific combination of tags efficiently.
E.g. findtags track dance
does not narrow down the list of students returned to students who have both the track
and dance
tag.
4.5. Undo/Redo mechanism
The undo/redo mechanism is facilitated by an UndoRedoStack
, which resides inside LogicManager
. It supports undoing and redoing of commands that modifies the state of the address book (e.g. add
, edit
). Such commands will inherit from UndoableCommand
.
UndoRedoStack
only deals with UndoableCommands
. Commands that cannot be undone will inherit from Command
instead. The following diagram shows the inheritance diagram for commands:
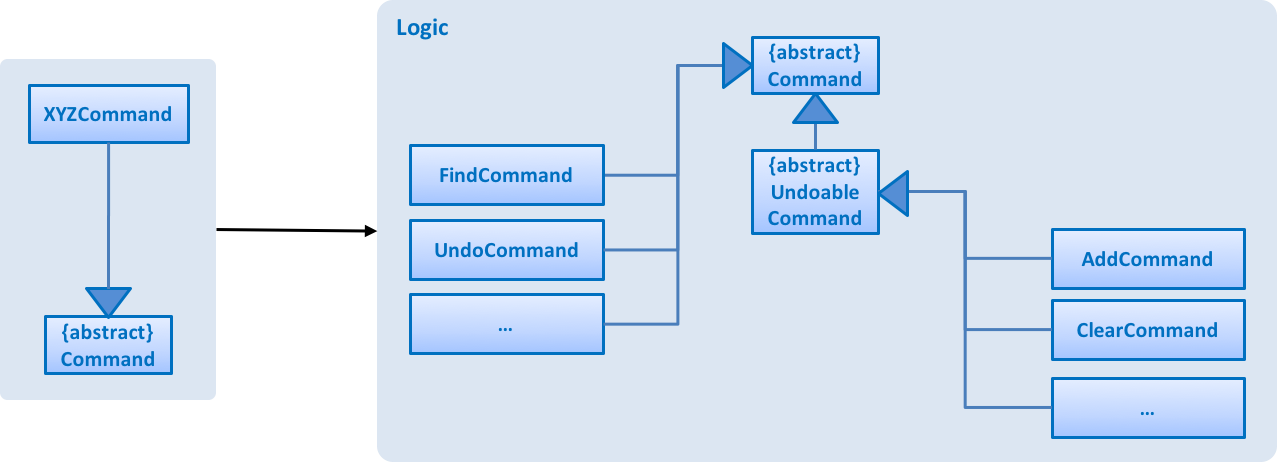
As you can see from the diagram, UndoableCommand
adds an extra layer between the abstract Command
class and concrete commands that can be undone, such as the DeleteCommand
. Note that extra tasks need to be done when executing a command in an undoable way, such as saving the state of the address book before execution. UndoableCommand
contains the high-level algorithm for those extra tasks while the child classes implements the details of how to execute the specific command. Note that this technique of putting the high-level algorithm in the parent class and lower-level steps of the algorithm in child classes is also known as the template pattern.
Commands that are not undoable are implemented this way:
public class ListCommand extends Command {
@Override
public CommandResult execute() {
// ... list logic ...
}
}
With the extra layer, the commands that are undoable are implemented this way:
public abstract class UndoableCommand extends Command {
@Override
public CommandResult execute() {
// ... undo logic ...
executeUndoableCommand();
}
}
public class DeleteCommand extends UndoableCommand {
@Override
public CommandResult executeUndoableCommand() {
// ... delete logic ...
}
}
Suppose that the user has just launched the application. The UndoRedoStack
will be empty at the beginning.
The user executes a new UndoableCommand
, delete 5
, to delete the 5th person in the address book. The current state of the address book is saved before the delete 5
command executes. The delete 5
command will then be pushed onto the undoStack
(the current state is saved together with the command).
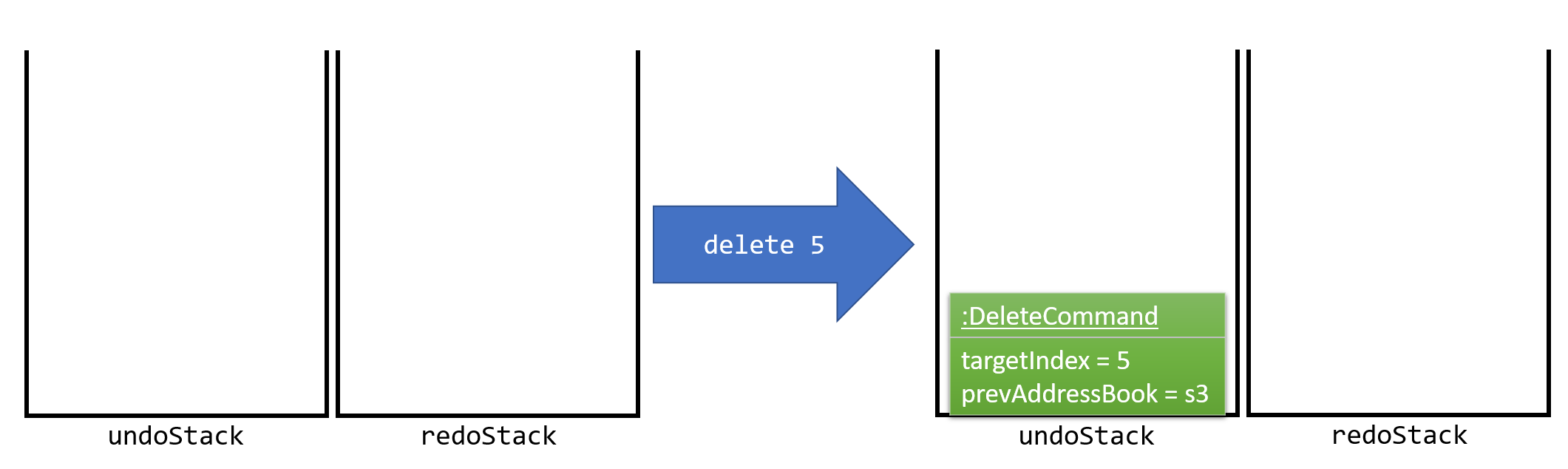
Figure 4.5.1 : Stack Diagram of Initial Stack
As the user continues to use the program, more commands are added into the undoStack
. For example, the user may execute add n/David …
to add a new person.
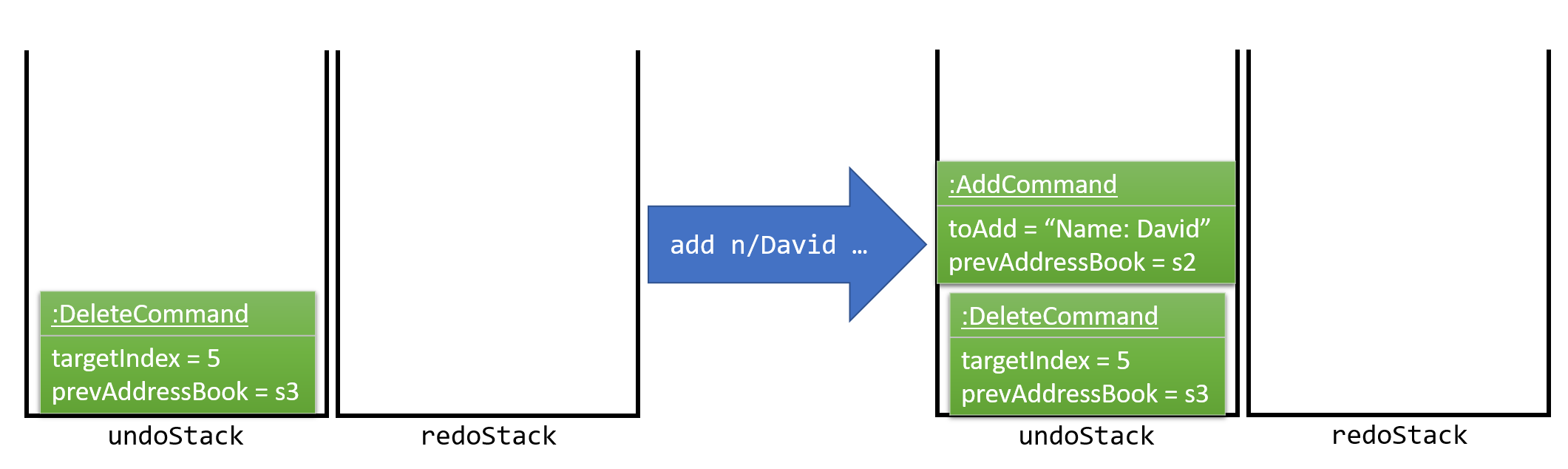
Figure 4.5.2 : Stack Diagram of Commands Added
If a command fails its execution, it will not be pushed to the UndoRedoStack at all.
|
The user now decides that adding the person was a mistake, and decides to undo that action using undo
.
We will pop the most recent command out of the undoStack
and push it back to the redoStack
. We will restore the address book to the state before the add
command executed.
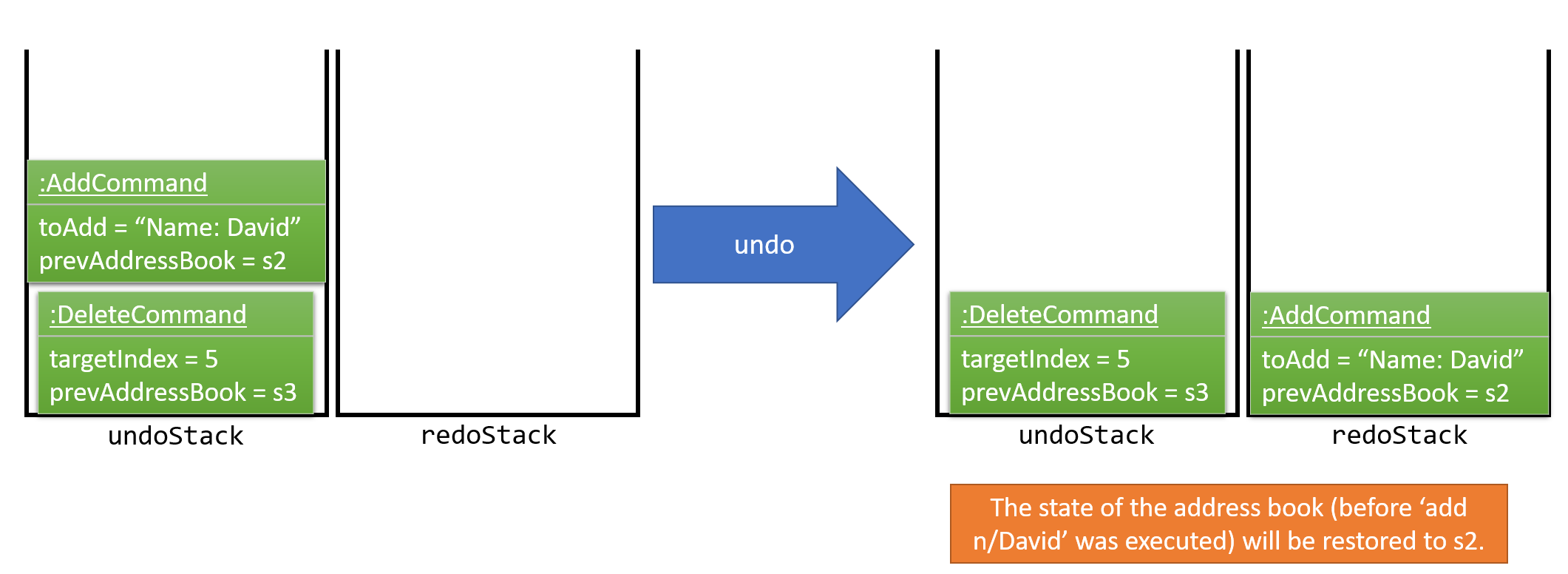
Figure 4.5.3 : Stack Diagram of Undo Execution
If the undoStack is empty, then there are no other commands left to be undone, and an Exception will be thrown when popping the undoStack .
|
The following sequence diagram shows how the undo operation works:
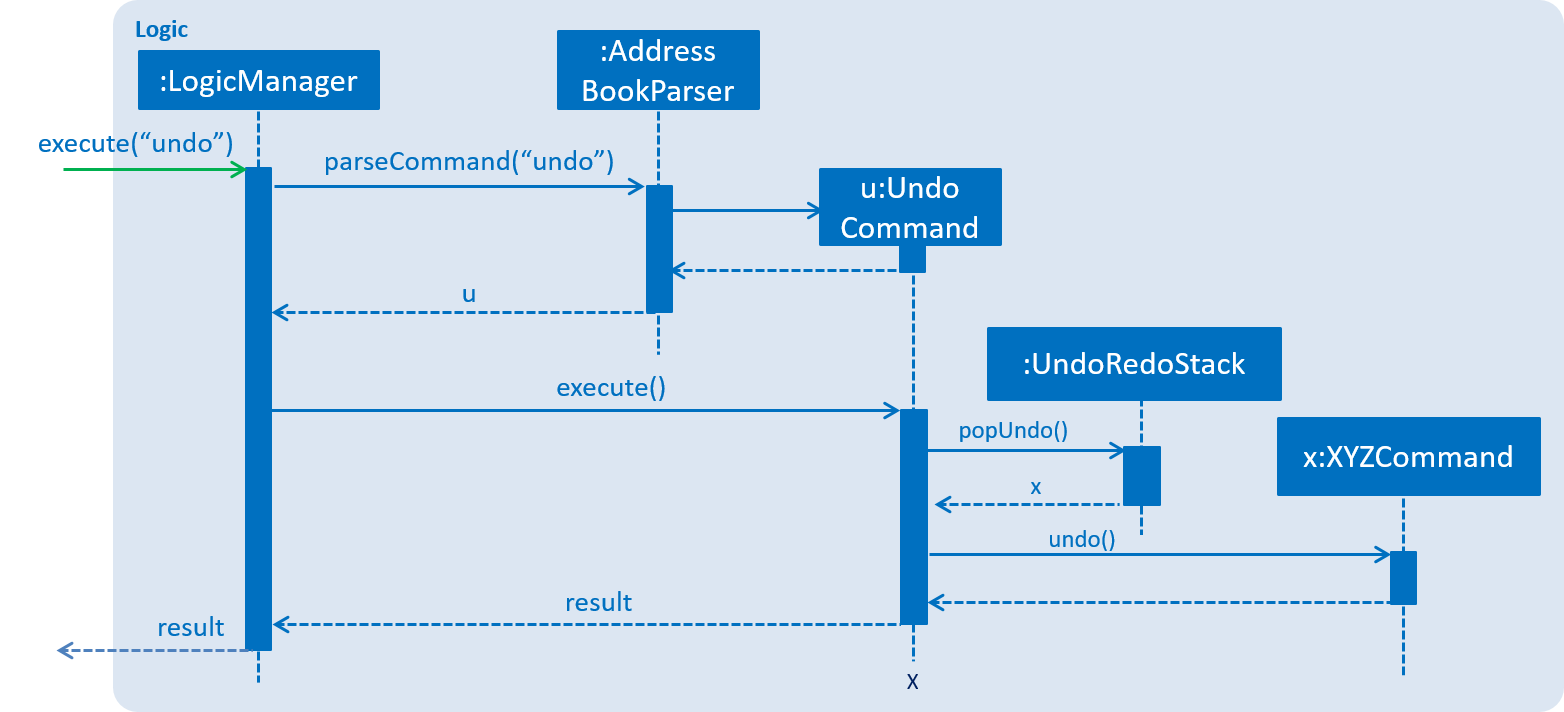
Figure 4.5.4 : Sequence Diagram of UndoRedo Feature
The redo does the exact opposite (pops from redoStack
, push to undoStack
, and restores the address book to the state after the command is executed).
If the redoStack is empty, then there are no other commands left to be redone, and an Exception will be thrown when popping the redoStack .
|
The user now decides to execute a new command, clear
. As before, clear
will be pushed into the undoStack
. This time the redoStack
is no longer empty. It will be purged as it no longer make sense to redo the add n/David
command (this is the behavior that most modern desktop applications follow).
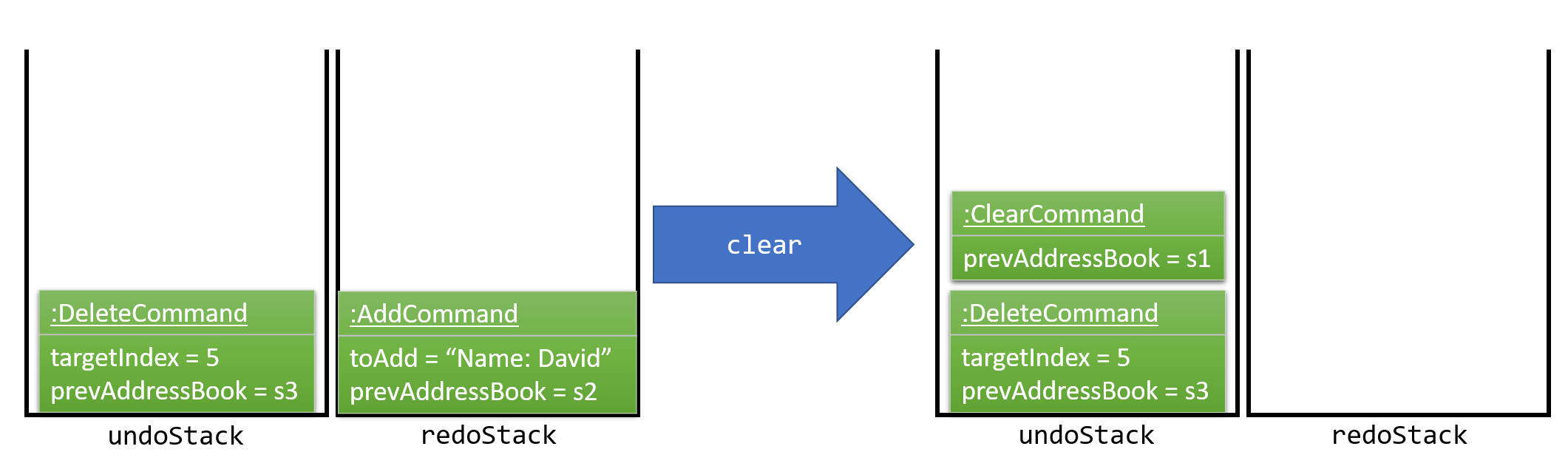
Figure 4.5.5 : Stack Diagram when a new Command, Clear is Executed
Commands that are not undoable are not added into the undoStack
. For example, list
, which inherits from Command
rather than UndoableCommand
, will not be added after execution:
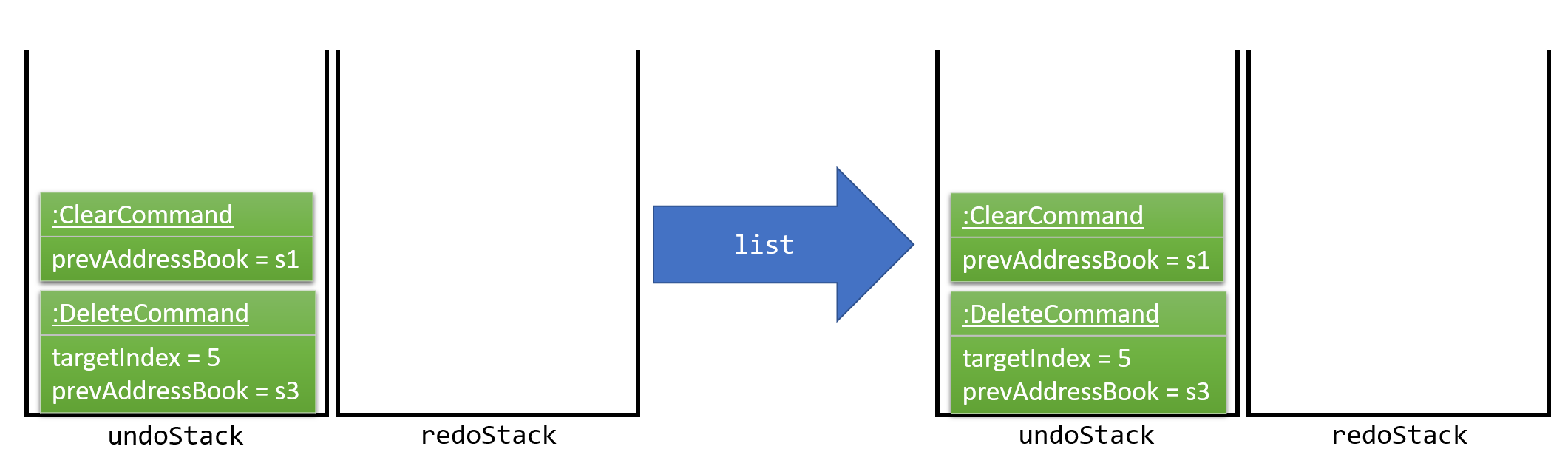
Figure 4.5.6 : Stack Diagram when Undoable Command is Executed
The following activity diagram summarize what happens inside the UndoRedoStack
when a user executes a new command:
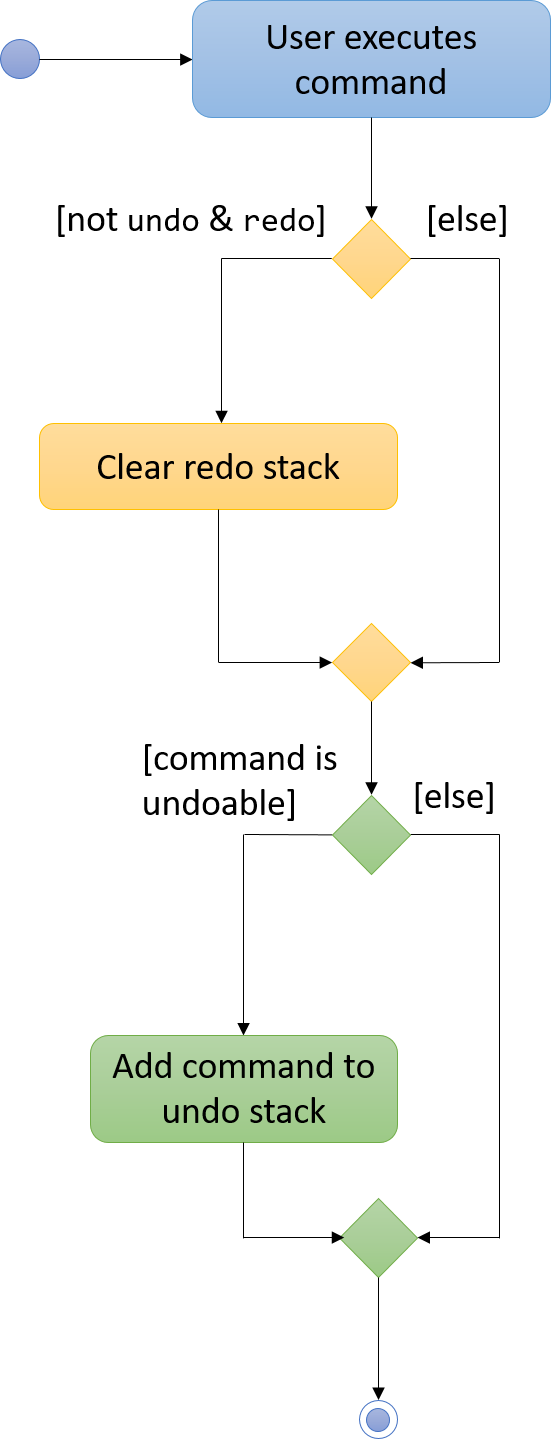
Figure 4.5.7 : Activity Diagram of UndoRedoStack
4.5.1. Design Considerations
Aspect: Implementation of UndoableCommand
Alternative 1 (current choice): Add a new abstract method executeUndoableCommand()
.
Pros: We will not lose any undone/redone functionality as it is now part of the default behaviour. Classes that deal with Command
do not have to know that executeUndoableCommand()
exist.
Cons: Hard for new developers to understand the template pattern.
Alternative 2: Just override execute()
.
Pros: Does not involve the template pattern, easier for new developers to understand.
Cons: Classes that inherit from UndoableCommand
must remember to call super.execute()
, or lose the ability to undo/redo.
Aspect: How undo & redo executes
Alternative 1 (current choice): Saves the entire address book.
Pros: Easy to implement.
Cons: May have performance issues in terms of memory usage.
Alternative 2: Individual command knows how to undo/redo by itself.
Pros: Will use less memory (e.g. for delete
, just save the person being deleted).
Cons: We must ensure that the implementation of each individual command are correct.
Aspect: Type of commands that can be undone/redone
Alternative 1 (current choice): Only include commands that modifies the address book (add
, clear
, edit
).
Pros: We only revert changes that are hard to change back (the view can easily be re-modified as no data are lost).
Cons: User might think that undo also applies when the list is modified (undoing filtering for example), only to realize that it does not do that, after executing undo
.
Alternative 2: Include all commands.
Pros: Might be more intuitive for the user.
Cons: User have no way of skipping such commands if he or she just want to reset the state of the address book and not the view.
Additional Info: See our discussion here.
Aspect: Data structure to support the undo/redo commands
Alternative 1 (current choice): Use separate stack for undo and redo.
Pros: Easy to understand for new Computer Science student undergraduates to understand, who are likely to be the new incoming developers of our project.
Cons: Logic is duplicated twice. For example, when a new command is executed, we must remember to update both HistoryManager
and UndoRedoStack
.
Alternative 2: Use HistoryManager
for undo/redo.
Pros: We do not need to maintain a separate stack, and just reuse what is already in the codebase.
Cons: Requires dealing with commands that have already been undone: We must remember to skip these commands. Violates Single Responsibility Principle and Separation of Concerns as HistoryManager
now needs to do two different things.
4.6. Sort mechanism
The reason for the implementation of sort allows the user to organise their list of contacts in cherBook more easily.
This provides an alternative for them to view their contacts instead of the usual way - most recent added contact being added to the last index of the list.
Users will find it easier to browse through their list of contacts in situations where the find
command cannot be used.
The sort
mechanism is implemented using SortCommand
. It supports the sorting of contact names in cherBook according to alphabetical order from A to Z.
When the user calls for sorting to be implemented, an empty ArrayList
would be created. Under the Model
component, UniquePersonList
would compare the names of all persons in lower case using a comparator and return an Observable List
containing of ReadOnlyPerson
s.
SortCommand
inherits from Command
.
SortCommand
is implemented this way:
public class SortCommand extends Command {
@Override
public CommandResult execute() {
// ... sort logic ...
}
}
The sort is case insensitive.
|
The following sequence diagram shows how the sort
operation works:
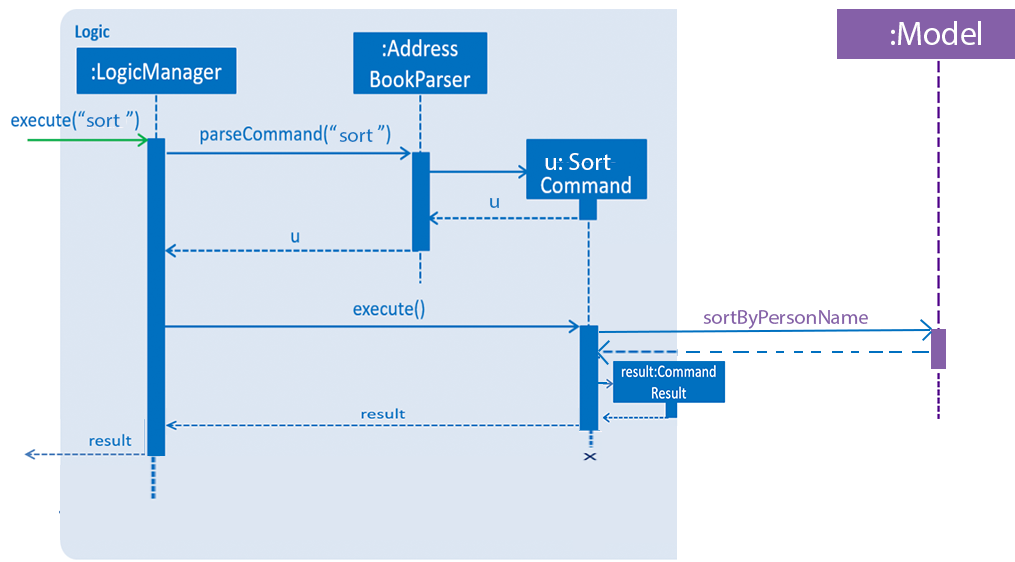
Figure 4.6.1 : Sequence Diagram of Sort Operation
When the user calls ‘sort’ or ‘st’ to sort
the contacts, the Model
will take in an ArrayList
named contactList from the Parser
.
Upon a successful sort
, all contacts in cherBook are displayed in alphabetical order.
If an empty list is given, the address book will return with the message: “No contacts to be sorted”.
If the list is already in the correct alphabetical order, the address book will return with the following message: “All contacts are sorted alphabetically by name”.
The following code will show how the list is being returned to the user:
/**
* Returns an observable list as as an unmodifiable {@code ObservableList}
*/
public ObservableList<ReadOnlyPerson> asObservableListSortedByName() {
internalList.sort((o1, o2) ->((o1.getName().toString().toLowerCase())
.compareTo(o2.getName().toString().toLowerCase())));
return FXCollections.unmodifiableObservableList(mappedList);
}
The sort mechanism is implemented this way to ensure that all person names in the UniquePersonList
is compared to at the lowest level of abstraction.
4.6.1. Design Considerations
Aspect: Implementation of SortCommand
Alternative 1 (current choice): SortCommand
inherits from Command.
Pros: After sorting his/her contacts, it remains sorted and the original list of unsorted contacts is replaced by this list.
Cons: The user is unable to save the list of contacts in the original order(unsorted format).
Alternative 2: Implement undo/redo function for SortCommand.
Pros: When undo is called, the list will return back to the most recent unsorted list. When redo is called, the list will return back to the sorted list of contacts.
Cons: The user is able to alter the list easily and may get confused whether is the current list the most recent sorted list.
Aspect: How sort executes
Alternative 1 (current choice): Uses the comparator
in Java Collections
to sort the arrays in alphabetical order.
Pros: Easy to implement.
Cons: Since method is inherited, it is difficult to debug and the only way is via the usage of vigorous user testing and logging.
Alternative 2: Write a sort
algorithm.
Pros: Case sensitivity can be accounted for and debugging is made simpler.
Cons: More error-proned as algorithm’s logic must be checked constantly to ensure that it accounts for all cases.
Aspect: Data structure to support the sort
command
Alternative 1 (current choice): Creates an empty ArrayList
to obtain the names from the UniquePersonList
before sorting occurs. The sort
overwrites the existing list of unsorted contacts.
Pros: The original list of unsorted contacts is not altered.
Cons: Additional space usage and longer time needed to overwrite the original contact list, inefficiency and memory wastage may arise.
Alternative 2: Creates a new list of persons to sort each time a command that filters the person list is entered.
Pros: User can sort accordingly to the filtered person list that he/she has implemented. Able to revert any changes if user does not want their contact list to be sorted.
Cons: User have to continously use the sort command for every filtered person list change. Inefficient if the user wants their contact list to be sorted at all times.
4.7. Add Schedule mechanism
The schedule
mechanism is implemented using ScheduleCommand
. It supports the scheduling of contacts in cherBook for better management of time.
The reason for the implementation of scheduling is to allow cherBook users (teacher/educator) to add schedules they have like consultations or meetings with their students or fellow colleagues.
With this scheduling command, users are able add their schedules with any person from their list of contacts just by typing the index of the person, the date and time of the schedule.
The date and time can be entered in natural language, helping users save their time to manually pen down their scheduled dates and timings.
When the user calls for scheduling to be implemented, two arguments would have to be passed into the constructor.
The two arguments are Name of the person being scheduled and the Calendar date with time.
Under the Model
component, the created schedule
is directly added into the address book.
ScheduleCommand
inherits from Command
.
ScheduleCommand is implemented this way:
public class ScheduleCommand extends Command {
@Override
public CommandResult execute() {
// ... schedule logic ...
}
}
Adding of schedules into the AddressBook can be done in natural language.
|
The following sequence diagram shows how the schedule
operation works:
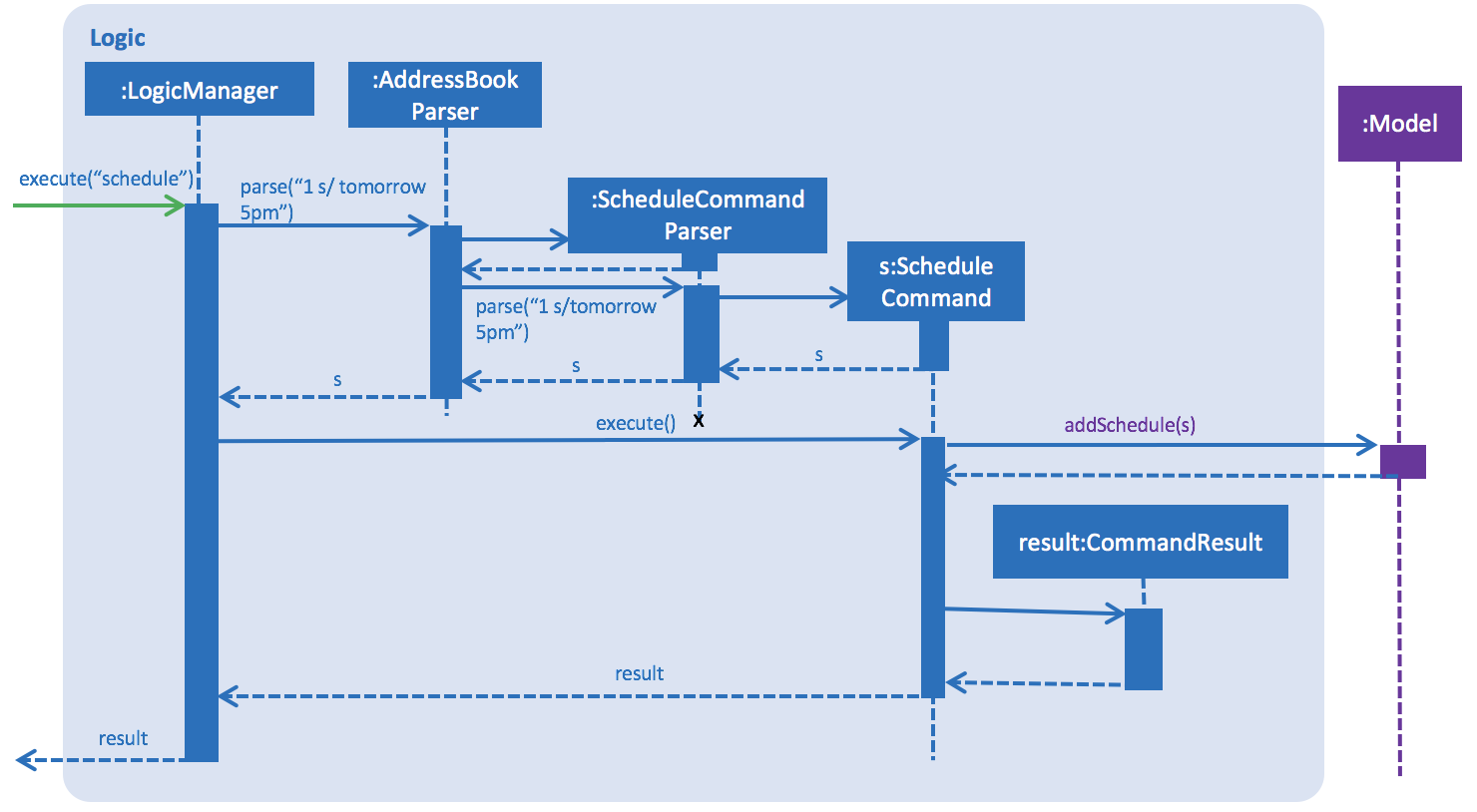
Figure 4.7.1 : Sequence Diagram of Schedule Operation
When the user calls ‘schedule’ or ‘sch’ to schedule the contacts in cherBook, the Model will receive a schedule from the Parser.
Upon successful scheduling, contacts are added into the set of schedules
.
If one or more arguments required to schedule a person is missing, the AddressBook will return with error messages corresponding to the missing arguments.
If one or more arguments required to schedule a person is invalid, the AddressBook will return with error messages corresponding to the invalid arguments.
The following will show how the schedule
is added into the AddressBook:
/**
* Adds a schedule for a student's consultation
*/
@Override
public void addSchedule(Schedule schedule) {
addressBook.addSchedule(schedule);
addressBook.sortSchedules();
indicateAddressBookChanged();
indicateScheduleListChanged();
}
It is implemented this way to ensure that all schedules
are added into the AddressBook with the lowest level of abstraction.
4.7.1. Design Considerations
Aspect: Implementation of ScheduleCommand
Alternative 1 (current choice): ScheduleCommand
inherits from Command.
Pros: After scheduling the contacts, the schedules remain in the order they are entered into the AddressBook.
Cons: The user is unable to sort the list of schedules according to chronological order.
Alternative 2: ScheduleCommand
inherits from Command.
Pros: After scheduling the contacts, the schedules remain in the order they are entered into the AddressBook.
Cons: The user is unable to sort the list of schedules according to chronological order.
Aspect: How schedule
executes
Alternative 1 (current choice): Adds a schedule
into the schedules list
once the person name and calendar date is parsed.
Pros: Easy to implement.
Cons: Vigorous user testing and logging is required to debug corner test cases because there is little control over imported APIs.
Aspect: Data structure to support the schedule
command
Alternative 1 (current choice): The list of schedules is altered directly from cherBook and is a standalone class.
Pros: Without association with any other classes, it is easy to implement and debug. Performance of program is fast because the list of schedules is retrieved directly from cherBook.
Cons: The user would not be able to view information of the schedules directly from each contact.
Alternative 2: Add each schedule into the contact as an attribute.
Pros: The user would be able to view the schedules directly from the respective contacts.
Cons: Performance of program would be affected, because to retrieve the whole list of schedules, the algorithm would have to iterate through all the contacts in cherBook.
4.8. View Schedule mechanism
The viewschedule
mechanism is implemented using ViewScheduleCommand
. It displays the schedules of the user of cherBook.
The reason for the implementation of viewing schedules is to allow cherBook users (teacher/educator) to keep track of their schedules as they often have many consultations or meetings with their students or fellow colleagues.
With this view schedule command, users are able to view all their schedules at once, sorted by date and time.
This way, the user is able to maintain their timetable easily.
When the user calls to view all the schedules, the 'Model'
component would return with the set of schedules that was previously added into cherBook.
ViewScheduleCommand is implemented this way:
public class ViewScheduleCommand extends Command {
@Override
public CommandResult execute() {
// ... view schedule logic ...
}
}
The schedules would be listed in the ResultDisplay
panel, and each schedule would be separated with a new line.
Aspect: Data structure to support the ViewSchedule
command
Alternative 1 (current choice): The list of schedules is obtained directly from the Model
before it is being displayed on the ResultDisplay
panel.
Pros: It is easy to implement and debug. Performance of program is fast because the list of schedules is retrieved directly from the Model
.
Cons: The user do not have the alternative to view information of the schedules from each individual contact.
4.9. Home Page mechanism
Home
mechanism is implemented using HomeCommand.
It displays the home page when the user enter the command and each time a randomly selected wallpaper and quote would be displayed.
When the home page is displayed, the extendedPersonCard, StatsPanel and GraphPanel would be switched out. Only when the user select a new person would the three panes swap back.
As we would be focusing on CLI implementations, we did not enable a way to select a person through clicking when the home page is displayed. |
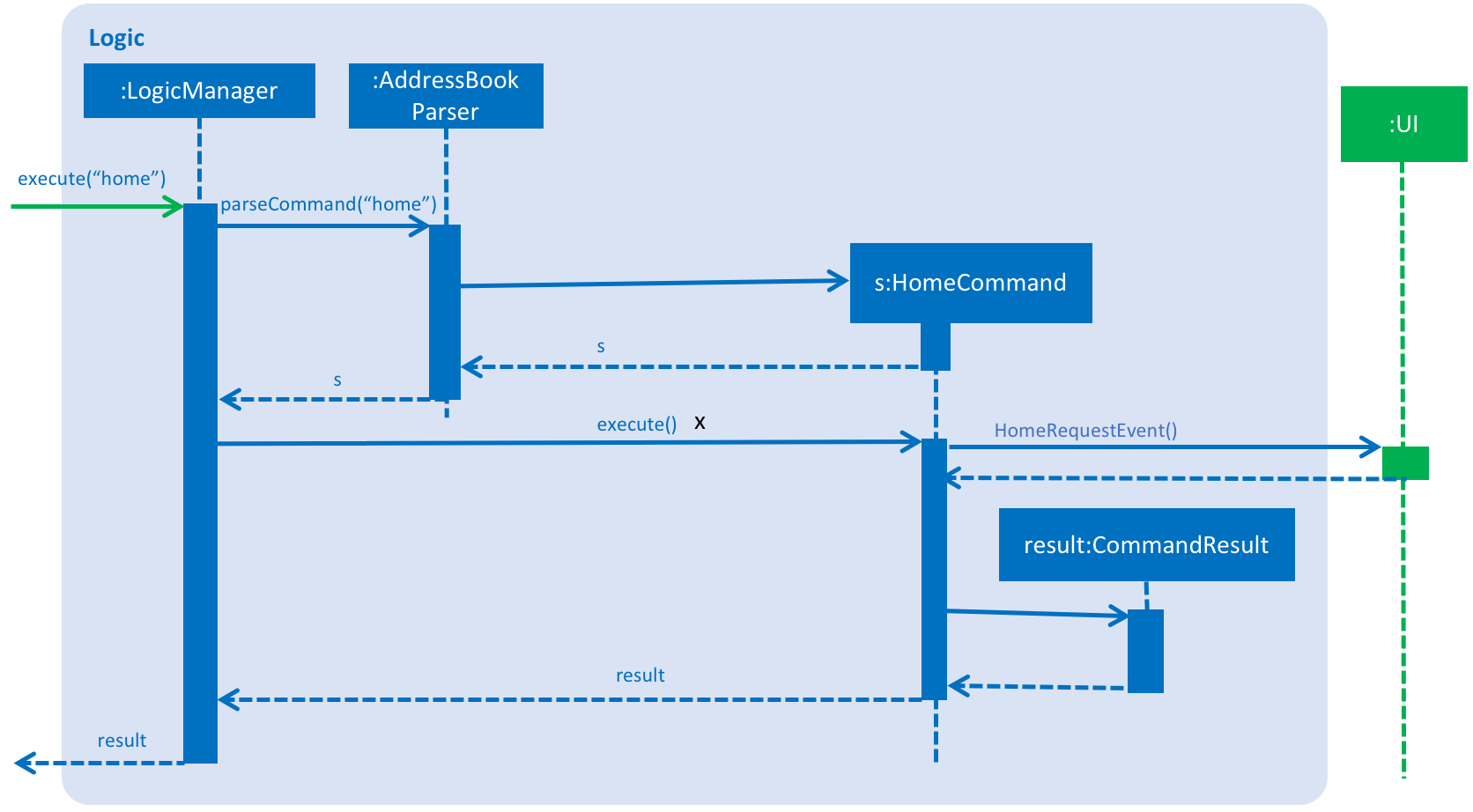
Figure 4.9.1 : Sequence Diagram of home
Operation
4.9.1. Design Considerations
Aspect: To have a soft security feature.
Alternative 1 (current choice): Disable easy access to data, only users who knows the command are able to view.
Pros: Easy to implement.
Cons: Easier to be break through
Alternative 2: Have a login page before displaying the main window.
Pros: The application as a whole would be more secure.
Cons: Takes a longer time to implement.
---
4.10. GraphPanel Mechanism
LineChart
mechanism is implemented using javaFX
. It takes in FilteredPersonList
and plots a graph based on those who are in the same formClass
.
Using XYChart.series
to store the data of the persons name and grade, a coordinate
is created and a line
is drawn connecting all the coordinates
.
These coordinates are sorted in ascending order so that the user is able to identify those in the lower quartile.
The LineChart
mechanism is implemented this way:
for (ReadOnlyPerson people : people) { if (people.getFormClass().equals(person.getFormClass())) { series.getData().add(new XYChart.Data<>(people.getName().toString(), Double.parseDouble(people.getGrades().toString()))); } } series.getData().sort(Comparator.comparingDouble(d -> d.getYValue())); //...other logic...
4.10.1. Design Considerations
Aspect: Graph to be displayed for an effective visual aid
Alternative 1 (current choice): Line chart to display the grades of student.
Pros: Easier to implement.
Cons: May not be the most appropriate way to display grades of students.
Alternative 2: Bell curve to display the grades of students.
Pros: A better representation of the student grades and it is easier to interpret.
Cons: Much harder to plot a graph based on statistics then raw data.
4.11. Tabs mechanism
Tabs
mechanism is implemented using TabsCommand.
It swaps tab panel between lineChart and barChart based on the index input by the user.
When the user types tab [INDEX]
, it creates raises new event
named JumpToTabRequestEvent
, which prompts the subscribed
method in GraphPanel
to change the tab page based on[INDEX].
The number of valid index is based on the number of tabs created, in this case it would be 2. A invalid index would be displayed if the index provided is out of bounds. |
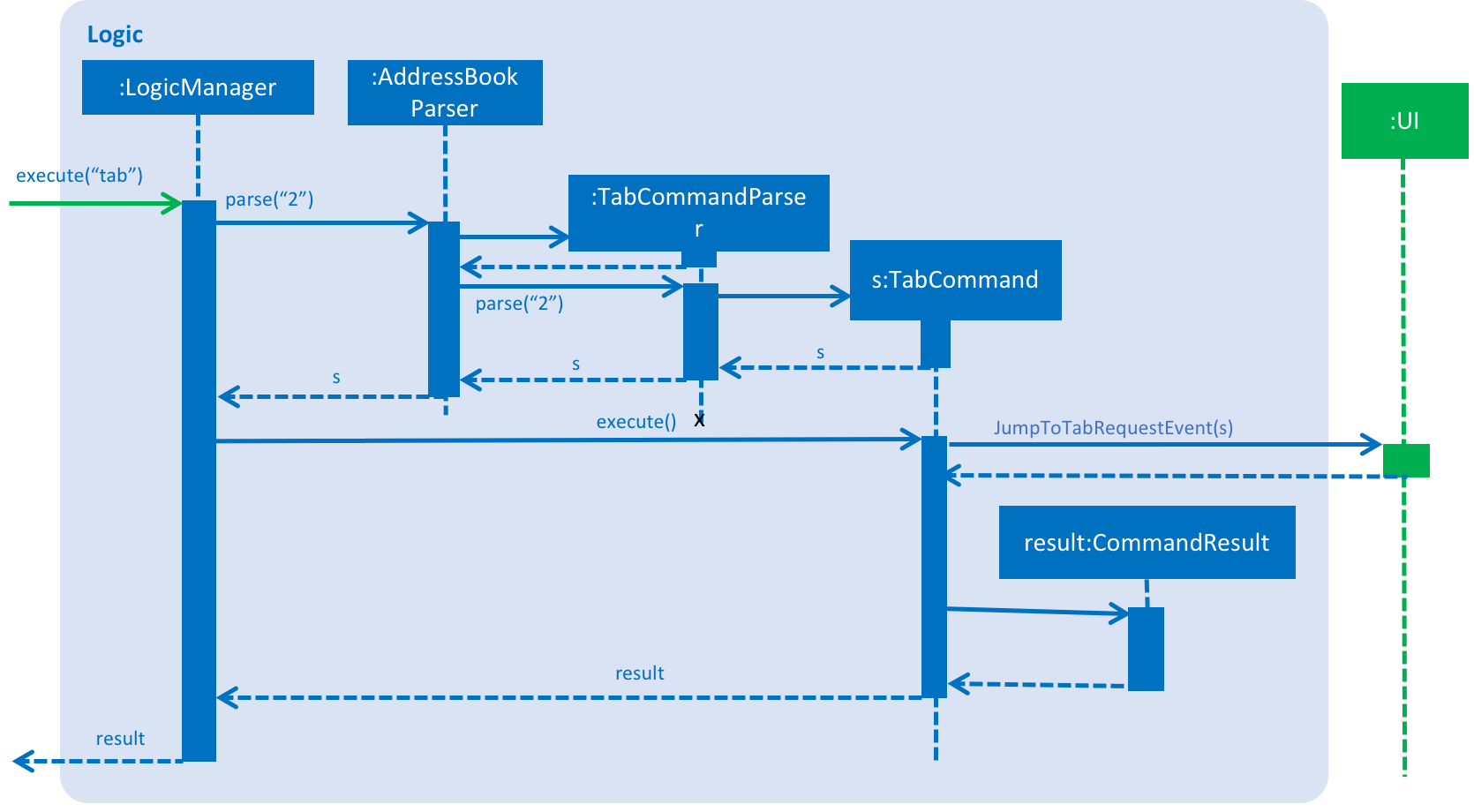
Figure 4.11.1 : Sequence Diagram of Tab
Operation
4.11.1. Design Considerations
Aspect: To display different chart types to help visual aid.
Alternative 1 (current choice): Do a TabPane.
Pros: More versatile and easier to further expand in the future.
Cons: More cumbersome to start.
Alternative 2: Multiple graphs on the same pane.
Pros: A easier comparison of the student grades between different graph types.
Cons: Difficult to further expand as there are limited space. Graph may be shrinked as well.
4.12. Statistics Panel mechanism
The StatisticsPanel
class inherits from the UiPart
class.
It displays to users the statistics of the current list of students in the StudentsPanel
element of the user interface.
The Statistics Panel
is implemented using the Statistics
class and The FilteredPersonListChangedEvent
event.
The Statistics
class supports the calculating of statistics for the current list of students in the PersonListPanel
and
the FilteredPersonListChangedEvent
indicates that the FilteredPersonList in the model has changed and is used to update the Statsistics Panel
.
The following activity diagram shows how the StatisticsPanel mechanism works:
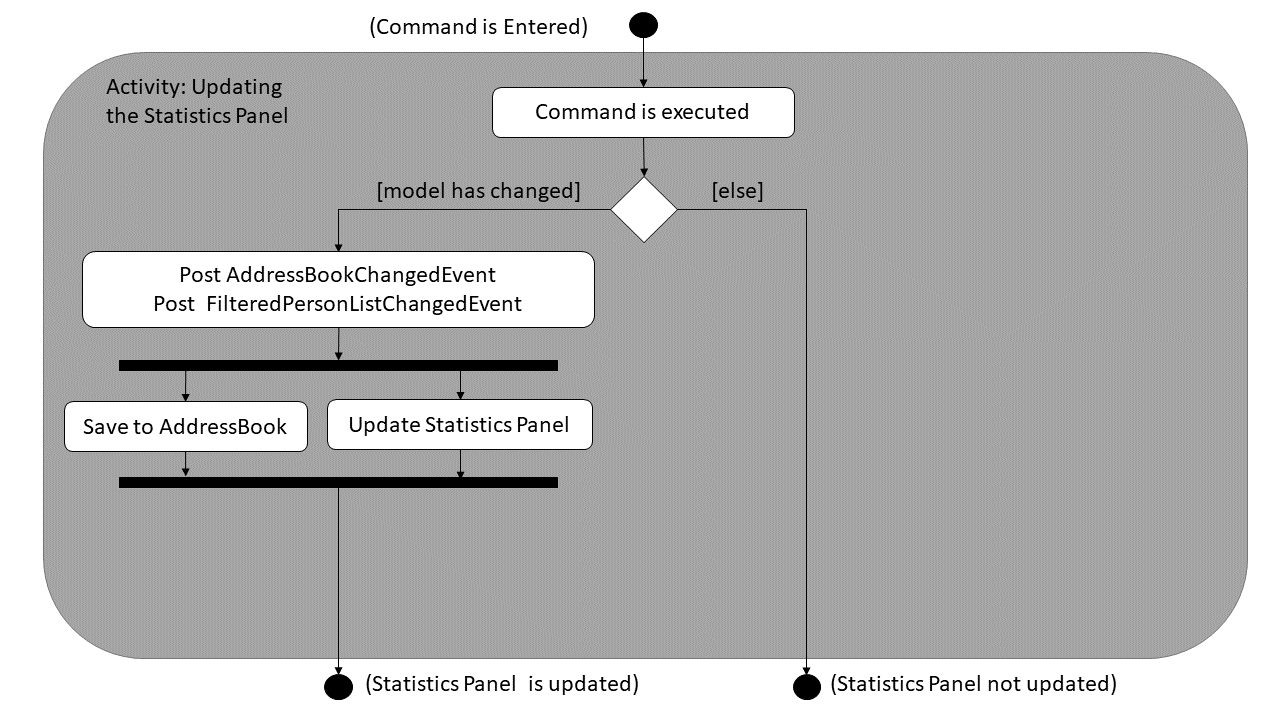
Figure 4.12.1 : Activity Diagram of updating the`Statistics Panel` .
Whenever the user enters any Command
that modifies the addressBook data, the Model
Component posts
a FilteredPersonListChangedEvent
containing the filteredPersonsList
to the EventsCenter
.
At the same time, an AddressBookChangedEvent
that indicates that the model has changed is also posted to the EventsCenter
.
The FilteredPersonListChangedEvent
is implemented this way:
public class FilteredPersonListChangedEvent extends BaseEvent {
private final ObservableList<ReadOnlyPerson> currentFilteredList;
public FilteredPersonListChangedEvent(ObservableList<ReadOnlyPerson> currentFilteredList) {
this.currentFilteredList = currentFilteredList;
}
@Override
public String toString() {
return this.getClass().getSimpleName();
}
public ObservableList<ReadOnlyPerson> getCurrentFilteredPersonList() {
return currentFilteredList;
}
}
Meanwhile in the StatisticsPanel
class, the handleFilteredPersonListChangedEvent
event handler which is subscribed to EventsCenter
handles the FilteredPersonListChangedEvent
event.
The handleFilteredPersonListChangedEvent
event handler is implemented this way:
@FXML
@Subscribe
private void handleFilteredPersonListChangedEvent(FilteredPersonListChangedEvent event) {
logger.info(LogsCenter.getEventHandlingLogMessage(event));
statistics.initScore(event.getCurrentFilteredPersonList()); // Updates the statistics instance values
loadListStatistics();
}
When handling the event, the Statistics
instance in StatisticsPanel
has its values updated by the initScore()
method.
This means that the values returned from the Statistics
class’s getter methods when called are now updated.
The loadListStatistics()
method in StatisticsPanel
is then called.
The loadListStatistics()
is implemented this way:
/**
* Updates list Statistics in the Statistics panel
*/
protected void loadListStatistics() {
mean.setText(statistics.getMeanString());
median.setText(statistics.getMedianString());
mode.setText(statistics.getModeString());
variance.setText(statistics.getVarianceString());
standardDeviation.setText(statistics.getStdDevString());
quartile1.setText(statistics.getQuartile1String());
quartile3.setText(statistics.getQuartile3String());
interquartileRange.setText(statistics.getInterquartileRangeString());
}
The Labels
(texts) in the User Interface are set according to the updated Statistics
instance’s getter methods.
This updates and shows the statistic values of the current filteredPersonList
on the StatisticsPanel
in the User Interface.
At the same time, the AddressBookChangedEvent
is also being handled, saving the addressBook data.
4.12.1. Design Considerations
Aspect: How to update the Statistics Panel
Alternative 1 (current choice): Create a new FilteredPersonListChangedEvent
just for updating the Statistics Panel
.
Pros: Adherence to the Single Responsibility Principle (SRP) increases cohesion among the different Events
and reduces coupling.
Cons: Increases the number of classes in the source code, making it more complex according to the More Is More Complex (MIMC) principle.
Alternative 2: Modify AddressBookChangedEvent
to also update the Statistics Panel
.
Pros: Since a change in the model
triggers both the AddressBookChangedEvent
and the updating of the Statistics Panel
,
only a few changes to are needed for AddressBookChangedEvent
to do also update the Statistics Panel
.
Cons: This violates the Single Responsibility Principle (SRP) by having giving AddressBookChangedEvent
the additional responsibility of updating the Statistics Panel
.
Aspect: How to raise the FilteredPersonListChangedEvent
in the ModelManager
Alternative 1 (current choice): Create a separate updateStatisticsPanel()
method to raise the event to update the Statistics Panel
Pros: Reduces coupling and increases cohesion by giving a single responsibility to the updateStatisticsPanel()
method.
Cons: Makes the application more complex to understand according to the More Is More Complex (MIMC) principle.
Alternative 2: Raise the FilteredPersonListChangedEvent
together with the AddressBookChangedEvent
Pros: Reduces the number of similar methods in the source code, making it less complex and easier to understand
Cons: Increases coupling in the source code
4.13. Unique Color Tag mechanism
The unique color tag
mechanism is facilitated by making changes to PersonCard
class in the UI
component. This feature enables
the assignment of unique colors to tags.
The color generated to be assigned to tags is random and all tags with the same description will be assigned a same color.
The following sequence diagram shows how the unique color tag
operation works:
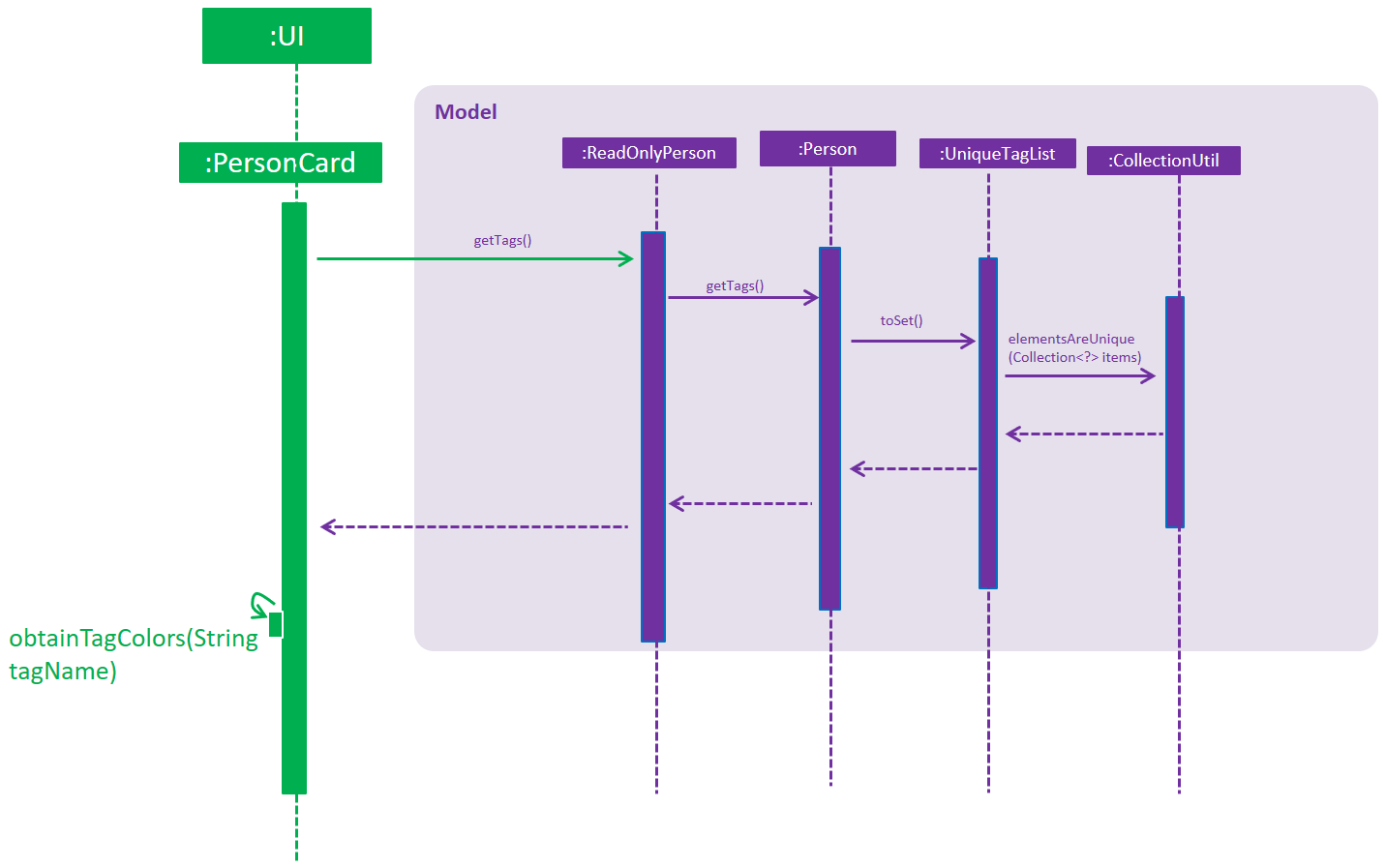
Figure 4.13.1 : Sequence Diagram of Unique Color Tag Operation
The method receives String tagName
(description of the tag) and returns the String assignedColor
associated to the tagName. It is shown in the code segment below.
public static String obtainTagColors(String tagName) { if (!currentTagColors.containsKey(tagName)) { do { Random random = new Random(); final float hue = random.nextFloat(); final float saturation = 0.65f + random.nextFloat() * (0.90f - 0.65f); final float luminance = 0.60f + random.nextFloat() * (0.90f - 0.60f); Color color = Color.getHSBColor(hue, saturation, luminance); Formatter hexRepresentation = new Formatter(new StringBuffer("#")); hexRepresentation.format("%02X", color.getRed()); hexRepresentation.format("%02X", color.getGreen()); hexRepresentation.format("%02X", color.getBlue()); assignedColor = hexRepresentation.toString(); } while (usedColors.contains(assignedColor)); usedColors.add(assignedColor); currentTagColors.put(tagName, assignedColor); } return currentTagColors.get(tagName); }
The section below explains how various parts of the above code segment works.
A color is determined by three factors, hue
, saturation
and luminance
.
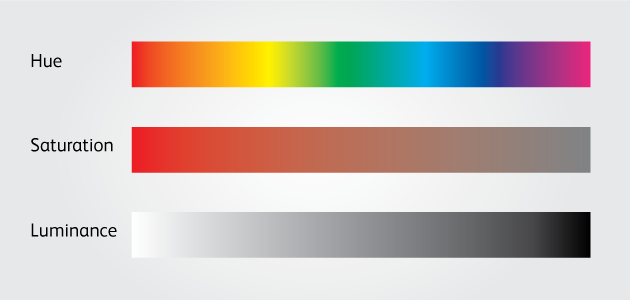
Figure 4.13.2 : Color Range of Hue, Saturation and Luminance
The code segment shown below randomises the value of each of the three factors,
and a random color
is obtained.
Random random = new Random(); final float hue = random.nextFloat(); final float saturation = 0.65f + random.nextFloat() * (0.90f - 0.65f); final float luminance = 0.60f + random.nextFloat() * (0.90f - 0.60f); Color color = Color.getHSBColor(hue, saturation, luminance);
Random range of saturation and luminance are tweaked to ensure that the random color generated will always mesh well with the white font of
the tag description.
|
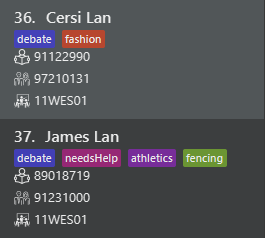
Figure 4.13.3 : Compatibility of tag color background with white font of tag description
Next, in the code segment below, the red
, green
and blue
components are extracted from the color
. It is then formatted into a hexadecimal
representation
of the color, before being converted to a String
data type and assigned to assignedColor
.
Hexadecimal representation of the color is compatible with JavaFx. |
Formatter hexRepresentation = new Formatter(new StringBuffer("#")); hexRepresentation.format("%02X", color.getRed()); hexRepresentation.format("%02X", color.getGreen()); hexRepresentation.format("%02X", color.getBlue()); assignedColor = hexRepresentation.toString();
The assignedColor
is added into an ArrayList<String> usedColors
. This ensures no same color will be assigned
to a tag with different description in subsequent iterations by randomising a different color again, should a same color is
generated. The method will then return assignedColor
.
Lastly, in the code below, obtainTagColors(tag.tagName)
, the hexadecimal String representation of the color, will be appended
to the tag background color. This sets the background color of the tag to be that of the assigned color.
private void initialiseTags(ReadOnlyPerson person) { person.getTags().forEach(tag -> { Label tagLabel = new Label(tag.tagName); tagLabel.setStyle("-fx-background-color: " + obtainTagColors(tag.tagName)); tags.getChildren().add(tagLabel); }); }
4.13.1. Design Considerations
Aspect: How tag colors are assigned.
Alternative 1 (current choice): Tag colors are assigned randomly.
Pros: Easy for developers to understand the mechanism of this feature.
Cons: User has no control over the assigning of tag colors.
Alternative 2: Tag colors are assigned by the user.
Pros: User will have more freedom in personalising the tags.
Cons: The range of colors will be more limited.
4.14. Logging
We are using java.util.logging
package for logging. The LogsCenter
class is used to manage the logging levels and logging destinations.
-
The logging level can be controlled using the
logLevel
setting in the configuration file (See Configuration) -
The
Logger
for a class can be obtained usingLogsCenter.getLogger(Class)
which will log messages according to the specified logging level -
Currently log messages are output through:
Console
and to a.log
file.
Logging Levels
-
SEVERE
: Critical problem detected which may possibly cause the termination of the application -
WARNING
: Can continue, but with caution -
INFO
: Information showing the noteworthy actions by the App -
FINE
: Details that is not usually noteworthy but may be useful in debugging e.g. print the actual list instead of just its size
4.15. Configuration
Certain properties of the application can be controlled (e.g App name, logging level) through the configuration file (default: config.json
).
5. Unused/Removed Features
In this section, you will see features/implementations that are removed before the release of the final version. They will be accompanied by a link to their unused code should you need them for your future improvements of cherBook.
5.1. Add Student/Parent Phone Contact mechanism [Functional code]
The student/parent phone contact
mechanism is facilitated by making changes
to the Phone class in the model component. It enables the input
of the phone contact information of the student and his/her parent.
To ensure consistency across the user inputs for add command, the user will have to input p/ student/(STUDENT_NUMBER) parent/(PARENT_NUMBER) instead of p/ Student: (STUDENT_NUMBER) Parent: (PARENT_NUMBER).
The following sequence diagram shows how the student/parent phone contact
operation works:
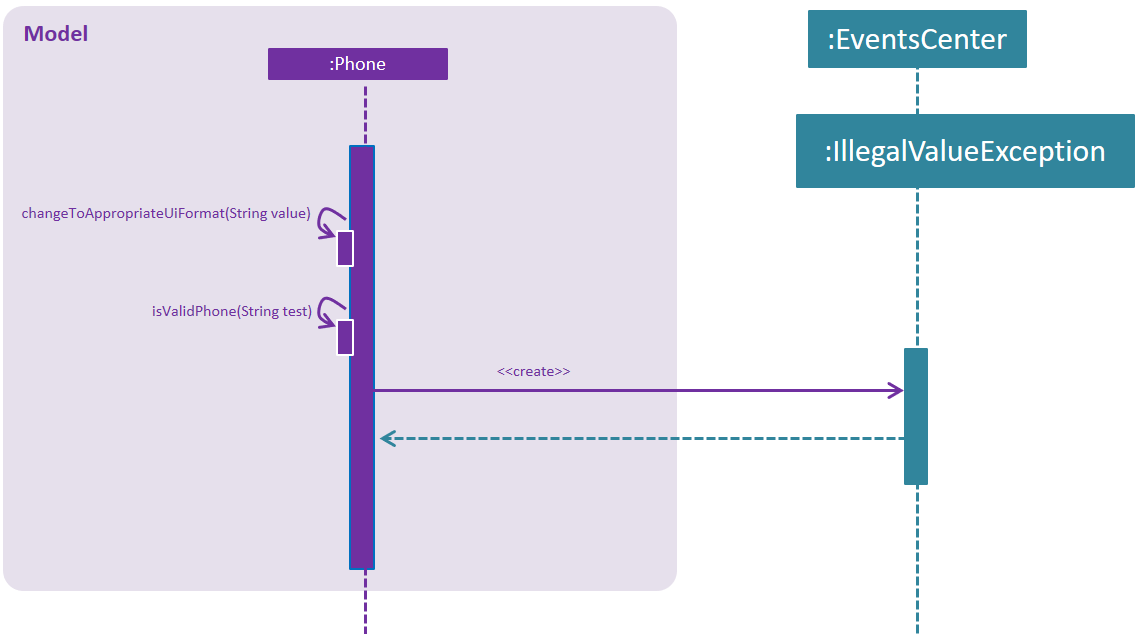
Figure 5.1.1 : Sequence Diagram of Student/Parent Phone Contact Operation
The following method replaces /
with :
, s
with S
, and p
with P
in the user input string
for appropriate presentation of displayed person card phone information
:
public static String changeToAppropriateUiFormat(String value) {
value = value.replace("/", ": ");
value = value.replace("s", "S");
value = value.replace("p", "P");
return value;
}
Validity of the displayed person card phone information
is checked in the following method in the Phone class:
public static boolean isValidPhone(String test) {
return test.matches(PHONE_VALIDATION_REGEX);
}
where the PHONE_VALIDATION_REGEX
refers to:
public static final String PHONE_VALIDATION_REGEX =
"((Student: )(\d\d\d\d\d\d\d\d)( Parent: )(\d\d\d\d\d\d\d\d))|((Parent: )(\d\d\d\d\d\d\d\d))";
The regex ensures that the string for displayed person card phone information
adheres to the
correct format of Student: (STUDENT_NUMBER) Parent: (PARENT_NUMBER)
or Parent: (PARENT_NUMBER)
as it is optional to
add the phone number of students.
The phone numbers must be exactly 8 digits
long.
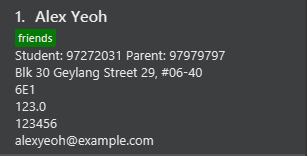
Figure 5.1.2 : Valid Displayed Person Card Phone Information
If the string format for displayed person card phone information
is valid, the
phone contact information of the student and his/her parent will be displayed
below the student’s name in the person card as seen in the figure above.

Figure 5.1.3 : Message to prompt Users to Enter Correct Phone Format
If the string format for displayed person card phone information
is invalid, an IllegalValueException
is thrown. The user will be prompted to follow the accepted format as seen in the figure above.
public Phone(String phone) throws IllegalValueException {
requireNonNull(phone);
String trimmedPhone = phone.trim();
trimmedPhone = changeToAppropriateUiFormat(trimmedPhone);
if (!isValidPhone(trimmedPhone)) {
throw new IllegalValueException(MESSAGE_PHONE_CONSTRAINTS);
}
this.value = trimmedPhone;
}
5.1.1. Design Considerations
Aspect: Phone numbers
Alternative 1 (current choice): Both the student’s phone number and their parent’s phone number is a single string.
Pros: Easy to implement as separate classes for student’s phone and parent’s phone is not required.
Cons: Harder to implement features that require solely the student’s number or parent’s number.
Alternative 2: Have a phoneStudent class for student’s phone number and phoneParent class for parent’s phone number.
Pros: Can edit properties of the student’s phone number or parent’s phone number separately.
Cons: Harder to implement as now there will be two phone classes instead of one.
Aspect: Validity of phone number
Alternative 1 (current choice): Only allow numbers that are exactly 8 digits
long.
Pros: Ensures consistency across all phone numbers.
Cons: Student’s parents might not have foreign numbers that are not exactly 8 digits
long.
Alternative 2: Modify phone validity regex to allow any length of phone numbers.
Pros: Able to have phone numbers that are local or non-local.
Cons: Inconsistent phone lengths displayed, might look very messy.
6. Documentation
In this section, we will be explaining how we edit and publish our documentation. We use asciidoc for writing documentation.
Asciidoc is chosen over Markdown because asciidoc, although a bit more complex than Markdown, provides more flexibility in formatting. |
6.1. Editing Documentation
You can look at UsingGradle.adoc to learn how to render .adoc
files locally to preview the end result of your edits.
Alternatively, you can download the AsciiDoc plugin for IntelliJ, which allows you to preview the changes you have made to your .adoc
files in real-time.
6.2. Publishing Documentation
You can look at UsingTravis.adoc to learn how to deploy GitHub Pages using Travis.
6.3. Converting Documentation to PDF format
Google Chrome is used for converting documentation to PDF format, as Chrome’s PDF engine preserves hyperlinks used in webpages.
Here are the steps to convert the project documentation files to PDF format.
-
Follow the instructions in UsingGradle.adoc to convert the AsciiDoc files in the
docs/
directory to HTML format. -
Go to your generated HTML files in the
build/docs
folder, right click on them and selectOpen with
→Google Chrome
. -
Within Chrome, click on the
Print
option in Chrome’s menu. -
Set the destination to
Save as PDF
, then clickSave
to save a copy of the file in PDF format. For best results, use the settings indicated in the screenshot below.
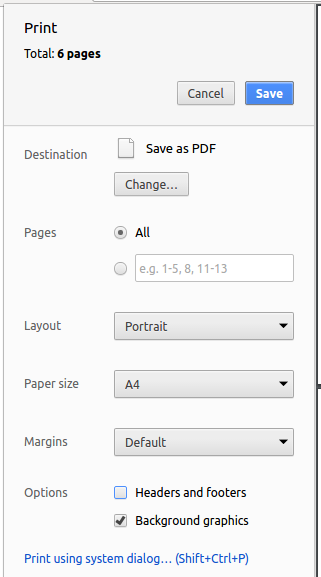
Figure 6.3.1 : Saving documentation as PDF files in Chrome
7. Testing
Having integrated unit tests that cover your API’s behaviour is important. It helps you verify the correctness, functional behaviour and usability of cherBook the app before you release it publicly. This section details the different types of tests cherBook uses and how to run them. Additionally, the troubleshooting section details the errors you might face while testing cherBook and different ways to solve them.
7.1. Running Tests
There are three ways to run tests.
The most reliable way to run tests is the third method. The first two methods might fail some GUI tests due to platform/resolution-specific idiosyncrasies. |
Method 1: Using IntelliJ JUnit test runner
-
To run all tests, right-click on the
src/test/java
folder and chooseRun 'All Tests'
-
To run a subset of tests, you can right-click on a test package, test class, or a test and choose
Run 'ABC'
Method 2: Using Gradle
-
Open a console and run the command
gradlew clean allTests
(Mac/Linux:./gradlew clean allTests
)
See UsingGradle.adoc for more info on how to run tests using Gradle. |
Method 3: Using Gradle (headless)
The TestFX library we use allows our GUI tests to be run in the headless mode. In the headless mode, GUI tests do not show up on the screen. Thus, the developer can do other things on the Computer while the tests are running.
To run tests in headless mode, open a console and run the command gradlew clean headless allTests
(Mac/Linux: ./gradlew clean headless allTests
)
7.2. Types of tests
We have two types of tests:
-
GUI Tests - These are tests involving the GUI. They include,
-
System Tests that test the entire App by simulating user actions on the GUI. These are in the
systemtests
package. -
Unit tests that test the individual components. These are in
seedu.address.ui
package.
-
-
Non-GUI Tests - These are tests not involving the GUI. They include,
-
Unit tests targeting the lowest level methods/classes.
e.g.seedu.address.commons.StringUtilTest
-
Integration tests that are checking the integration of multiple code units (those code units are assumed to be working).
e.g.seedu.address.storage.StorageManagerTest
-
Hybrids of unit and integration tests. These test are checking multiple code units as well as how the are connected together.
e.g.seedu.address.logic.LogicManagerTest
-
7.3. Troubleshooting Testing
Problem: HelpWindowTest
fails with a NullPointerException
.
-
Reason: One of its dependencies,
UserGuide.html
insrc/main/resources/docs
is missing. -
Solution: Execute Gradle task
processResources
by openingcmd
in thecherBook directory
and inserting the linegradlew processResources
.
8. Dev Ops
This section teaches you about tools building and provides you with instructions on how to test and release apps faster and more efficiently.
8.1. Build Automation
See UsingGradle.adoc to learn how to use Gradle for build automation.
8.2. Continuous Integration
We use Travis CI and AppVeyor to perform Continuous Integration on our projects. See UsingTravis.adoc and UsingAppVeyor.adoc for more details.
8.3. Making a Release
Here are the steps to create a new release.
-
Update the version number in
MainApp.java
. -
Generate a JAR file using Gradle.
-
Tag the repo with the version number. e.g.
v0.1
-
Create a new release using GitHub and upload the JAR file you created.
8.4. Managing Dependencies
A project often depends on third-party libraries. For example, Address Book depends on the Jackson library for XML parsing. Managing these dependencies can be automated using Gradle. For example, Gradle can download the dependencies automatically, which is better than these alternatives.
a. Include those libraries in the repo (this bloats the repo size)
b. Require developers to download those libraries manually (this creates extra work for developers)
Appendix A: Suggested Programming Tasks to Get Started
Suggested path for new programmers:
-
First, add small local-impact (i.e. the impact of the change does not go beyond the component) enhancements to one component at a time. Some suggestions are given in this section Improving a Component.
-
Next, add a feature that touches multiple components to learn how to implement an end-to-end feature across all components. The section Creating a new command:
remark
explains how to go about adding such a feature.
A.1. Improving each component
Each individual exercise in this section is component-based (i.e. you would not need to modify the other components to get it to work).
Logic
component
Do take a look at the Design: Logic Component section before attempting to modify the Logic component.
|
-
Add a shorthand equivalent alias for each of the individual commands. For example, besides typing
clear
, the user can also typec
to remove all persons in the list.
Model
component
Do take a look at the Design: Model Component section before attempting to modify the Model component.
|
-
Add a
removeTag(Tag)
method. The specified tag will be removed from everyone in the address book.
Ui
component
Do take a look at the Design: UI Component section before attempting to modify the UI component.
|
-
Use different colors for different tags inside person cards. For example,
friends
tags can be all in grey, andcolleagues
tags can be all in red.Before
After
-
Modify
NewResultAvailableEvent
such thatResultDisplay
can show a different style on error (currently it shows the same regardless of errors).Before
After
-
Modify the
StatusBarFooter
to show the total number of people in the address book.Before
After
Storage
component
Do take a look at the Design: Storage Component section before attempting to modify the Storage component.
|
-
Add a new method
backupAddressBook(ReadOnlyAddressBook)
, so that the address book can be saved in a fixed temporary location.
A.2. Creating a new command: remark
By creating this command, you will get a chance to learn how to implement a feature end-to-end, touching all major components of the app.
A.2.1. Description
Edits the remark for a person specified in the INDEX
.
Format: remark INDEX r/[REMARK]
Examples:
-
remark 1 r/Likes to drink coffee.
Edits the remark for the first person toLikes to drink coffee.
-
remark 1 r/
Removes the remark for the first person.
A.2.2. Step-by-step Instructions
[Step 1] Logic: Teach the app to accept 'remark' which does nothing
Let’s start by teaching the application how to parse a remark
command. We will add the logic of remark
later.
Main:
-
Add a
RemarkCommand
that extendsUndoableCommand
. Upon execution, it should just throw anException
. -
Modify
AddressBookParser
to accept aRemarkCommand
.
Tests:
-
Add
RemarkCommandTest
that tests thatexecuteUndoableCommand()
throws an Exception. -
Add new test method to
AddressBookParserTest
, which tests that typing "remark" returns an instance ofRemarkCommand
.
[Step 2] Logic: Teach the app to accept 'remark' arguments
Let’s teach the application to parse arguments that our remark
command will accept. E.g. 1 r/Likes to drink coffee.
Main:
-
Modify
RemarkCommand
to take in anIndex
andString
and print those two parameters as the error message. -
Add
RemarkCommandParser
that knows how to parse two arguments, one index and one with prefix 'r/'. -
Modify
AddressBookParser
to use the newly implementedRemarkCommandParser
.
Tests:
-
Modify
RemarkCommandTest
to test theRemarkCommand#equals()
method. -
Add
RemarkCommandParserTest
that tests different boundary values forRemarkCommandParser
. -
Modify
AddressBookParserTest
to test that the correct command is generated according to the user input.
[Step 3] Ui: Add a placeholder for remark in PersonCard
Let’s add a placeholder on all our PersonCard
s to display a remark for each person later.
Main:
-
Add a
Label
with any random text insidePersonListCard.fxml
. -
Add FXML annotation in
PersonCard
to tie the variable to the actual label.
Tests:
-
Modify
PersonCardHandle
so that future tests can read the contents of the remark label.
[Step 4] Model: Add Remark
class
We have to properly encapsulate the remark in our ReadOnlyPerson
class. Instead of just using a String
, let’s follow the conventional class structure that the codebase already uses by adding a Remark
class.
Main:
-
Add
Remark
to model component (you can copy fromAddress
, remove the regex and change the names accordingly). -
Modify
RemarkCommand
to now take in aRemark
instead of aString
.
Tests:
-
Add test for
Remark
, to test theRemark#equals()
method.
[Step 5] Model: Modify ReadOnlyPerson
to support a Remark
field
Now we have the Remark
class, we need to actually use it inside ReadOnlyPerson
.
Main:
-
Add three methods
setRemark(Remark)
,getRemark()
andremarkProperty()
. Be sure to implement these newly created methods inPerson
, which implements theReadOnlyPerson
interface. -
You may assume that the user will not be able to use the
add
andedit
commands to modify the remarks field (i.e. the person will be created without a remark). -
Modify
SampleDataUtil
to add remarks for the sample data (delete youraddressBook.xml
so that the application will load the sample data when you launch it.)
[Step 6] Storage: Add Remark
field to XmlAdaptedPerson
class
We now have Remark
s for Person
s, but they will be gone when we exit the application. Let’s modify XmlAdaptedPerson
to include a Remark
field so that it will be saved.
Main:
-
Add a new Xml field for
Remark
. -
Be sure to modify the logic of the constructor and
toModelType()
, which handles the conversion to/fromReadOnlyPerson
.
Tests:
-
Fix
validAddressBook.xml
such that the XML tests will not fail due to a missing<remark>
element.
[Step 7] Ui: Connect Remark
field to PersonCard
Our remark label in PersonCard
is still a placeholder. Let’s bring it to life by binding it with the actual remark
field.
Main:
-
Modify
PersonCard#bindListeners()
to add the binding forremark
.
Tests:
-
Modify
GuiTestAssert#assertCardDisplaysPerson(…)
so that it will compare the remark label. -
In
PersonCardTest
, callpersonWithTags.setRemark(ALICE.getRemark())
to test that changes in thePerson
's remark correctly updates the correspondingPersonCard
.
[Step 8] Logic: Implement RemarkCommand#execute()
logic
We now have everything set up… but we still can’t modify the remarks. Let’s finish it up by adding in actual logic for our remark
command.
Main:
-
Replace the logic in
RemarkCommand#execute()
(that currently just throws anException
), with the actual logic to modify the remarks of a person.
Tests:
-
Update
RemarkCommandTest
to test that theexecute()
logic works.
A.2.3. Full Solution
See this PR for the step-by-step solution.
Appendix B: User Stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
|
new user |
see usage instructions |
refer to instructions when I forget how to use the App |
|
user |
add a new person |
|
|
user |
delete a person |
remove entries that I no longer need |
|
user |
find a person by name |
locate details of persons without having to go through the entire list |
|
user |
find a person by tags |
locate people easily |
|
user |
get confirmation before I edit or delete contacts |
[Coming in v2.0] |
|
user |
undo my actions |
fix my mistakes |
|
user |
add multiple numbers to a contact |
separate their mobile and home numbers |
|
teacher |
sort students by name and classes |
find students easily |
|
busy teacher |
be able to see who are the underperforming students |
find out who I should spend more time on |
|
teacher |
have contact information of students and parents |
contact either of them |
|
concerned teacher |
display statistics for different group of students with the same tags |
analyse and track their academic progress easily |
|
precise teacher |
find out the 25th, 50th and 75th percentile scores for each class |
get a better idea of students' academic progress |
|
precise teacher |
compare the grades statistics between any two groups of students |
make a quick comparison [Coming in v2.0] |
|
teacher |
schedule my timetable according to my classes |
plan my timetable to fit other activities |
|
caring teacher |
schedule appointments with my students |
conduct extra classes when they ask for it |
|
user |
have different colours of the tags for different tag names |
can differentiate different groups easily |
|
forgetful user |
see a history of my last used commands |
|
|
expert user |
have shorthand commands |
so that I can search for contacts faster |
|
user |
redo an action |
redo an undone action |
|
user |
have a welcome page |
feel motivated from wallpaper and quotes |
|
user |
hide private contact details by default |
minimize chance of someone else seeing them by accident [Coming in v2.0] |
|
first time user |
prompt user to input name |
personalise the addressBook [Coming in v2.0] |
|
user |
back up my address book data online |
I can access my address book data from other places [Coming in v2.0] |
|
user with many friends |
autoComplete my searches |
[Coming in v2.0] |
|
user |
share my friend’s contact with others |
[Coming in v2.0] |
|
user |
delete all people with a tag |
clean up addressBook faster [Coming in v2.0] |
|
lazy user |
addressBook to auto delete contacts after a certain date |
[Coming in v2.0] |
|
expert user |
have separate address books |
can separate my work and personal contacts [Coming in v2.0] |
|
user |
a calendar to sync my events |
can plan them more efficiently [Coming in v2.0] |
|
user |
see most recently accessed contacts |
so that I can access them faster [Coming in v2.0] |
|
busy user |
see a list of frequently accessed contacts |
so that I locate them faster [Coming in v2.0] |
|
user |
password protect my addressBook |
protect my contacts' information [Coming in v2.0] |
|
caring teacher |
help students find suitable study buddies |
motivate and aid them in their academic progress [Coming in v2.0] |
|
caring teacher |
be able to see my students' home location in extended screen using google maps |
conduct house visits easily if they fall sick [Coming in v2.0] |
|
busy teacher |
auto tag students according to their grades |
get a quick overview of their progress [Coming in v2.0] |
|
user |
add profile pictures to contacts |
find my friends easily [Coming in v2.0] |
|
user |
use facial recognition |
enhance security [Coming in v2.0] |
|
user |
call/message directly from my address book |
reach them easily [Coming in v2.0] |
|
thoughtful teacher |
remind students about homework assignments |
[Coming in v2.0] |
{More to be added}
Appendix C: Use Cases
(For all use cases below, the System is the AddressBook
and the Actor is the user
, unless specified otherwise)
Use case: Delete person
MSS
-
User requests to list persons
-
AddressBook shows a list of persons
-
User requests to delete a specific person in the list
-
AddressBook deletes the person
Use case ends.
Extensions
-
2a. The list is empty
Use case ends.
-
3a. The given index is invalid
-
3a1. AddressBook shows an error message
Use case resumes at step 2.
-
Use case: Add a person
MSS
-
User requests to add person
-
User inputs person details
-
AddressBook adds the person
Use case ends.
EXTENSIONS
-
2a. The given input is invalid (Wrong format, etc)
-
2a1. AddressBook shows an error message
-
-
3a. The same person details exists in AddressBook
-
3a1. AddressBook shows an error message.
Use case resumes at step 2.
-
Use case: List all persons
MSS
-
User requests to list persons
-
AddressBook shows a list of persons
Use case ends.
Extensions
-
2a. The list is empty
Use case ends.
Use case: Edit a person
MSS
-
User requests to list persons
-
AddressBook shows a list of persons
-
User requests to edit a person in the list
-
User inputs person details
-
AddressBook edit the person
Use case ends.
EXTENSIONS
-
2a. The given input is invalid (Wrong format, etc)
-
2a1. Addressbook shows an error message for such situation
Use case ends.
-
-
3a. The list contains no matching person to edit
-
3a1. Addressbook shows an error message for such situation
Use case ends.
-
Use case: Find person
MSS
-
User requests to find person
-
AddressBook lists the person found
Use case ends.
Extensions
-
2a. Addressbook does not contain the person requested
-
2a1. AddressBook displays an empty list
-
Use case ends.
-
3a. The given input or command is invalid (Wrong format, etc)
-
3a1. AddressBook shows an error message
Use case resumes at step 1.
-
Use case: Select person
MSS
-
User requests to list persons
-
AddressBook shows a list of persons
-
User requests to select a person in the list
-
AddressBook selects the person and displays the Google search page for the person
Use case ends.
Extensions
-
2a. Addressbook does not contain any person
-
2a1. AddressBook displays an empty list
-
Use case ends.
-
3a. The given input or command is invalid (Wrong format, etc)
-
3a1. AddressBook shows an error message
Use case resumes at step 1.
-
Use case: Display History
MSS
-
User requests to list history of commands
-
AddressBook shows a list of previous commands arranged from most recent to earliest
Use case ends.
Extensions
-
2a. Addressbook does not contain any history of commands
-
2a1. AddressBook displays an empty list
-
Use case ends.
Use case: Sort
MSS
-
User requests to sort persons in alphabetical order
-
AddressBook sorts the list of persons
-
AddressBook displays the sorted list
Use case ends.
Extensions
-
2a. The list is empty
Use case ends.
Use case: Add Schedule
MSS
-
User requests to add schedule with a given person index, time and date
-
AddressBook adds the schedule to the schedule list
-
AddressBook displays the result of user’s request
Use case ends.
Extensions
-
2a. Addressbook does not contain any person
-
2a1. AddressBook shows an error message
-
Use case ends.
-
3a. The given input or command is invalid (Wrong format, etc)
-
3a1. AddressBook shows an error message
Use case resumes at step 1.
-
Use case: Undo
MSS
-
User requests to undo previous command
-
AddressBook search for previous commands
-
AddressBook undo the previous command
Use case ends.
Extensions
-
2a. The user undo without making any previous commands
-
2a1. AddressBook shows an error message Use case ends.
-
-
3a. The previous command cannot be done
Use case resumes at step 1.
Use case: Redo
MSS
-
User requests to redo most recent undo command
-
AddressBook search for previous undo command
-
AddressBook redo the previous undo command
Use case ends.
Extensions
-
2a. The user redo without making any previous undo commands
-
2a1. AddressBook shows an error message Use case ends.
-
Use case: Clear
MSS
-
User requests to clear all entries from AddressBook
-
AddressBook clears all entries
Use case ends.
{More to be added}
Appendix D: Non Functional Requirements
-
Should work on any mainstream OS as long as it has Java
1.8.0_60
or higher installed. -
Should be able to hold up to 1000 persons without a noticeable sluggishness in performance for typical usage.
-
A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
-
Should work in systems with at least 20MB of storage space.
-
Should work in systems with internet connection.
-
A user with sufficient proficiency in English to understand addressbook commands and instructions.
-
Should be able to hold up to 100 tags per person.
-
The system should respond within five seconds.
-
The system should be able to store at least 1000 histories of a user.
-
The system should be able to store at least 1000 contacts.
{More to be added}
Appendix E: Glossary
Mainstream OS
Windows, Linux, Unix, OS-X
Private contact detail
A contact detail that is not meant to be shared with others
API
Application programming interface (API) is a set of subroutine definitions, protocols, and tools for building application software.
JUnit
JUnit is a simple framework to write repeatable tests. It is an instance of the xUnit architecture for unit testing frameworks.
Gradle
Gradle is an open source build automation system that builds upon the concepts of Apache Ant and Apache Maven and introduces a Groovy-based domain-specific language (DSL).
Shorthand Commands
Shortcut keys that help to provide an easier and usually quicker method of navigating and executing commands in computer software programs.
Travis_CI
Travis_CI is a hosted, distributed continuous integration service used to build and test software projects hosted at GitHub.
Appendix F: Product Survey
Product Name
Pros | Cons |
---|---|
Easy to store data of contacts |
Need to enter data in manually |
Can edit details of contacts easily using Command Line Interface(CLI) |
No option for Graphical User Interface(GUI) editing |
Pros | Cons |
---|---|
Easy to use |
|
Interface design is clean and sleek |
Cannot customise the design to my needs/favourite colour |
Sort function is intuitive and fast - sorts my contacts in an instant |
Does not allow automatic sorting once contacts are stored |
Pros | Cons |
---|---|
Colour of interface is great |
Cannot personalise to fit my mood |
Easy to manage a contact list |
Does not allow adding in other fields that I need in the addressbook |
Contact list on starter page is shown clearly - all contacts can be seen with the scroller |
Details of person in contact list cannot be edited according to my needs |