Project: cherBook
cherBook - is a desktop address book application used for teachers and educators. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java.
Code contributed: [Functional code] [Test code]
Enhancement Added: Sort Command [v1.2]
Sorting all persons : sort
Since v1.2
Helps you sort all persons in cherBook.
Shows you a list of all persons in cherBook with their names sorted in alphabetical order.
If you want your list to be sorted at all times, you can use this sort command after adding contacts into cherBook,
After that, your contact list would always be sorted when you perform other commands on cherBook.
Format: sort
Shorthand: st
Examples:
-
list
sort
Sorts the list in alphabetical order. -
find Betsy
st
Sorts the list in alphabetical order.
Example:
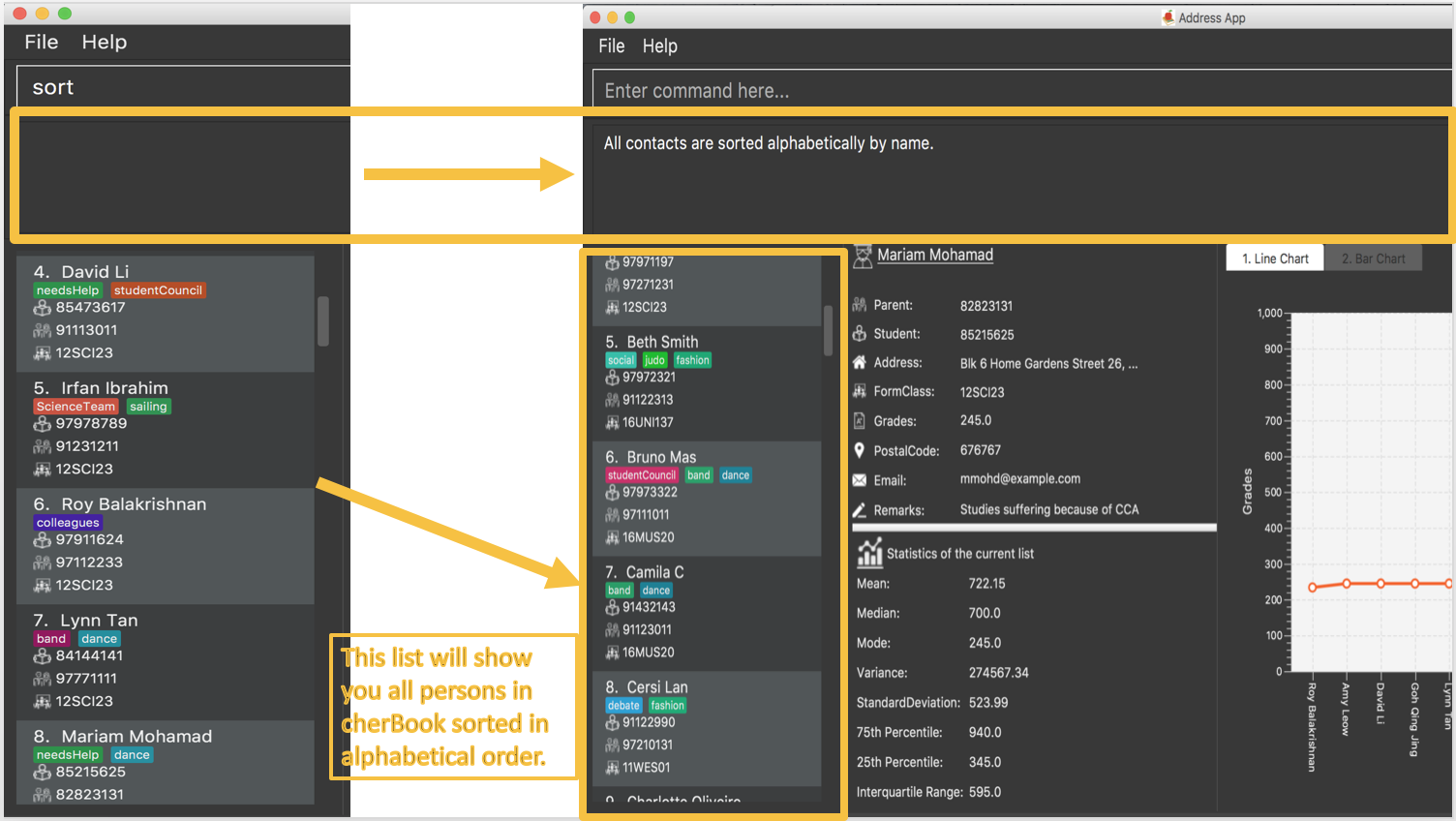
Figure 5.1.9.1 : Sorts and displays all your contacts in cherBook
End of Extract
Justification
Start of Extract [from: Developer Guide]
The reason for the implementation of sort allows the user to organise their list of contacts in cherBook more easily.
This provides an alternative for them to view their contacts instead of the usual way - most recent added contact being added to the last index of the list.
Users will find it easier to browse through their list of contacts in situations where the find
command cannot be used.
The sort
mechanism is implemented using SortCommand
. It supports the sorting of contact names in cherBook according to alphabetical order from A to Z.
End of Extract
Implementation
Start of Extract [from: Developer Guide]
When the user calls for sorting to be implemented, an empty ArrayList
would be created. Under the Model
component, UniquePersonList
would compare the names of all persons in lower case using a comparator and return an Observable List
containing of ReadOnlyPerson
s.
SortCommand
inherits from Command
.
SortCommand
is implemented this way:
public class SortCommand extends Command {
@Override
public CommandResult execute() {
// ... sort logic ...
}
}
The sort is case insensitive.
|
The following sequence diagram shows how the sort
operation works:
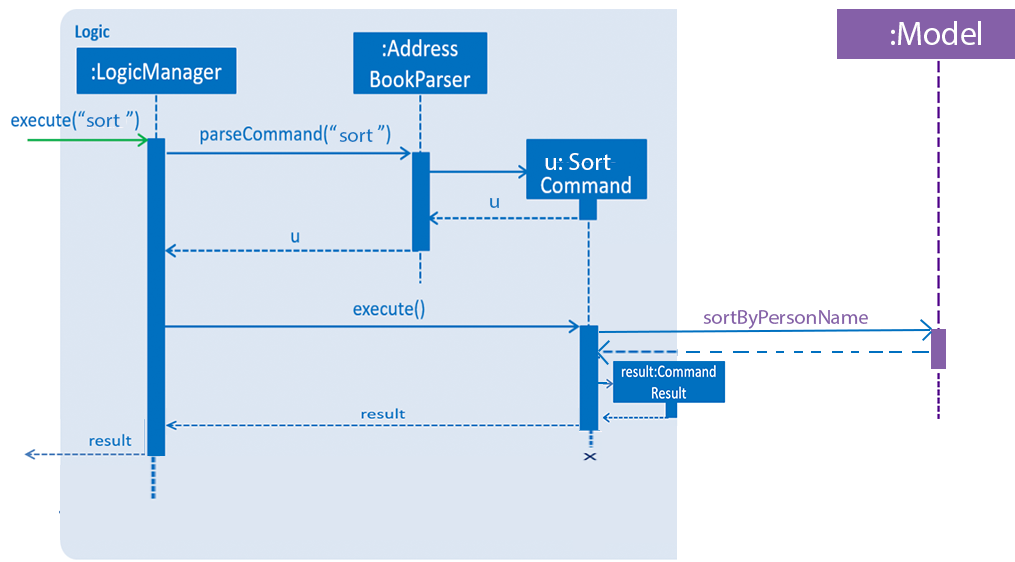
Figure 4.6.1 : Sequence Diagram of Sort Operation
When the user calls ‘sort’ or ‘st’ to sort
the contacts, the Model
will take in an ArrayList
named contactList from the Parser
.
Upon a successful sort
, all contacts in cherBook are displayed in alphabetical order.
If an empty list is given, the address book will return with the message: “No contacts to be sorted”.
If the list is already in the correct alphabetical order, the address book will return with the following message: “All contacts are sorted alphabetically by name”.
The following code will show how the list is being returned to the user:
/**
* Returns an observable list as as an unmodifiable {@code ObservableList}
*/
public ObservableList<ReadOnlyPerson> asObservableListSortedByName() {
internalList.sort((o1, o2) ->((o1.getName().toString().toLowerCase())
.compareTo(o2.getName().toString().toLowerCase())));
return FXCollections.unmodifiableObservableList(mappedList);
}
The sort mechanism is implemented this way to ensure that all person names in the UniquePersonList
is compared to at the lowest level of abstraction.
Design Considerations
Aspect: Implementation of SortCommand
Alternative 1 (current choice): SortCommand
inherits from Command.
Pros: After sorting his/her contacts, it remains sorted and the original list of unsorted contacts is replaced by this list.
Cons: The user is unable to save the list of contacts in the original order(unsorted format).
Alternative 2: Implement undo/redo function for SortCommand.
Pros: When undo is called, the list will return back to the most recent unsorted list. When redo is called, the list will return back to the sorted list of contacts.
Cons: The user is able to alter the list easily and may get confused whether is the current list the most recent sorted list.
Aspect: How sort executes
Alternative 1 (current choice): Uses the comparator
in Java Collections
to sort the arrays in alphabetical order.
Pros: Easy to implement.
Cons: Since method is inherited, it is difficult to debug and the only way is via the usage of vigorous user testing and logging.
Alternative 2: Write a sort
algorithm.
Pros: Case sensitivity can be accounted for and debugging is made simpler.
Cons: More error-proned as algorithm’s logic must be checked constantly to ensure that it accounts for all cases.
Aspect: Data structure to support the sort
command
Alternative 1 (current choice): Creates an empty ArrayList
to obtain the names from the UniquePersonList
before sorting occurs. The sort
overwrites the existing list of unsorted contacts.
Pros: The original list of unsorted contacts is not altered.
Cons: Additional space usage and longer time needed to overwrite the original contact list, inefficiency and memory wastage may arise.
Alternative 2: Creates a new list of persons to sort each time a command that filters the person list is entered.
Pros: User can sort accordingly to the filtered person list that he/she has implemented. Able to revert any changes if user does not want their contact list to be sorted.
Cons: User have to continously use the sort command for every filtered person list change. Inefficient if the user wants their contact list to be sorted at all times.
End of Extract
Enhancement Added: Extended Person Card Panel [v1.3]
External behavior
Start of Extract [from: User Guide]
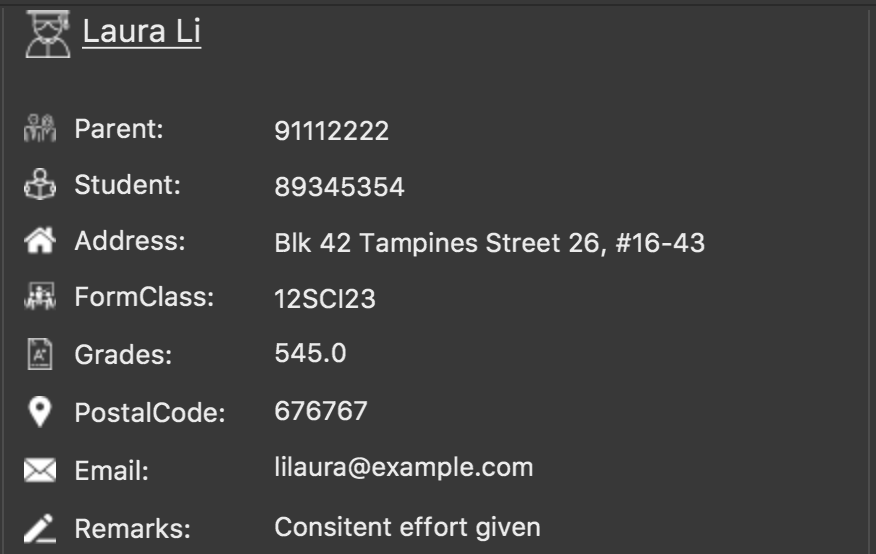
This figure shows how the Extended Person Card looks like in cherBook. It contains all the information of a student.
End of Extract
Student Details Panel Since v1.3
cherBook uses the Student Details Panel
to display details belonging to the currently selected student.
The details changes automatically where there is a change to the list of students in the Students Panel
.
You can use these details to pick out relevant information you need from your student.
End of Extract
Enhancement Added: Schedule Command [v1.4]
Adding a schedule : schedule
Since v1.4
Schedules the person identified by the index number used in the last person listing.
Format: addschedule INDEX s/[DATE]
Shorthand: addsch INDEX s/[DATE]
Examples:
-
list
addschedule 2 s/tomorrow 7pm
Schedules the 2nd person in cherBook for tomorrow at 7pm and adds the schedule to the schedule list. -
find Betsy
addsch 1 s/25 december 2017 3pm
Schedules the 1st person in cherBook on 25 December at 3pm and adds the schedule to the schedule list in the results of thefind
command.
Example:
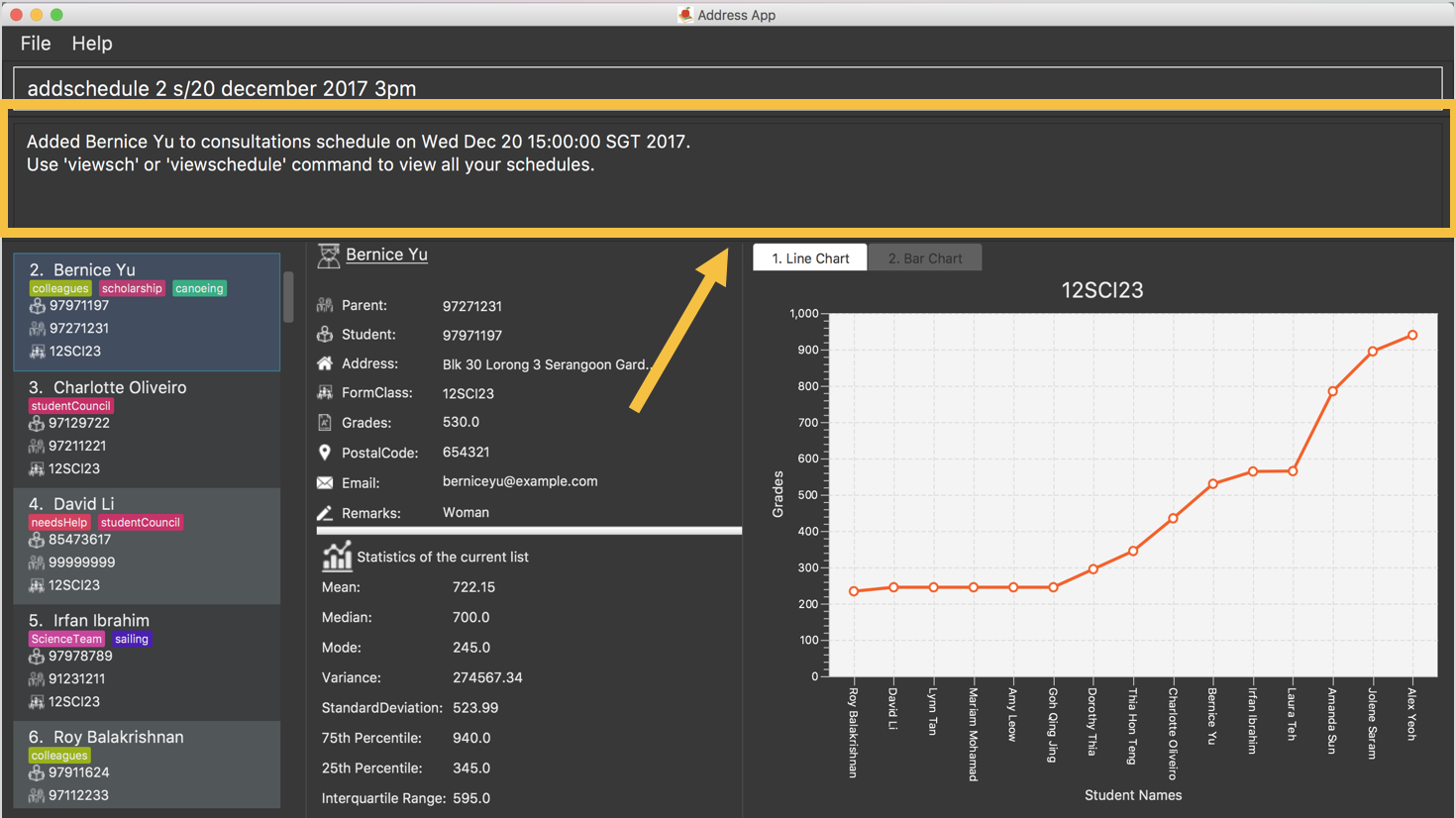
Figure 5.2.1.1 : Adds a schedule to your cherBook
End of Extract
Justification
Start of Extract [from: Developer Guide]
The schedule
mechanism is implemented using ScheduleCommand
. It supports the scheduling of contacts in cherBook for better management of time.
The reason for the implementation of scheduling is to allow cherBook users (teacher/educator) to add schedules they have like consultations or meetings with their students or fellow colleagues.
With this scheduling command, users are able add their schedules with any person from their list of contacts just by typing the index of the person, the date and time of the schedule.
The date and time can be entered in natural language, helping users save their time to manually pen down their scheduled dates and timings.
End of Extract
Implementation
Start of Extract [from: Developer Guide]
When the user calls for scheduling to be implemented, two arguments would have to be passed into the constructor.
The two arguments are Name of the person being scheduled and the Calendar date with time.
Under the Model
component, the created schedule
is directly added into the address book.
ScheduleCommand
inherits from Command
.
ScheduleCommand is implemented this way:
public class ScheduleCommand extends Command {
@Override
public CommandResult execute() {
// ... schedule logic ...
}
}
Adding of schedules into the AddressBook can be done in natural language.
|
The following sequence diagram shows how the schedule
operation works:
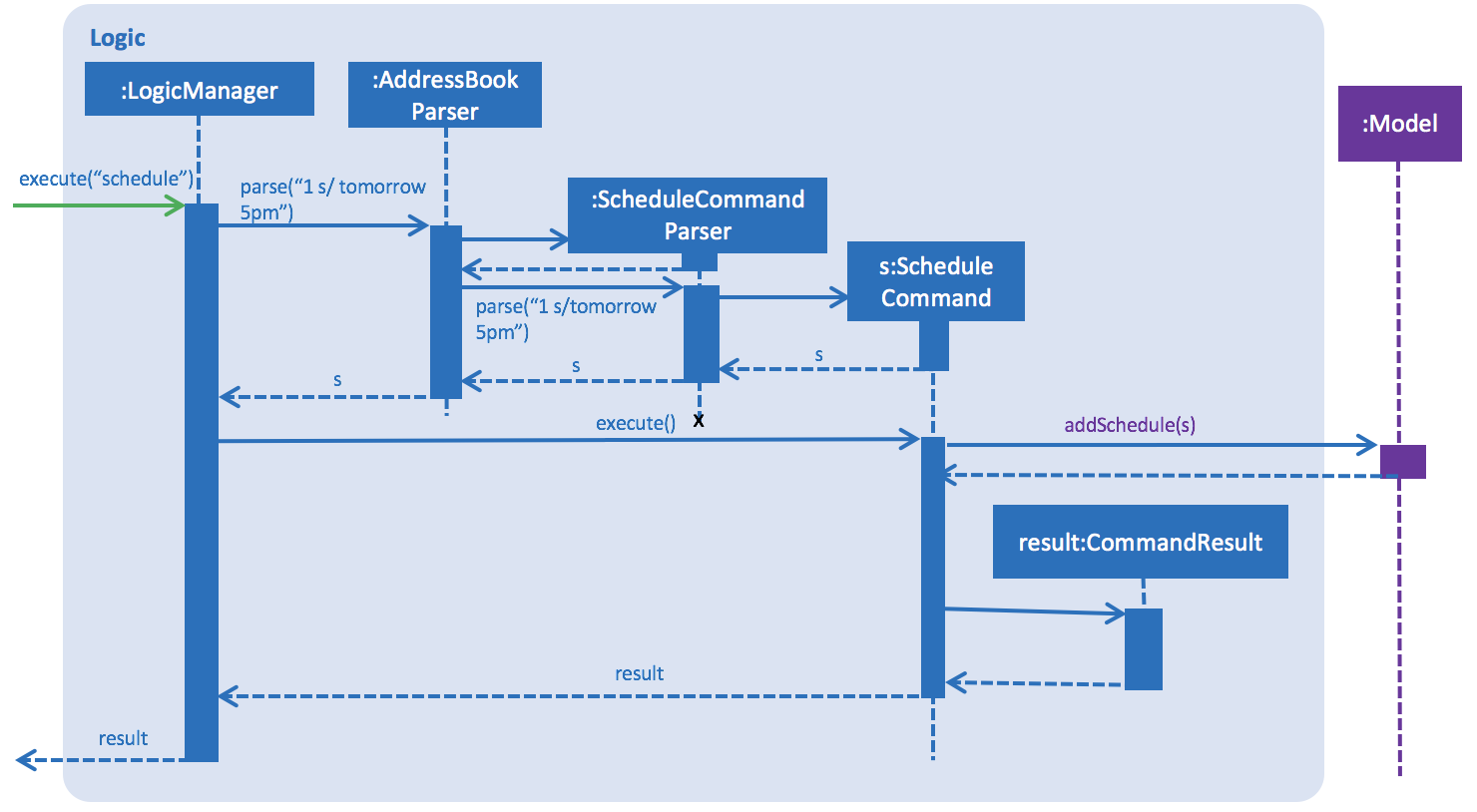
Figure 4.7.1 : Sequence Diagram of Schedule Operation
When the user calls ‘schedule’ or ‘sch’ to schedule the contacts in cherBook, the Model will receive a schedule from the Parser.
Upon successful scheduling, contacts are added into the set of schedules
.
If one or more arguments required to schedule a person is missing, the AddressBook will return with error messages corresponding to the missing arguments.
If one or more arguments required to schedule a person is invalid, the AddressBook will return with error messages corresponding to the invalid arguments.
The following will show how the schedule
is added into the AddressBook:
/**
* Adds a schedule for a student's consultation
*/
@Override
public void addSchedule(Schedule schedule) {
addressBook.addSchedule(schedule);
addressBook.sortSchedules();
indicateAddressBookChanged();
indicateScheduleListChanged();
}
It is implemented this way to ensure that all schedules
are added into the AddressBook with the lowest level of abstraction.
Design Considerations
Aspect: Implementation of ScheduleCommand
Alternative 1 (current choice): ScheduleCommand
inherits from Command.
Pros: After scheduling the contacts, the schedules remain in the order they are entered into the AddressBook.
Cons: The user is unable to sort the list of schedules according to chronological order.
Alternative 2: ScheduleCommand
inherits from Command.
Pros: After scheduling the contacts, the schedules remain in the order they are entered into the AddressBook.
Cons: The user is unable to sort the list of schedules according to chronological order.
Aspect: How schedule
executes
Alternative 1 (current choice): Adds a schedule
into the schedules list
once the person name and calendar date is parsed.
Pros: Easy to implement.
Cons: Vigorous user testing and logging is required to debug corner test cases because there is little control over imported APIs.
Aspect: Data structure to support the schedule
command
Alternative 1 (current choice): The list of schedules is altered directly from cherBook and is a standalone class.
Pros: Without association with any other classes, it is easy to implement and debug. Performance of program is fast because the list of schedules is retrieved directly from cherBook.
Cons: The user would not be able to view information of the schedules directly from each contact.
Alternative 2: Add each schedule into the contact as an attribute.
Pros: The user would be able to view the schedules directly from the respective contacts.
Cons: Performance of program would be affected, because to retrieve the whole list of schedules, the algorithm would have to iterate through all the contacts in cherBook.
End of Extract
Enhancement Added: View Schedule Command [v1.5]
Viewing my schedule : viewschedule
Since v1.5
View your full schedule list in cherBook.
Format: viewschedules
Shorthand: viewsch
Examples:
-
list
viewschedules
Displays all your schedules in the command box. -
find Betsy
viewsch
Displays all your schedules in the command box.
Example:
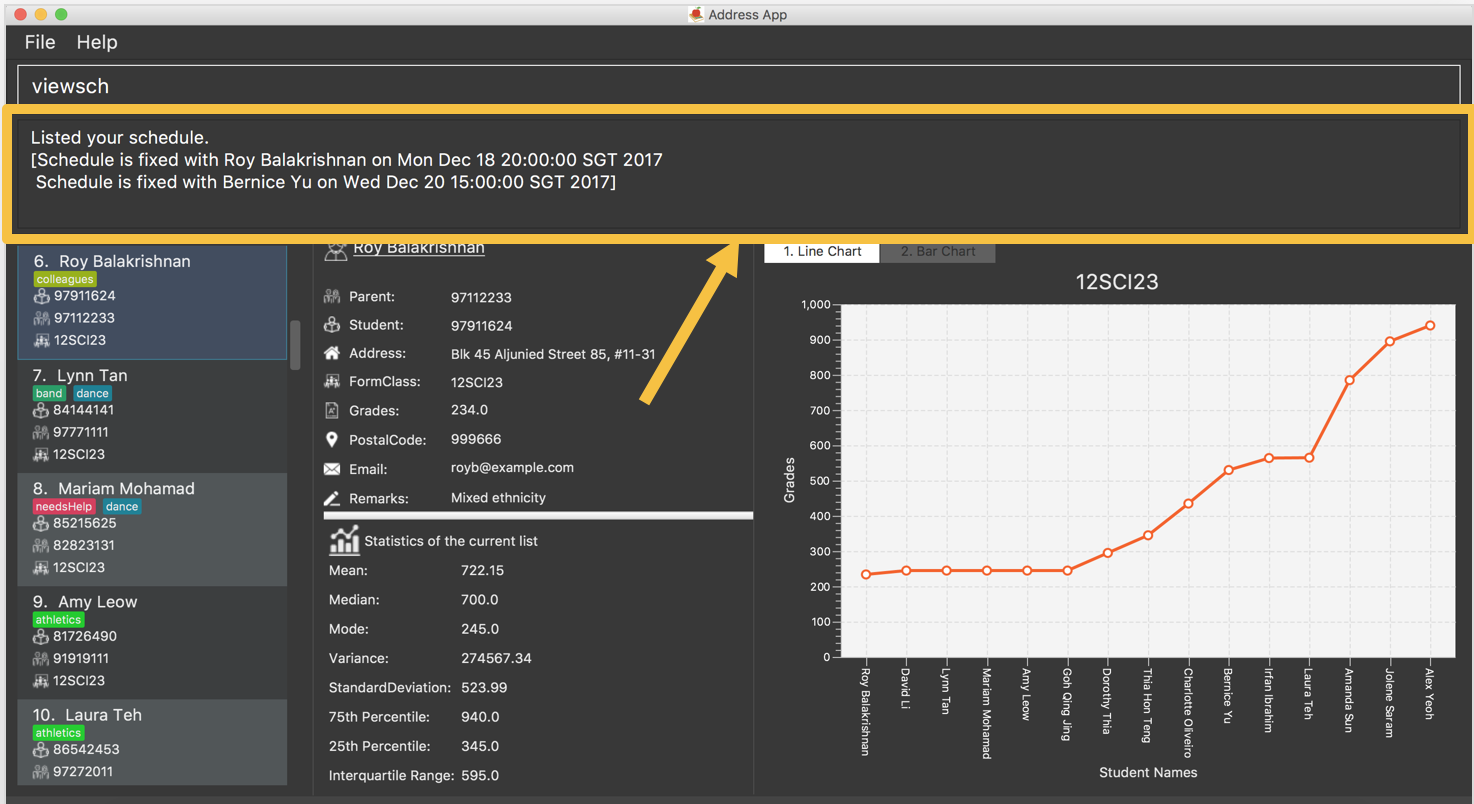
Figure 5.2.2.1 : View all your schedules in cherBook as shown in the box
End of Extract
Justification
Start of Extract [from: Developer Guide]
The viewschedule
mechanism is implemented using ViewScheduleCommand
. It displays the schedules of the user of cherBook.
The reason for the implementation of viewing schedules is to allow cherBook users (teacher/educator) to keep track of their schedules as they often have many consultations or meetings with their students or fellow colleagues.
With this view schedule command, users are able to view all their schedules at once, sorted by date and time.
This way, the user is able to maintain their timetable easily.
End of Extract
Implementation
Start of Extract [from: Developer Guide]
When the user calls to view all the schedules, the 'Model'
component would return with the set of schedules that was previously added into cherBook.
ViewScheduleCommand is implemented this way:
public class ViewScheduleCommand extends Command {
@Override
public CommandResult execute() {
// ... view schedule logic ...
}
}
The schedules would be listed in the ResultDisplay
panel, and each schedule would be separated with a new line.
Aspect: Data structure to support the ViewSchedule
command
Alternative 1 (current choice): The list of schedules is obtained directly from the Model
before it is being displayed on the ResultDisplay
panel.
Pros: It is easy to implement and debug. Performance of program is fast because the list of schedules is retrieved directly from the Model
.
Cons: The user do not have the alternative to view information of the schedules from each individual contact.
End of Extract
Enhancement Added: Delete Schedule Command [v1.5]
Deleting a schedule : deleteschedule
Since v1.5
Deletes the specified schedule from cherBook.
Format: deleteschedule INDEX
Shorthand: deletesch INDEX
Examples:
-
viewschedule
deleteschedule 2
Deletes the 2nd schedule in the results of theviewschedule
command which returns a list of schedules. -
viewsch
deletesch 1
Deletes the 1st schedule in the results of theviewsch
command which returns a list of schedules.
Example:
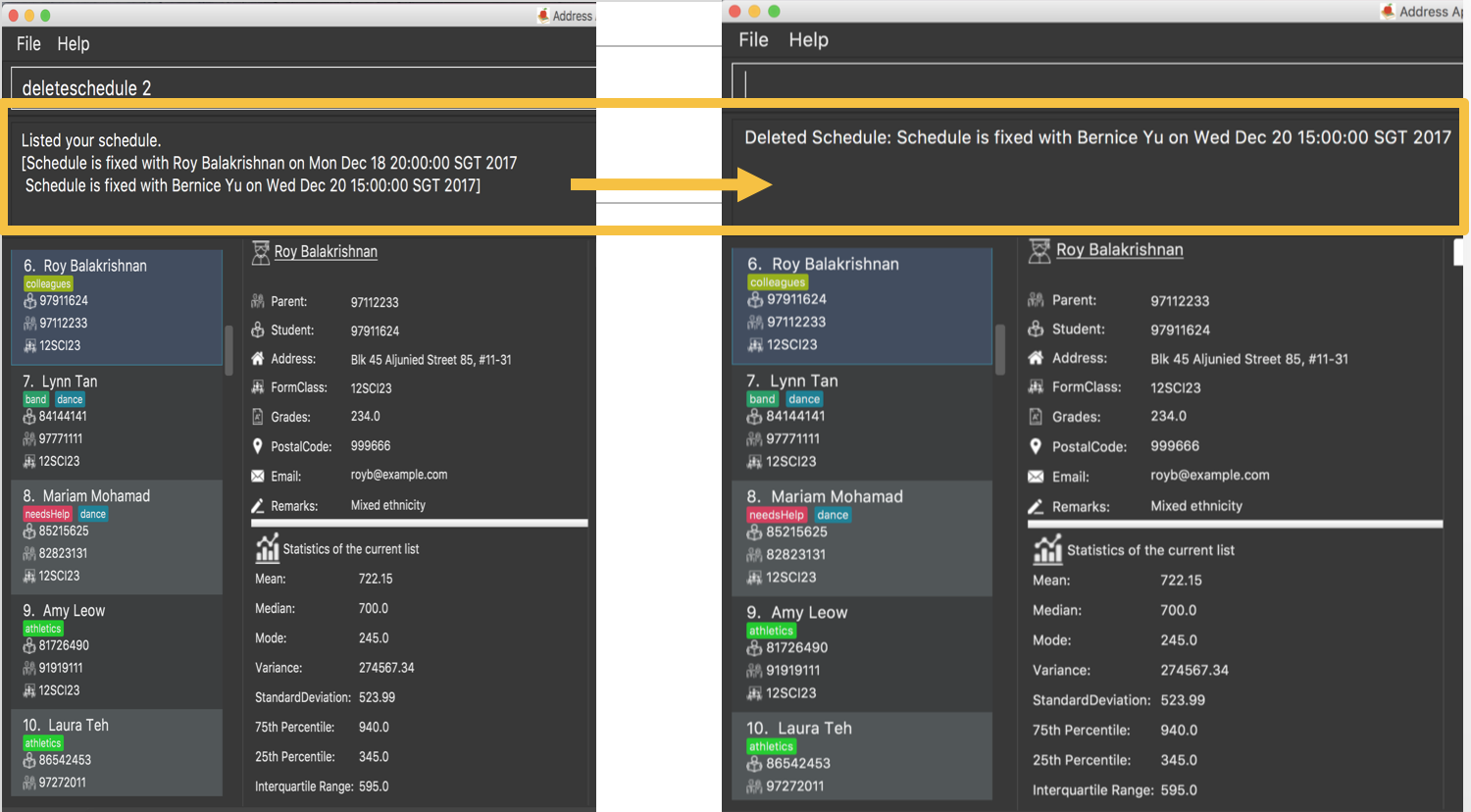
Figure 5.2.3.1 : Deletes the schedule in your cherBook with the specified index
End of Extract
Enhancement Proposed: Use Postal Code
to display Google Map of student’s address
External behavior
Clicking the Postal Code
attribute displayed on the Student Details
panel would open a Google Map
panel that displays the map of the student’s address. The map would display the surroundings as well as provide all possible ways to get to the student’s house.
Justification
Sometimes when students fall sick for a long period of time, they might face huge difficulties in trying to catch up with their studies. The reason for this implementation is so that users of cherBook (teachers / educators) have the ability to conduct house visits easily to update their student on the school-related assignments, as well as to show concern for the student.
Implementation
Link the Postal Code
attribute to Google Map
panel whereby the map would display the vicinity of the student’s address. Google
API would be called and the Postal Code
of the user’s current and designated location would be parsed before the map and routes are displayed and calculated respectively.
Enhancement Proposed: Use Schedules to remind users (teachers / educators) about their meetings and appointments
External behavior
No external behaviour. The teacher only has to enter their schedules into cherBook. cherBook will automatically remind them ten minutes before when their scheduled date and time is due.
Justification
When teachers have many schedules to keep and meetings to attend in one day, and not forgetting their regular classes and duties, it is often hard for them to keep track of time.
Our cherBook application can make use of the schedules that the teacher has added and remind them ten minutes before any schedules are due. This is so that the teachers can focus on their work and let cherBook keep time for them instead.
Implementation
The clock function in cherBook is required to take note of the time and compare it with the date and time of every schedule in the schedule list.
cherBook will produce a audible reminder ten minutes before every scheduled appointment.
Other contributions
-
Update READMEs for the different versions, created Ui mockups and cherBook icon