Project: cherBook
cherBook is an address book application used by teachers to monitor students' academic progress. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 30 kLoC.
Enhancement Added: Remark
Overwrites a remark to a person : remark
Since v1.2
Overwrites a remark from the specified person in cherBook
Format: remark Index [rm/REMARK]
Shorthand: rm Index [rm/REMARK]
Addition of remarks on a person already with remarks will be overwritten. Person with no remarks will display (add a new remark) .Can only be changed through the use of the remark command.
|
Examples:
-
list
remark 2 rm/This is a remark
Overwrites the 1st person remark. -
find betsy
remark 1 rm/Betsy is a female
Overwrites the 1st person in the results offind
command. -
list
remark 2 rm/
Overwritesremark
from the 2nd person in the address book and displays it as(add a new remark)
.
Example:
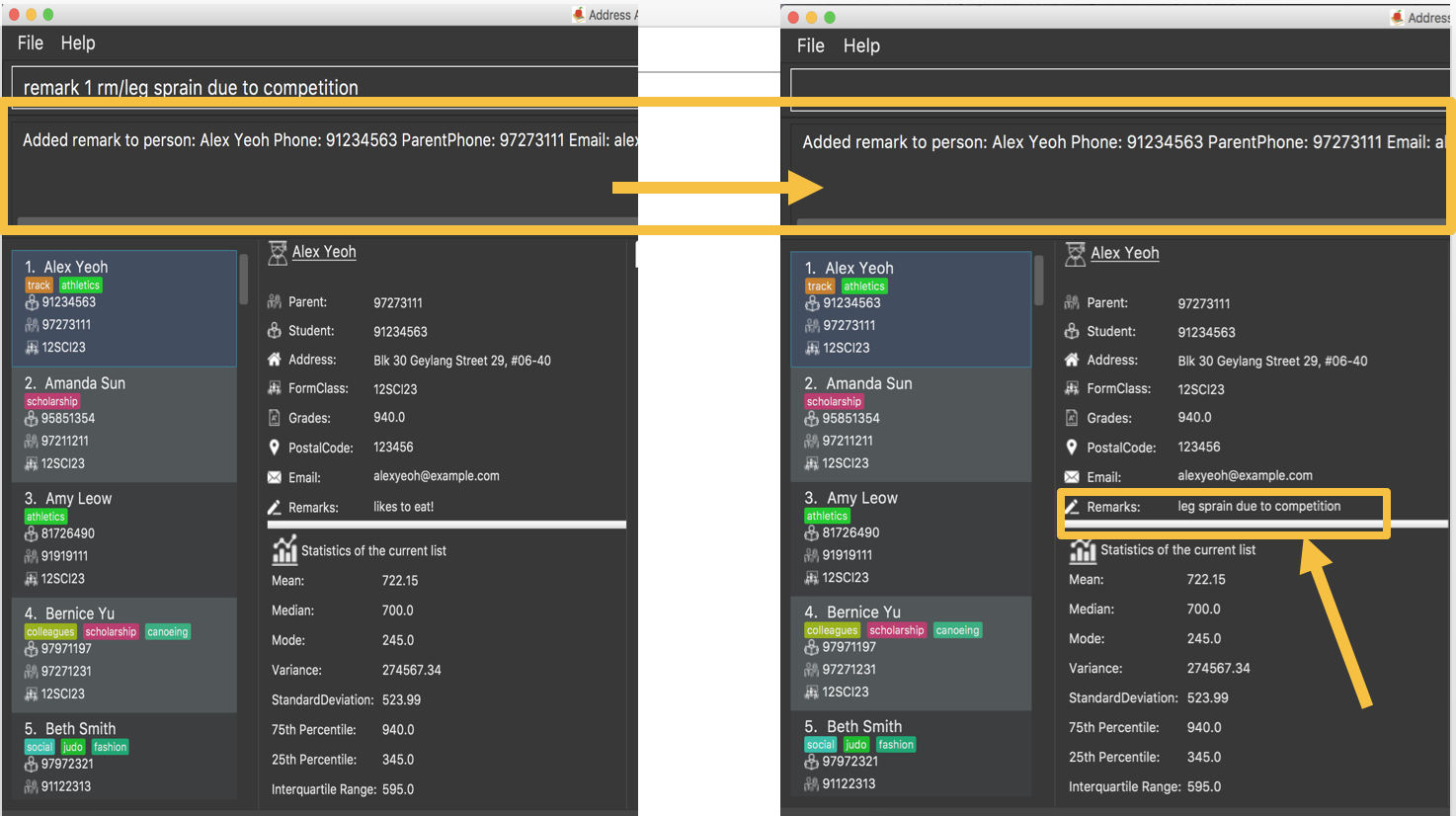
Figure 5.1.4.1 : Add a remark to your contacts in cherBook
End of Extract
Justification
We added remark such that the user can record the progress of students that can be easily known at a glance.
Remark mechanism
The remark
mechanism is facilitated by making changes to the Remark
class in the Model
component. It enables the user to overwrite a single remark
of the student.
To avoid confusion and an overly complicated UI
, only one remark
per person is stored.
The following sequence diagram shows how the remark
operation works:
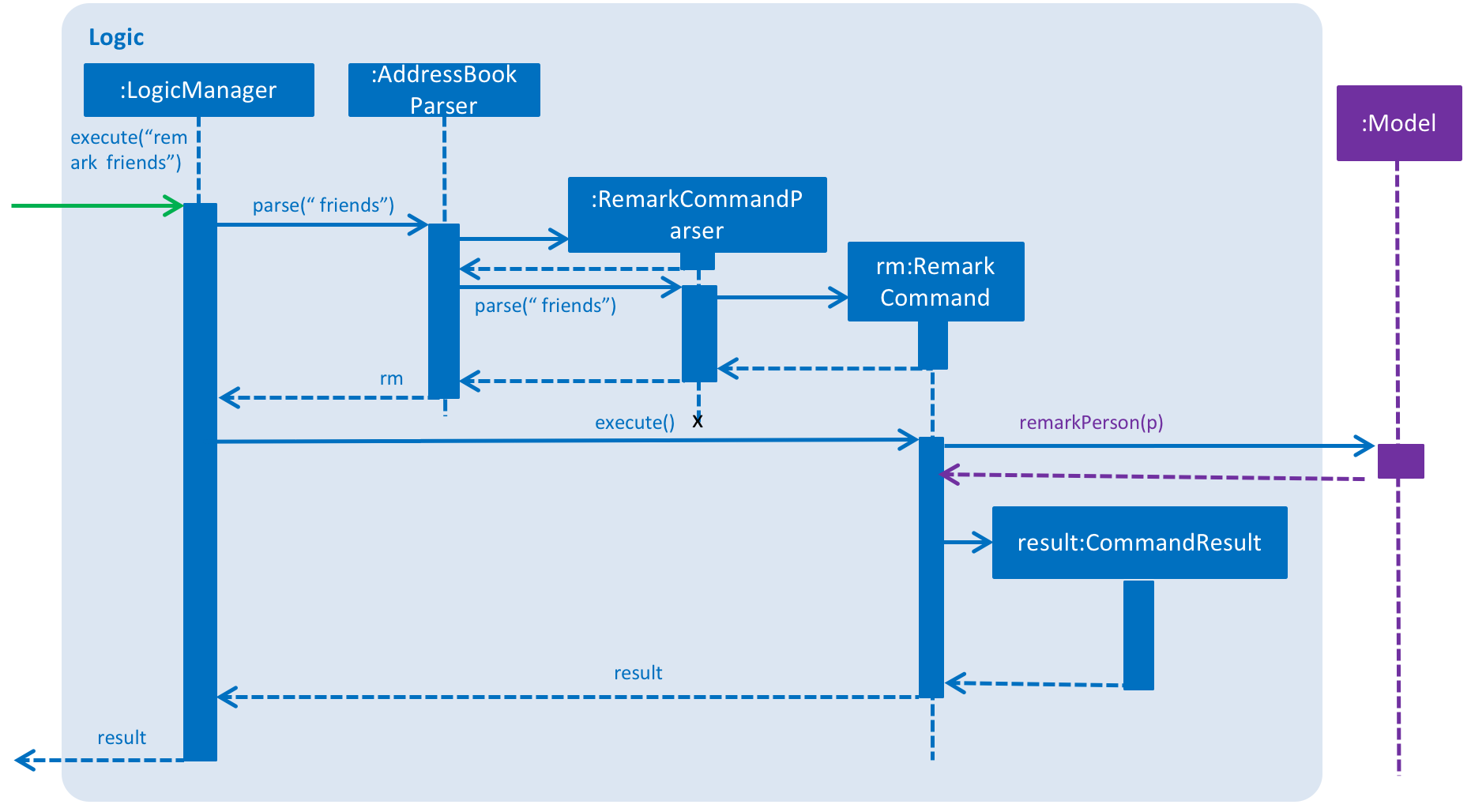
Figure 4.3.1 : Sequence Diagram of Remark
Operation
Remark
only deals with UndoableCommands
. Commands that cannot be undone will inherit from Command
instead. The following diagram shows the inheritance diagram for commands:
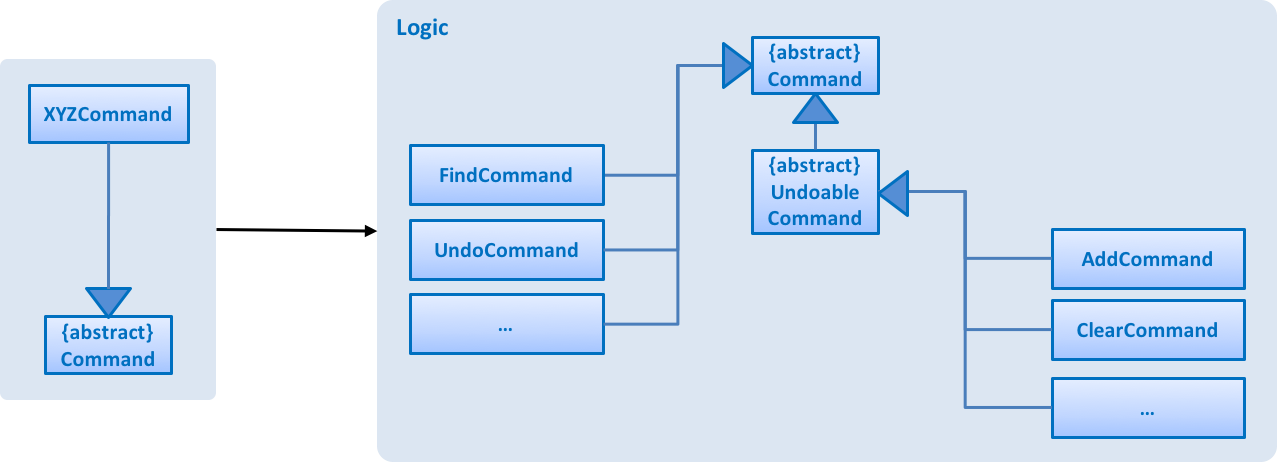
Figure 4.3.2 : Inheritance Diagram for Commands
As you can see from the diagram, UndoableCommand
adds an extra layer between the abstract Command
class and concrete commands that can be undone, such as the DeleteCommand
. Note that extra tasks need to be done when executing a command in an undoable way, such as saving the state of the address book before execution. UndoableCommand
contains the high-level algorithm for those extra tasks while the child classes implements the details of how to execute the specific command. Note that this technique of putting the high-level algorithm in the parent class and lower-level steps of the algorithm in child classes is also known as the template pattern.
Commands that are not undoable are implemented this way:
public class RemarkCommand extends Command {
@Override
public CommandResult execute() {
// ... remark logic ...
}
}
With the extra layer, the commands that are undoable are implemented this way:
public abstract class UndoableCommand extends Command {
@Override
public CommandResult execute() {
// ... undo logic ...
executeUndoableCommand();
}
}
public class DeleteCommand extends UndoableCommand {
@Override
public CommandResult executeUndoableCommand() {
// ... delete logic ...
}
}
When the user adds a new person
to the address book, that person’s remark
field will be denoted by (add a remark)
. Users are then able to add a single remark
.
If the user tries to add remark together with adding a new Person , it will display as invalid as the addCommand does not allow the addition of remarks other then through the use of using the Remark command.
|
When the user attempts to add /edit a remark via the add /edit command, he will be prompted by a message stating
that adding of remarks in add /edit command is not allowed.
|
Only one remark is saved at any one time, if the user does Remark on the same person, it will be overwritten.
|
Design Considerations
Aspect: Implementation of UndoableCommand
Alternative 1 (current choice): Add a new abstract method executeUndoableCommand()
.
Pros: We will not lose any undone/redone functionality as it is now part of the default behaviour. Classes that deal with Command
do not have to know that executeUndoableCommand()
exist.
Cons: Hard for new developers to understand the template pattern.
Alternative 2: Just override execute()
.
Pros: Does not involve the template pattern, easier for new developers to understand.
Cons: Classes that inherit from UndoableCommand
must remember to call super.execute()
, or lose the ability to undo/redo.
Aspect Implementation of Remark
field.
Alternative 1 (current choice): Add a field in person as well as making it a command.
Pros: Able to easily change context of the field without much hassle.
Cons: Harder to debug as it is both a field and a command.
Alternative 2: Making it a field only.
Pros: Easier to keep track and debug.
Cons: Much harder and longer to implement a feature to solely change the remarks.
End of Extract
Enhancement Added: GraphPanel
Graph Panel Since v1.3
cherBook uses a GraphPanel
to plot the grades of all classmates of the selected student on a graph.
The graphs are automatically sorted from the lowest grade to the highest.
There are two tabs for you to choose from, line or bar and can be switch by using the tab
command.
The details changes when another student from a different class is selected.
You can use these graph to better understand the trends of grades
in a glance to easily see who are the weaker or stronger students.
End of Extract
Justification
To display the results of all students in the same class to see the difference in grades that can be seen at a glance.
GraphPanel Mechanism
LineChart
mechanism is implemented using javaFX
. It takes in FilteredPersonList
and plots a graph based on those who are in the same formClass
.
Using XYChart.series
to store the data of the persons name and grade, a coordinate
is created and a line
is drawn connecting all the coordinates
.
These coordinates are sorted in ascending order so that the user is able to identify those in the lower quartile.
The LineChart
mechanism is implemented this way:
for (ReadOnlyPerson people : people) { if (people.getFormClass().equals(person.getFormClass())) { series.getData().add(new XYChart.Data<>(people.getName().toString(), Double.parseDouble(people.getGrades().toString()))); } } series.getData().sort(Comparator.comparingDouble(d -> d.getYValue())); //...other logic...
Design Considerations
Aspect: Graph to be displayed for an effective visual aid
Alternative 1 (current choice): Line chart to display the grades of student.
Pros: Easier to implement.
Cons: May not be the most appropriate way to display grades of students.
Alternative 2: Bell curve to display the grades of students.
Pros: A better representation of the student grades and it is easier to interpret.
Cons: Much harder to plot a graph based on statistics then raw data.
End of Extract
Enhancement Added: Tab
External behavior
Start of Extract [from: User Guide]
Switching between graphs: tab
Since v1.5
Switches between the line and bar chart in the GraphPanel
.
Format: tab TABNUMBER
Valid numbers are determined by number of tabs available. In this case, only 1 and 2 are valid, which displays line or bar respectively. Typing the same index on the selected graph would just do nothing. |
Example:
-
tab 2
Remains at tab 2 if tab 2 is selected else switches to tab 2.
Examples:
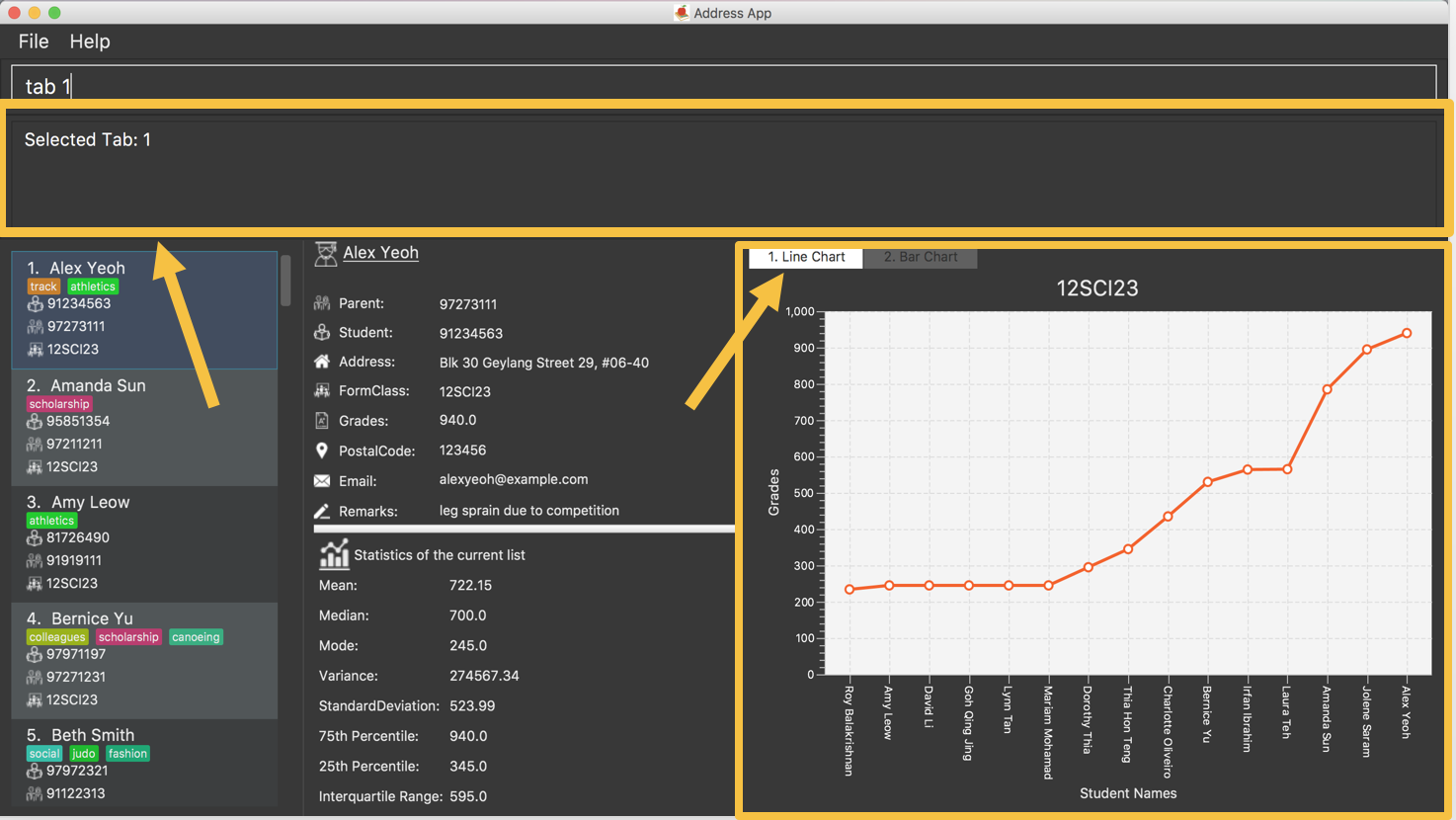
Figure 5.3.4.1 : Displays the graph in your cherBook with the first tab index
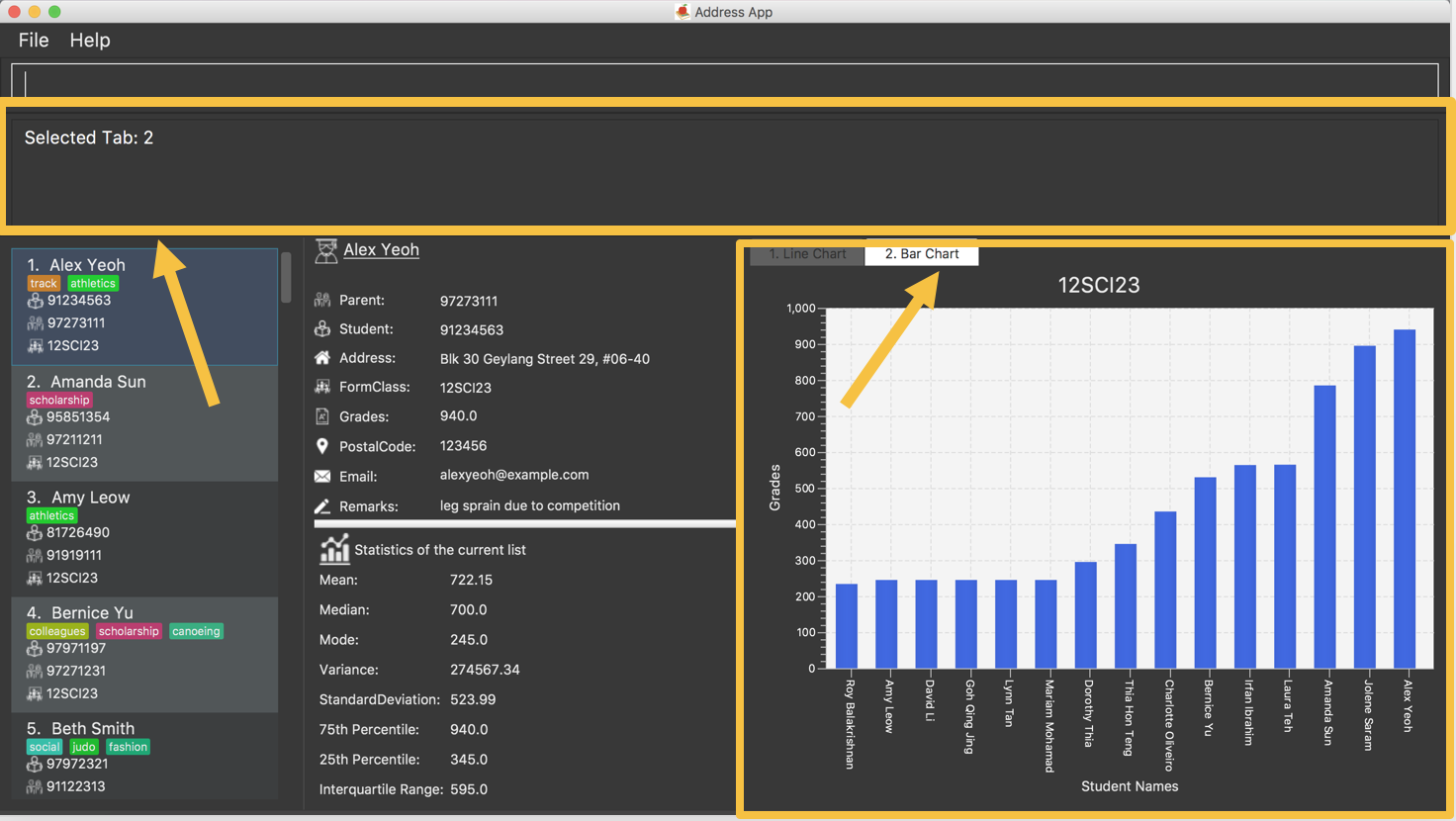
Figure 5.3.4.2 : Displays the graph in your cherBook with the second tab index
End of Extract
Justification
To display different representations of graphs as some are more appropriate in different scenarios.
Tabs mechanism
Tabs
mechanism is implemented using TabsCommand.
It swaps tab panel between lineChart and barChart based on the index input by the user.
When the user types tab [INDEX]
, it creates raises new event
named JumpToTabRequestEvent
, which prompts the subscribed
method in GraphPanel
to change the tab page based on[INDEX].
The number of valid index is based on the number of tabs created, in this case it would be 2. A invalid index would be displayed if the index provided is out of bounds. |
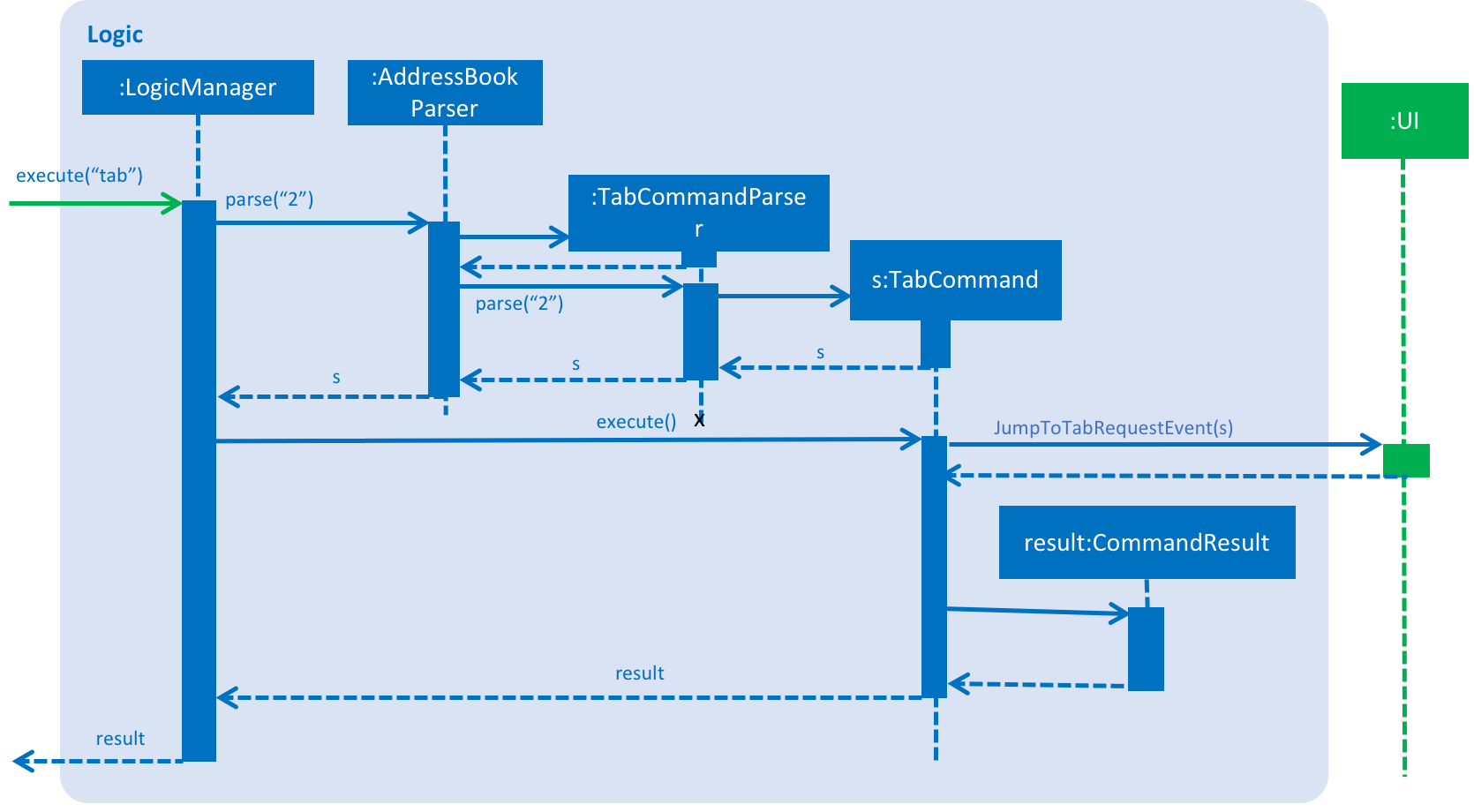
Figure 4.11.1 : Sequence Diagram of Tab
Operation
Design Considerations
Aspect: To display different chart types to help visual aid.
Alternative 1 (current choice): Do a TabPane.
Pros: More versatile and easier to further expand in the future.
Cons: More cumbersome to start.
Alternative 2: Multiple graphs on the same pane.
Pros: A easier comparison of the student grades between different graph types.
Cons: Difficult to further expand as there are limited space. Graph may be shrinked as well.
End of Extract
Enhancement Added: Home
External behavior
Start of Extract [from: User Guide]
Switching to home screen: home
Since v1.5
Switch all panels on the right to the home page.
The quotes on the home page is generated on random for you.
Using the select command
toggles the panel to Student Details Panel
, Statistics Panel
and Graph Panel
.
Format: home
To select your contacts on this page, use the select command . This is to ensure that people who access your cherBook are unable to view the information of your contacts easily.Do Not click on contacts while home page is being displayed! Use select command first and only when the panels do toggle are you able to click on the Students Panel.
|
Examples:
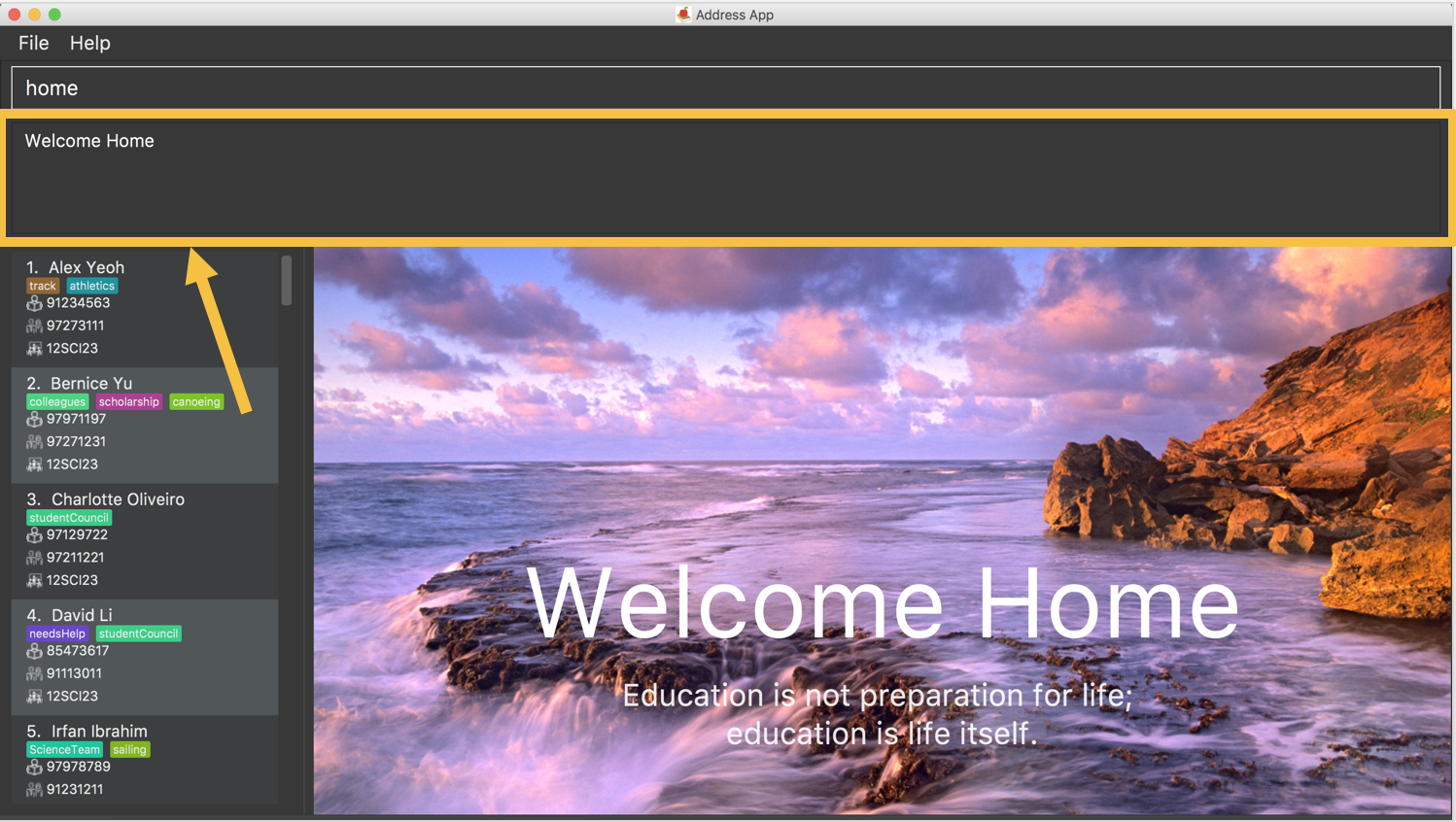
Figure 5.3.1.1 : Returns to the home page of cherBook
Home Panel Since v1.5
cherBook has a start up Home panel
which displays a picture to help you unwind even just a tiny bit.
It also displays random inspirational quotes to help you make yourself feel better.
What is better then starting off a day right feeling good.
These quotes changes every time the home page displayed through either calling then home
command or starting the application.
End of Extract
Justification
Added a home page to help lift one’s mood when using the application. In addition, it can act as a soft security measure when a teacher needs to leave the desk and in doing so prevents leak of information from passer bys.
Home Page mechanism
Home
mechanism is implemented using HomeCommand.
It displays the home page when the user enter the command and each time a randomly selected wallpaper and quote would be displayed.
When the home page is displayed, the extendedPersonCard, StatsPanel and GraphPanel would be switched out. Only when the user select a new person would the three panes swap back.
As we would be focusing on CLI implementations, we did not enable a way to select a person through clicking when the home page is displayed. |
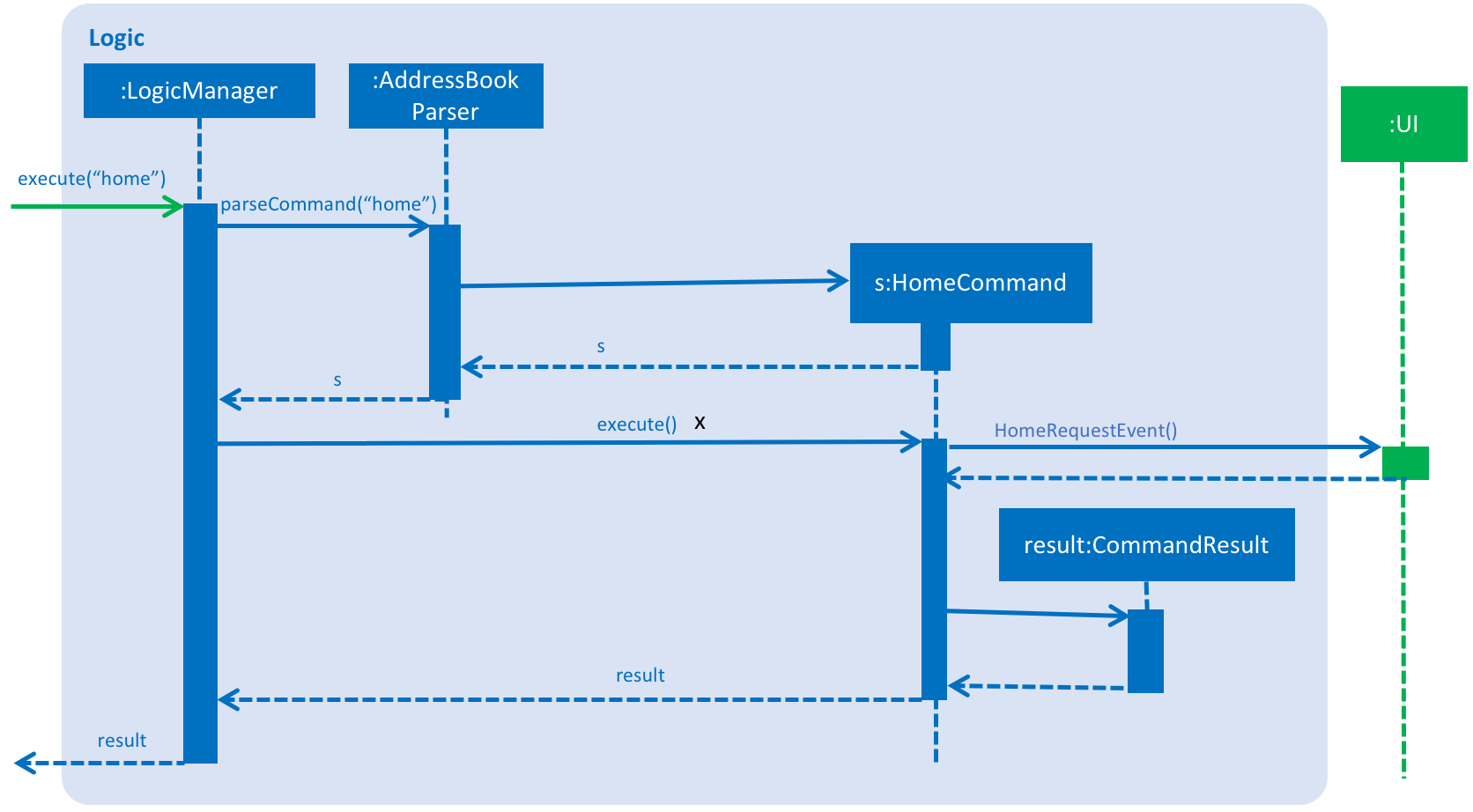
Figure 4.9.1 : Sequence Diagram of home
Operation
Design Considerations
Aspect: To have a soft security feature.
Alternative 1 (current choice): Disable easy access to data, only users who knows the command are able to view.
Pros: Easy to implement.
Cons: Easier to be break through
Alternative 2: Have a login page before displaying the main window.
Pros: The application as a whole would be more secure.
Cons: Takes a longer time to implement.
---
End of Extract
Enhancement Proposed: Add 25 & 75 percentile lines
External Behaviour
No external behaviour, when the statistics panel calculates the results, the line would be drawn at the different percentiles on the line graph.
Justification
Helps the teacher to easily show who are the students who are over and under performing and can easily use the graph for their reports.
Implementation
Create a line on the graph which would by sync to the figures provided by the statistics panel, such that every time the list changes, so does the line.
Enhancement Proposed: Refresh Home panel wallpaper
External Behaviour
Changes the wallpaper every time the home command is typed or when the application starts up.
Justification
Adds more variety to their wallpaper so that they do not have to feel bored looking at the same photo
Implementation
Read the filepath of the folder of wallpapers and creating a new array of files. Randomly pick a file and set it to Image every time the home command is entered.
Other contributions
-
Updated the GUI to include graphs of students either in class or tags (pull request #65, #87)
-
Wrote additional tests to increase coverage from 88% to 92% (Pull requests #36, #38)
-
Logic tabCommand implementation (Special thanks to Celine who helped me layout tabCommand) (https://github.com/CS2103AUG2017-T16-B2/main/pull/114)
-
Update Manual Testing Script (https://github.com/CS2103AUG2017-T16-B2/main/pull/146)
-
Rearranged panels in FXML such that Student Details Panel, Statistics Panel and Graph Panel are all on the same stack pane. (https://github.com/CS2103AUG2017-T16-B2/main/pull/139/files)
-
Fixed some bugs issues regarding help window (https://github.com/CS2103AUG2017-T16-B2/main/pull/157)
Project: Tea (Game)
A unity made 2D side scroller game that focuses on interacting with the surrounding environment to survive as long as possible. Some features include a combat system with wild animals, materials collected to create vase to collect water, wood chopped from trees to start fire etc. Some other features are event system where choices are given and there would be penalties (reduction of health, etc) or boons (receiving more food). Weapons and shelter can be crafted to aid in survival as well.